Introduction to Generators
Have you ever encountered a situation where you needed to process a large amount of data but struggled with insufficient memory? Or have you ever wondered why some Python functions can be iterated over without returning all results at once? If you're interested in these questions, then Python generators are definitely a tool you shouldn't miss!
Generators are a very elegant and powerful feature in Python. Simply put, a generator is a function that can "generate" values on demand. Unlike ordinary functions that return all results at once, generators can return results one by one during iteration. This feature makes generators particularly useful when dealing with large amounts of data, as they don't need to load all the data into memory at once.
How They Work
So, how do generators work? Let's understand through a simple example:
def count_up_to(n):
i = 1
while i <= n:
yield i
i += 1
for num in count_up_to(5):
print(num)
In this example, count_up_to
is a generator function. Notice that it uses the yield
keyword instead of return
. This small change turns the function into a generator.
When we call this function, it doesn't immediately execute the function body. Instead, it returns a generator object. Each time we iterate over this object (like in a for loop), the function executes up to the yield
statement, returns the current value, and then "pauses" there. On the next iteration, the function continues from where it paused.
This "lazy" execution makes generators particularly suitable for handling large datasets or infinite sequences. You can imagine, if we were to generate a list containing millions of numbers, a regular function would occupy a lot of memory at once, while a generator can generate numbers as needed, greatly saving memory usage.
Generator Expressions
Besides defining generators using functions, Python also provides a more concise way — generator expressions. Their syntax is similar to list comprehensions, but uses parentheses instead of square brackets. For example:
squares = (x**2 for x in range(10))
for square in squares:
print(square)
This generator expression will generate the squares of numbers from 0 to 9. Unlike list comprehensions, it doesn't calculate all results at once, but calculates the current value only when iterating.
Practical Applications
After talking about so much theory, you might ask: what's the practical use of generators? Let me give a few examples:
- File Reading: When dealing with large files, reading the entire file at once might exhaust memory. Using generators, we can read the file line by line:
def read_large_file(file_path):
with open(file_path, 'r') as file:
for line in file:
yield line.strip()
for line in read_large_file('huge_file.txt'):
process_line(line)
- Data Stream Processing: When processing real-time data streams, generators can continuously generate new data points:
import time
def sensor_data():
while True:
yield get_sensor_reading()
time.sleep(1)
for data in sensor_data():
analyze_data(data)
- Infinite Sequences: Generators can be used to represent infinite sequences, such as the Fibonacci sequence:
def fibonacci():
a, b = 0, 1
while True:
yield a
a, b = b, a + b
for i, num in enumerate(fibonacci()):
if i >= 10:
break
print(num)
Performance Considerations
Generators not only save memory but can also improve program execution speed in some cases. Because they generate data on demand, they can avoid unnecessary calculations and memory allocations when dealing with large amounts of data.
However, generators also have their limitations. Because they can only access data sequentially, generators might not be the best choice if you need random access or need to traverse the data multiple times. In these cases, you might need to weigh memory usage against access flexibility.
In-Depth Discussion
So far, we've learned about the basic concepts and usage of generators. But Python generators have some more advanced features that are worth exploring in depth:
1. send() Method
Generators can not only produce values but also receive values! This is achieved through the send()
method:
def echo_generator():
while True:
value = yield
print(f"Received: {value}")
gen = echo_generator()
next(gen) # Start the generator
gen.send("Hello") # Output: Received: Hello
gen.send("World") # Output: Received: World
In this example, the generator becomes a two-way channel, not only producing values but also receiving external input. This provides the basis for implementing complex coroutines.
2. throw() and close() Methods
Generators also provide throw()
and close()
methods, allowing us to throw exceptions to the generator or close the generator:
def number_generator():
try:
yield 1
yield 2
yield 3
except GeneratorExit:
print("Generator is closing")
except ValueError:
print("Caught ValueError")
yield "Error occurred"
gen = number_generator()
print(next(gen)) # Output: 1
print(gen.throw(ValueError)) # Output: Caught ValueError, then output: Error occurred
gen.close() # Output: Generator is closing
These methods allow us to more flexibly control the behavior of generators, handle exceptional situations, or release resources in a timely manner when they are no longer needed.
3. yield from
Python 3 introduced the yield from
syntax, which allows us to yield values from another iterable or generator:
def subgenerator():
yield 1
yield 2
yield 3
def main_generator():
yield "Start"
yield from subgenerator()
yield "End"
for item in main_generator():
print(item)
This feature not only simplifies code but also makes the composition and reuse of generators easier.
Practical Case
Let's consolidate what we've learned through a slightly more complex practical case. Suppose we're developing a log analysis tool that needs to process a large number of log files. Our task is to find all log lines containing "ERROR" and extract the error information.
import re
from typing import Generator
def read_logs(file_path: str) -> Generator[str, None, None]:
with open(file_path, 'r') as file:
for line in file:
yield line.strip()
def filter_errors(logs: Generator[str, None, None]) -> Generator[str, None, None]:
error_pattern = re.compile(r'ERROR: (.+)')
for log in logs:
match = error_pattern.search(log)
if match:
yield match.group(1)
def process_logs(file_path: str) -> Generator[str, None, None]:
logs = read_logs(file_path)
return filter_errors(logs)
for error in process_logs('application.log'):
print(f"Found error: {error}")
In this example, we used multiple generator functions:
read_logs
: Reads the log file line by line.filter_errors
: Filters out log lines containing "ERROR" and extracts the error information.process_logs
: Combines the above two generators to form a processing pipeline.
The advantages of this approach are:
- Memory efficiency: No matter how large the log file is, we only need to keep the currently processed line in memory.
- Flexible processing: We can easily add more steps to the processing pipeline, such as adding timestamp filtering, error classification, etc.
- Clear code: Each function has a clear single responsibility, making the code easy to understand and maintain.
Summary and Reflection
Through this article, we've explored in depth the concept, working principle, and practical applications of Python generators. Generators are a powerful and elegant feature in Python that not only help us save memory and improve performance but also make our code clearer and more expressive.
In my view, generators embody an important design philosophy of Python — "Simple is better than complex." Through a simple yield
keyword, Python provides us with an elegant way to handle large datasets and infinite sequences. This design not only makes the code more concise but also encourages us to think about problems in a more "Pythonic" way.
However, like all programming concepts, generators are not omnipotent. In some cases, such as when you need to traverse data multiple times or access data randomly, using lists or other data structures might be more appropriate. As programmers, we need to choose the most suitable tool based on specific situations.
So, have you used generators in your daily programming? Have you encountered scenarios where generators were particularly useful or not quite suitable? I'd love to hear about your experiences and thoughts.
Finally, I'd like to leave you with a thought question: If you were developing an application that needs to process real-time data streams (such as stock trading data), how would you use generators to design your program? Consider the various stages of data reception, processing, and output.
Remember, programming is not just about writing code, but more about a way of thinking. I hope this article has sparked your interest and thoughts on Python programming. Let's explore more mysteries in the ocean of Python together!
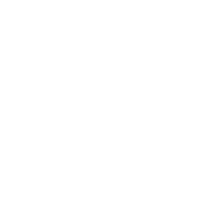
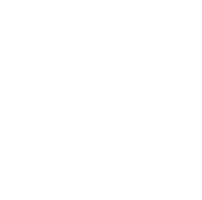
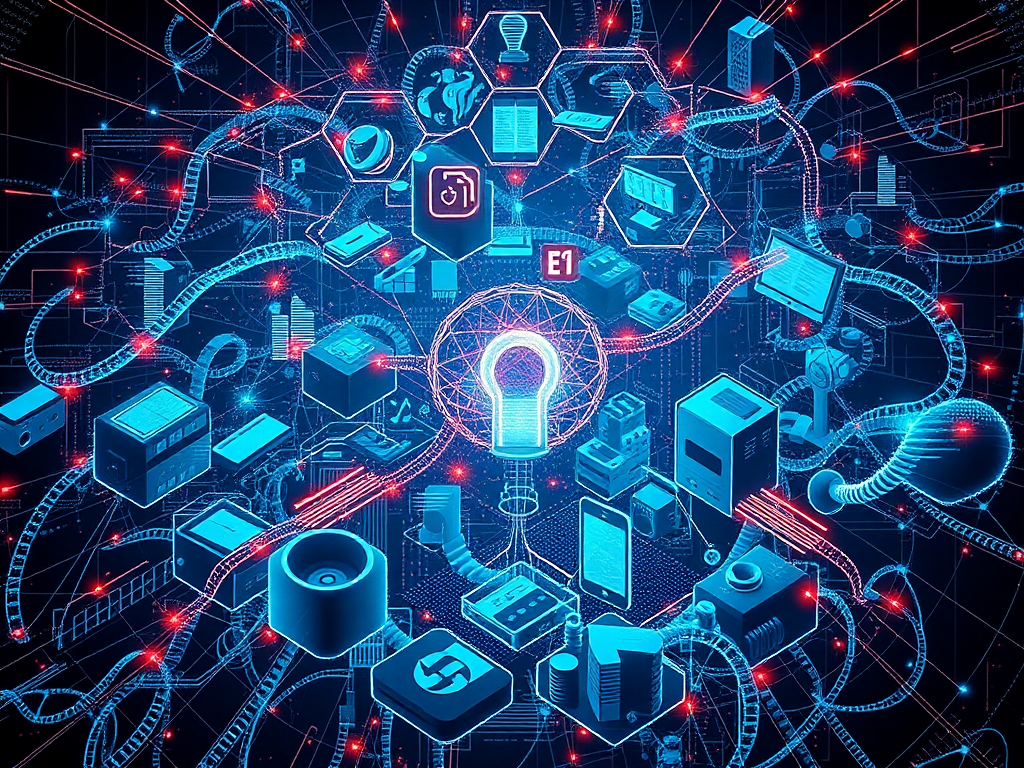
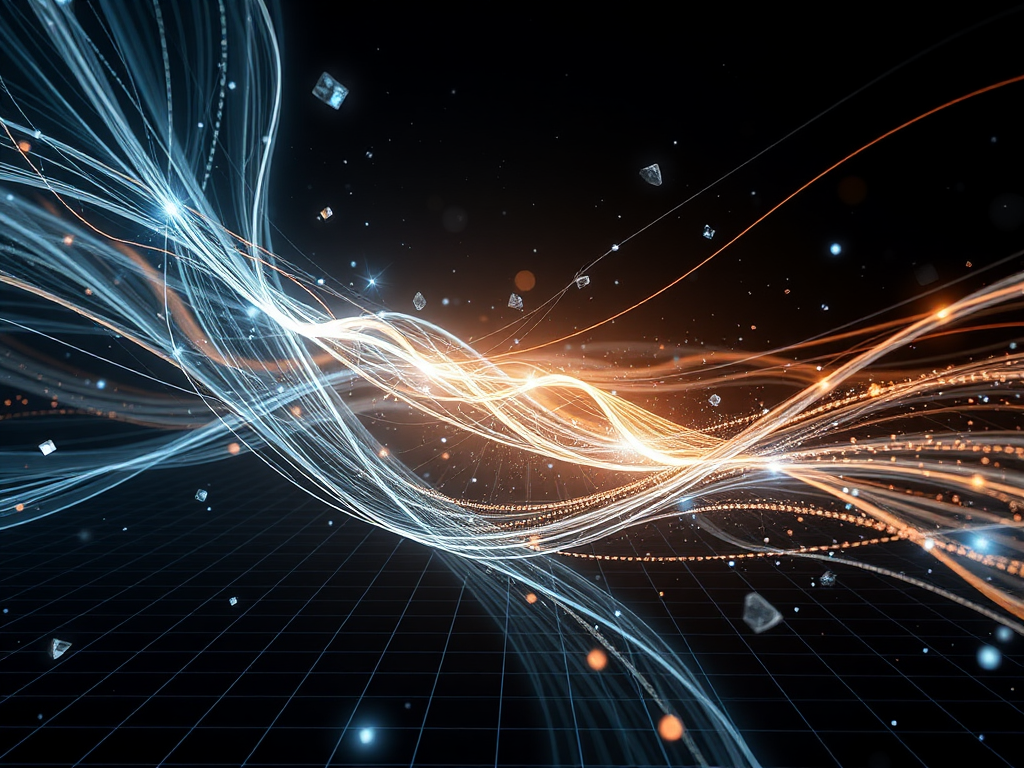
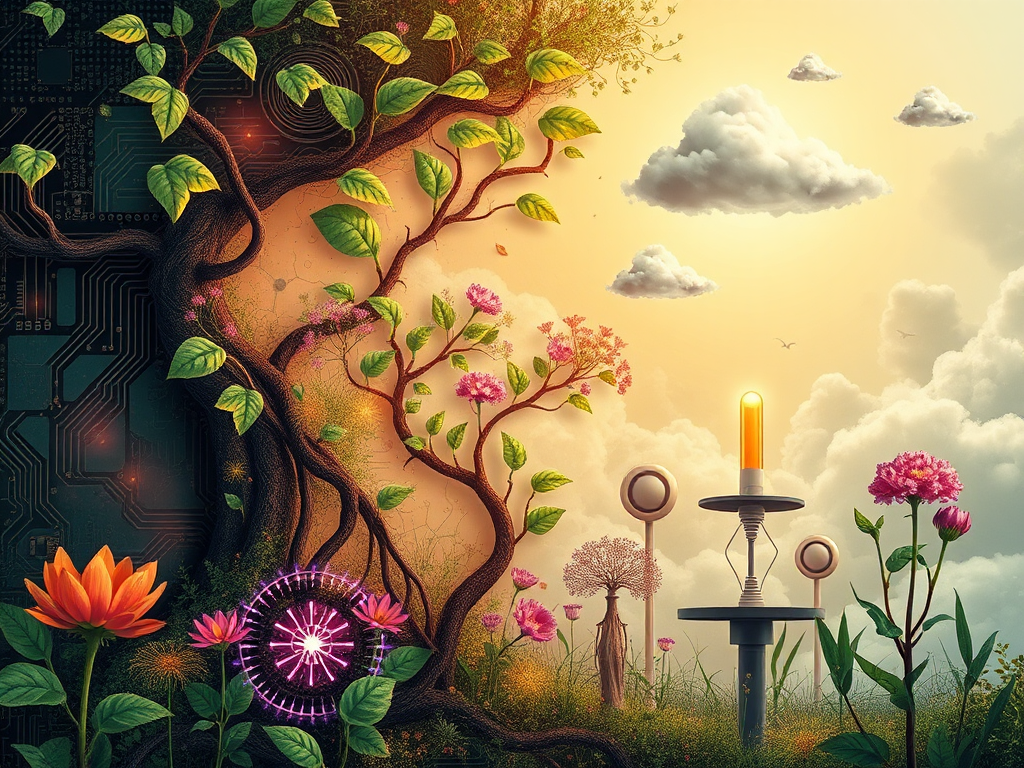