Hello, dear Python programming enthusiasts! Today, let's talk about the application of Python in IoT (Internet of Things) development. This topic is quite popular and practical! Let's explore how Python helps us connect and control various smart devices, achieving data collection, transmission, and analysis. Ready? Let's start this exciting IoT journey!
Introduction to the Internet of Things
First, let's briefly understand what the Internet of Things is. As the name suggests, it's a technology that connects various objects through networks. It makes our lives smarter and more convenient. Imagine being able to control your home's lights, air conditioning, or even the refrigerator remotely with your phone! That's the magic of IoT.
So, what role does Python play in this? With its simple syntax and rich library support, Python has become one of the main forces in IoT development. Whether it's collecting sensor data or controlling smart devices, Python can handle it. Next, let's look at specific applications of Python in IoT.
MQTT Protocol: The "Common Language" of IoT
In the IoT world, devices need a "common language" to communicate. This language is the MQTT protocol. MQTT (Message Queuing Telemetry Transport) is a lightweight message transmission protocol, especially suitable for resource-constrained devices and low-bandwidth, high-latency, or unreliable networks.
Python has a powerful library called Paho MQTT, which allows us to easily use the MQTT protocol. Let's look at a simple example:
import paho.mqtt.client as mqtt
def on_connect(client, userdata, flags, rc):
print("Connected with result code "+str(rc))
# Subscribe to topic
client.subscribe("home/temperature")
def on_message(client, userdata, msg):
print(f"Received message: {msg.topic} {str(msg.payload)}")
client = mqtt.Client()
client.on_connect = on_connect
client.on_message = on_message
client.connect("mqtt.example.com", 1883, 60)
client.loop_forever()
This code creates an MQTT client, connects to an MQTT broker, and subscribes to the "home/temperature" topic. Whenever new temperature data is published to this topic, our program will be notified. Isn't it amazing?
You might ask, what is the MQTT broker in this example? It's like a relay station responsible for receiving and distributing messages. In practical applications, you can use public MQTT brokers or set up your own.
Raspberry Pi: A Good Partner for Python
Speaking of IoT development, we must mention the Raspberry Pi. This credit card-sized computer, paired with Python, is simply the perfect combination for IoT development!
Let's look at an example of using Raspberry Pi and Python to read data from a temperature and humidity sensor:
import Adafruit_DHT
import time
sensor = Adafruit_DHT.DHT22
pin = 4
while True:
# Try to read temperature and humidity data
humidity, temperature = Adafruit_DHT.read_retry(sensor, pin)
if humidity is not None and temperature is not None:
print(f'Temperature: {temperature:.1f}°C, Humidity: {humidity:.1f}%')
else:
print('Failed to get reading. Try again!')
time.sleep(2) # Read every 2 seconds
This program uses a DHT22 sensor to read temperature and humidity data every two seconds. Isn't it simple? You can easily send this data to the cloud using MQTT or store it in a database for analysis.
Cloud IoT Platform: Making Devices Smarter
Now, we know how to read data from sensors and communicate using MQTT. But if we want to manage a large number of devices or achieve more complex functions, we need to use a cloud IoT platform.
Azure IoT Hub is a great choice. It offers powerful features like device management and data analysis. Let's see how to connect to Azure IoT Hub using Python:
from azure.iot.device import IoTHubDeviceClient, Message
import time
CONNECTION_STRING = "Your device connection string"
def send_telemetry():
try:
client = IoTHubDeviceClient.create_from_connection_string(CONNECTION_STRING)
print("Connecting to Azure IoT Hub...")
client.connect()
print("Connected!")
while True:
# Simulate temperature data
temperature = 20 + (random.random() * 15)
message = Message(f'{{"temperature": {temperature}}}')
print(f"Sending message: {message}")
client.send_message(message)
time.sleep(5) # Send data every 5 seconds
except KeyboardInterrupt:
print("Stopped by user")
finally:
client.disconnect()
print("Disconnected from Azure IoT Hub")
if __name__ == '__main__':
send_telemetry()
This program simulates a temperature sensor, sending temperature data to Azure IoT Hub every 5 seconds. You can view this data in real-time on the Azure portal and even set alert rules.
Deep Dive: Machine Learning and IoT
We've learned how to collect and transmit data, but the charm of IoT doesn't stop there. By combining machine learning, we can make devices even smarter. For example, we can use collected temperature and humidity data to predict future weather conditions.
Here's a simple example showing how to use the scikit-learn library to train a linear regression model:
from sklearn.linear_model import LinearRegression
import numpy as np
temperatures = np.array([20, 21, 22, 23, 24, 25, 26, 27]).reshape(-1, 1)
humidities = np.array([50, 52, 54, 56, 58, 60, 62, 64])
model = LinearRegression()
model.fit(temperatures, humidities)
future_temp = np.array([[28]])
predicted_humidity = model.predict(future_temp)
print(f"Predicted humidity when temperature is 28°C: {predicted_humidity[0]:.2f}%")
This example is simple, but it shows how to apply machine learning to IoT data analysis. You can imagine that with more data and more complex models, we can make more accurate predictions and intelligent decisions.
Security: The Top Priority of IoT
When it comes to IoT, we must mention a crucial topic: security. Imagine how terrifying it would be if hackers could control the smart devices in your home! Therefore, when developing IoT applications, we must always remember security.
Here are some basic security suggestions:
- Use encrypted communication: Always use HTTPS and MQTT over TLS.
- Implement authentication: Ensure that only authorized devices and users can access your system.
- Update promptly: Keep your software and firmware up to date to fix known security vulnerabilities.
- Principle of least privilege: Give devices and users the minimum necessary permissions.
Let's see an example of an MQTT connection using TLS encryption:
import paho.mqtt.client as mqtt
import ssl
def on_connect(client, userdata, flags, rc):
print(f"Connected with result code {rc}")
client.subscribe("secure/topic")
def on_message(client, userdata, msg):
print(f"{msg.topic} {str(msg.payload)}")
client = mqtt.Client()
client.on_connect = on_connect
client.on_message = on_message
client.tls_set(ca_certs="path/to/ca.crt", certfile="path/to/client.crt",
keyfile="path/to/client.key", cert_reqs=ssl.CERT_REQUIRED,
tls_version=ssl.PROTOCOL_TLS, ciphers=None)
client.connect("secure-broker.example.com", 8883, 60)
client.loop_forever()
This example shows how to use TLS encryption to protect an MQTT connection. Remember, security is an ongoing process, and we need to stay vigilant.
Conclusion: The Bright Future of Python and IoT
Our IoT journey has come to a temporary stop. We learned about the MQTT protocol, explored applications with Raspberry Pi, understood cloud IoT platforms, and even touched on the application of machine learning in IoT. But this is just the beginning!
There are many more applications of Python in IoT development. For example, we can use Django or Flask to create web interfaces to control and monitor devices, use Pandas and Matplotlib to analyze and visualize data, or use TensorFlow for more complex machine learning tasks.
IoT technology is rapidly developing, and Python, as a flexible, powerful, and easy-to-learn language, will undoubtedly play an increasingly important role in this field. I believe that as you delve deeper into Python and IoT technology, you will be able to develop amazing smart applications!
So, are you ready to start your IoT journey? Pick up your Raspberry Pi, open your Python IDE, and let's create a smart future together!
Remember, in IoT development, the most important thing is to maintain curiosity and an innovative spirit. Constantly try new technologies and solve practical problems, and you'll discover the infinite possibilities of combining Python and IoT.
Let's look forward to more miracles created by Python in the IoT world! Do you have any ideas or projects? Feel free to share in the comments section, and we'll discuss and learn together. Happy coding, and enjoy IoT!
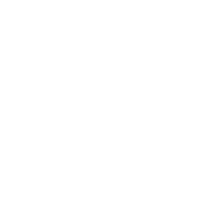
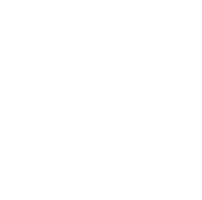