Hey, Python enthusiasts! Today we're going to talk about a super cool topic - Python's application in Internet of Things (IoT) development. Are you eager to learn how to use Python to make various devices "talk" to each other, creating awesome scenarios like smart homes and smart cities? Then join me in exploring this field full of opportunities and challenges!
Connectivity
In the world of IoT, connectivity is like the road system of a city. Without smooth connectivity, even the smartest devices can only admire themselves in isolation. So, how does Python help us build these "information highways"?
ESP8266
First up is the star of IoT development - ESP8266. This small but powerful WiFi module allows us to easily connect various devices to the network. But how do we use Python to establish a connection with a SocketIO server?
Here's a little trick: use the socket.io-client
library. It acts like a translator between ESP8266 and the SocketIO server, helping them "communicate" smoothly. However, before using it, don't forget to check if the WiFi connection is stable and if the server address and port are correct!
import socketio
sio = socketio.Client()
@sio.event
def connect():
print("Successfully connected to the server!")
@sio.event
def disconnect():
print("Disconnected from the server")
sio.connect('http://your_socketio_server:port')
See, it's that simple! Just a few lines of code can establish a stable connection between your ESP8266 and the server. Are you already imagining how cool it would be to control smart devices in your home this way?
SIM7080G
Next, let's turn our attention to a more challenging scenario - using the SIM7080G module to connect to AWS IoT Core. This task might intimidate some developers, but don't worry, Python always has a way to make complicated things simple!
The key is to correctly configure the AT commands for MQTT connection and ensure that the correct certificates and keys are used. It's like equipping your device with a unique key that only it can use to open the door to AWS IoT Core.
import serial
ser = serial.Serial('/dev/ttyUSB0', 115200, timeout=1)
def send_at(command, expected_response="OK", timeout=1):
ser.write((command + '\r
').encode())
response = ser.read(100).decode()
return expected_response in response
send_at('AT+CMQTTACCQ=0,"your_client_id"')
send_at('AT+CMQTTCONNECT=0,"ssl://your_aws_endpoint:8883",60,1')
send_at('AT+CMQTTTOPIC=0,18')
ser.write(b'your/mqtt/topic')
send_at('AT+CMQTTPAYLOAD=0,13')
ser.write(b'Hello, World!')
send_at('AT+CMQTTPUB=0,1,60')
print("Message successfully published!")
This code might look a bit complex, but it actually does something really cool: it allows your device to communicate securely with the cloud via cellular network. Imagine being able to remotely monitor and control devices distributed across various locations this way, isn't that exciting?
Data Management
After establishing the connection, the next challenge is how to manage and process massive amounts of data. In the world of IoT, data is like an endless river, and we need to use wisdom to navigate it.
Event-Driven Architecture
When dealing with IoT data, event-driven architecture is a very powerful pattern. It's like equipping your system with a pair of keen "eyes" and a flexible "brain" that can respond to various changes in real-time.
Python's advantages are fully demonstrated here. By using JSON format to serialize event messages, coupled with message brokers like Kafka or RabbitMQ, you can build an efficient and scalable data processing system.
import json
from kafka import KafkaProducer
producer = KafkaProducer(bootstrap_servers=['localhost:9092'])
def send_event(topic, event_data):
json_data = json.dumps(event_data).encode('utf-8')
producer.send(topic, json_data)
event = {
"device_id": "thermostat_01",
"event_type": "temperature_alert",
"temperature": 35.5,
"timestamp": "2024-10-16T14:30:00Z"
}
send_event('temperature_alerts', event)
print("Temperature alert sent!")
This code demonstrates how to use Kafka to send a temperature alert event. You can imagine that in a smart factory, such a system could monitor device status in real-time, promptly detect and handle abnormal situations, greatly improving production efficiency and safety.
Thingsboard Rule Chain
Speaking of data processing, we can't ignore the powerful IoT platform Thingsboard. Its rule chain function is like a visual "if-this-then-that" system, allowing you to easily design complex data processing logic.
For example, if you want to wait for a period of time after receiving sensor data before triggering an action, you can do this:
- Add a "delay" node in the rule chain.
- Set an appropriate delay time, such as 5 seconds.
- Connect the delay node to the subsequent processing node.
This way, you've created a simple timer function! Imagine using this method to control the fading effect of smart bulbs, or waiting for a period of time after detecting an anomaly before triggering an alarm to avoid false alarms. Python's flexibility is fully demonstrated here, allowing you to easily transform complex business logic into simple rule chains.
Security
When talking about IoT, we can't ignore the crucial topic of security. After all, no one wants their smart devices to become toys for hackers, right?
Secure Communication
Taking the Teltonika EYE sensor as an example, how can we securely send control instructions to it? This is where Python's bleak
library comes in handy, providing a secure and reliable way to communicate with Bluetooth devices.
import asyncio
from bleak import BleakClient
async def send_secure_code(address, code):
async with BleakClient(address) as client:
# Assume the characteristic UUID is "00001234-0000-1000-8000-00805f9b34fb"
characteristic_uuid = "00001234-0000-1000-8000-00805f9b34fb"
await client.write_gatt_char(characteristic_uuid, code.encode())
print(f"Security code {code} sent successfully!")
asyncio.run(send_secure_code("XX:XX:XX:XX:XX:XX", "1234"))
This code demonstrates how to use the bleak
library to send a security code to a Bluetooth device. It's like sending an encrypted command to your device, which only devices that know the "password" can correctly interpret and execute. This method is not only secure but also flexible, allowing you to easily apply it to various scenarios that require secure communication.
Challenges and Solutions
While IoT development is full of opportunities, it's not without challenges. Let's look at some common problems and their solutions.
Network Stability
In IoT projects, network connection stability is often a big challenge. Devices may be deployed in environments with unstable signals, so how do we ensure reliable data transmission?
Here's a little trick: implement an automatic reconnection mechanism. You can use Python's exception handling and loops to achieve this:
import time
import random
def connect_to_server():
# Simulate connection process
if random.random() < 0.3: # 30% chance of connection failure
raise ConnectionError("Connection failed")
print("Successfully connected to the server")
def send_data(data):
# Simulate data sending
if random.random() < 0.1: # 10% chance of sending failure
raise ConnectionError("Sending failed")
print(f"Data sent successfully: {data}")
max_retries = 5
retry_delay = 2
while True:
try:
connect_to_server()
for i in range(10): # Try to send data 10 times
send_data(f"Data packet {i}")
time.sleep(1)
except ConnectionError as e:
print(f"Error: {e}")
retries = 0
while retries < max_retries:
print(f"Attempting to reconnect ({retries + 1}/{max_retries})...")
time.sleep(retry_delay)
try:
connect_to_server()
break
except ConnectionError:
retries += 1
if retries == max_retries:
print("Maximum retry attempts reached, exiting program")
break
else:
print("Data sending complete, waiting for next round")
time.sleep(5)
This code simulates an unstable network environment and implements an automatic reconnection mechanism. It's like equipping your program with a "stubborn" personality that doesn't give up when faced with difficulties, but keeps trying until success. In actual IoT projects, such a mechanism can greatly improve the reliability of the system.
Resource Limitations
IoT devices are usually resource-limited. How can we implement complex functions with limited computing power and memory? This requires us to carefully design algorithms and optimize code structure.
For example, when dealing with large amounts of data, we can consider using generators to reduce memory usage:
def data_processor(data_source):
for item in data_source:
# Do some processing
yield process(item)
for processed_item in data_processor(large_data_source):
# Perform further operations
do_something(processed_item)
This method is like building an efficient "assembly line" that allows you to process an almost unlimited data stream with limited resources.
Looking to the Future
With the popularization of 5G technology and the development of edge computing, the application scenarios of IoT will become more diverse. Python, as a flexible, powerful, and easy-to-learn language, will undoubtedly play an increasingly important role in this field.
Imagine that in the near future, you might use Python to: - Control the traffic system of smart cities, achieving more efficient traffic management - Optimize agricultural production by improving crop yields through precise data analysis - Develop a new generation of wearable devices, providing more accurate data support for people's health management
Doesn't this sound exciting? But to realize these beautiful visions, we need to keep learning and innovating. For example, how can we better utilize artificial intelligence technology to process massive amounts of IoT data? How can we improve the interoperability of devices while ensuring security? These are all directions worth our in-depth research.
Do you have any thoughts on Python's application in the IoT field? Feel free to share your views and experiences in the comments section! Let's explore the endless possibilities of Python in the IoT world together!
![[xxx]]
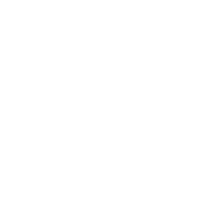
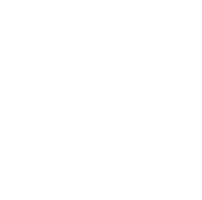