Hello, Python enthusiasts! Today, let's talk about the application of Python in IoT hardware development. This topic is both interesting and practical. As a Python programming blogger, I've always been passionate about the IoT field. Let's explore how Python shines in this exciting domain!
First Encounter with Raspberry Pi
Do you remember my excitement when I first got my Raspberry Pi? That tiny board holds such powerful functions. And Python is the key to unlocking this "magic box."
First, we need to set up the development environment. Ensure your Raspberry Pi has the Raspbian OS and Python 3.5 or higher. Don't worry; the process is simple. If you're uncertain, check the Raspberry Pi website for detailed installation guides.
Once set up, we can start exploring. Did you know? Controlling the Raspberry Pi's GPIO pins with Python is as fun as conducting a small symphony. We can connect various electronic components, like LEDs, buttons, and sensors.
Let me show you a simple example - making an LED blink:
import RPi.GPIO as GPIO
import time
GPIO.setmode(GPIO.BCM)
GPIO.setup(18, GPIO.OUT)
try:
while True:
GPIO.output(18, GPIO.HIGH) # LED on
time.sleep(1) # Wait 1 second
GPIO.output(18, GPIO.LOW) # LED off
time.sleep(1) # Wait another second
except KeyboardInterrupt:
GPIO.cleanup() # Clean up GPIO settings
See? With just a few lines of code, we can make the LED blink rhythmically. Isn't it amazing?
The World of Sensors
When talking about IoT, we can't ignore sensors. Temperature and humidity sensors, light sensors, motion sensors... these little guys are like our "electronic eyes" and "electronic noses," helping us perceive the world.
Python provides many libraries to simplify sensor interaction. For example, we can use the Adafruit_DHT
library to read data from a DHT11 temperature and humidity sensor:
import Adafruit_DHT
sensor = Adafruit_DHT.DHT11
pin = 4
humidity, temperature = Adafruit_DHT.read_retry(sensor, pin)
if humidity is not None and temperature is not None:
print(f'Temperature: {temperature:.1f}°C Humidity: {humidity:.1f}%')
else:
print('Failed to get reading. Try again!')
This code looks simple, right? But the principles behind it are not. Sensors convert environmental temperature and humidity into electrical signals through a series of complex physical and electronic processes, and our Python code then converts these signals into values we can understand. Isn't that the charm of IoT?
MQTT: The "WeChat" of IoT
When talking about IoT, we must mention the MQTT protocol. This lightweight messaging protocol is like the "WeChat" for IoT devices, allowing easy communication between devices.
Python's Paho MQTT library lets us easily implement MQTT communication on IoT devices. Let's see how to connect to the Alibaba Cloud IoT platform using it:
import paho.mqtt.client as mqtt
import time
productKey = "a1LhUsK***"
deviceName = "python***"
deviceSecret = "bdd043d193782d11***"
host = f"{productKey}.iot-as-mqtt.cn-shanghai.aliyuncs.com"
port = 1883
def on_connect(client, userdata, flags, rc):
if rc == 0:
print("Connected successfully!")
else:
print("Connection failed, error code:", rc)
client = mqtt.Client()
client.on_connect = on_connect
client.username_pw_set(username=deviceName, password=deviceSecret)
client.connect(host, port)
client.loop_start()
for i in range(5):
message = f"Message {i}"
client.publish(f"/{productKey}/{deviceName}/user/update", message)
print(f"Published message: {message}")
time.sleep(2)
client.loop_stop()
client.disconnect()
This code might seem a bit complex, but don't worry. Let me explain. First, we created an MQTT client and set up a connection callback function. Then, we connected to Alibaba Cloud's IoT platform and started publishing messages.
Just like that, our IoT device can communicate with the cloud platform. Isn't it fascinating? This is the charm of IoT - it makes our devices "smart," able to communicate with each other and even talk to the cloud.
Multithreading: Making IoT Devices More Powerful
In IoT development, we often need to handle multiple tasks simultaneously. For example, we might need to read data from multiple sensors at the same time or control multiple LEDs. This is where multithreading comes in handy.
Let's see how to use threads to control multiple LEDs simultaneously:
import RPi.GPIO as GPIO
import threading
import time
GPIO.setmode(GPIO.BCM)
led_pins = [18, 23]
for pin in led_pins:
GPIO.setup(pin, GPIO.OUT)
def blink_led(pin):
while True:
GPIO.output(pin, GPIO.HIGH)
time.sleep(1)
GPIO.output(pin, GPIO.LOW)
time.sleep(1)
threads = []
for pin in led_pins:
thread = threading.Thread(target=blink_led, args=(pin,))
threads.append(thread)
thread.start()
try:
while True:
time.sleep(0.1)
except KeyboardInterrupt:
GPIO.cleanup()
This code creates two threads, each controlling an LED. This way, we can control two LEDs to blink simultaneously, independently.
Multithreading might seem a bit complex, but it can significantly improve the performance and responsiveness of our IoT devices. Imagine if our device needs to process data from multiple sensors simultaneously while controlling multiple actuators. Multithreading becomes very useful.
Asynchronous Programming: Making IoT Devices More Efficient
To improve efficiency, we must mention asynchronous programming. In IoT development, we often need to handle many I/O operations, like reading sensor data or sending network requests. These operations often involve waiting, and if we use synchronous programming, our program does nothing while waiting.
With asynchronous programming, we can continue with other tasks while waiting for an operation to complete. Python's asyncio
library provides us with powerful asynchronous programming tools.
Here's an example of asynchronously controlling multiple LEDs and reading sensor data:
import asyncio
import RPi.GPIO as GPIO
import Adafruit_DHT
GPIO.setmode(GPIO.BCM)
led_pins = [18, 23]
sensor = Adafruit_DHT.DHT11
sensor_pin = 4
for pin in led_pins:
GPIO.setup(pin, GPIO.OUT)
async def blink_led(pin):
while True:
GPIO.output(pin, GPIO.HIGH)
await asyncio.sleep(1)
GPIO.output(pin, GPIO.LOW)
await asyncio.sleep(1)
async def read_sensor():
while True:
humidity, temperature = Adafruit_DHT.read_retry(sensor, sensor_pin)
if humidity is not None and temperature is not None:
print(f'Temperature: {temperature:.1f}°C Humidity: {humidity:.1f}%')
await asyncio.sleep(5)
async def main():
tasks = [blink_led(pin) for pin in led_pins] + [read_sensor()]
await asyncio.gather(*tasks)
try:
asyncio.run(main())
except KeyboardInterrupt:
GPIO.cleanup()
This code looks complex but accomplishes a powerful function: controlling two LEDs to blink while reading temperature and humidity data every 5 seconds. Moreover, these operations are asynchronous and do not affect each other.
Asynchronous programming might take some time to master, but it can make our IoT devices more efficient and responsive. Isn't that cool?
Event-Driven Programming: Making IoT Devices Smarter
In IoT development, we often need to respond to various events. For example, when a button is pressed, we need to perform specific actions; when a sensor detects abnormal data, we need to issue an alert. This is where event-driven programming comes into play.
Python's asyncio
library supports both asynchronous and event-driven programming. Let's look at an example:
import asyncio
import RPi.GPIO as GPIO
GPIO.setmode(GPIO.BCM)
button_pin = 17
led_pin = 18
GPIO.setup(button_pin, GPIO.IN, pull_up_down=GPIO.PUD_UP)
GPIO.setup(led_pin, GPIO.OUT)
async def button_pressed(pin):
while True:
GPIO.wait_for_edge(pin, GPIO.FALLING)
print("Button pressed!")
GPIO.output(led_pin, GPIO.HIGH)
await asyncio.sleep(1)
GPIO.output(led_pin, GPIO.LOW)
async def main():
task = asyncio.create_task(button_pressed(button_pin))
await task
try:
asyncio.run(main())
except KeyboardInterrupt:
GPIO.cleanup()
This code implements a simple function: the LED lights up for 1 second when a button is pressed. It looks simple, right? But it contains the core idea of event-driven programming: instead of constantly polling the button's state, our program waits for the "button pressed" event. This approach saves resources and improves response speed.
Event-driven programming allows our IoT devices to respond intelligently to various situations rather than executing the same operations repeatedly. Isn't that cool?
Data Analysis: Making IoT Devices "Smarter"
Collecting data is only the first step in IoT; more importantly, it's about analyzing the data to extract valuable information. Python has a powerful ecosystem for data analysis, such as NumPy, Pandas, and Matplotlib.
Let's look at an example of using Pandas and Matplotlib to analyze and visualize temperature and humidity data collected from sensors:
import pandas as pd
import matplotlib.pyplot as plt
from datetime import datetime, timedelta
import random
start_time = datetime.now()
times = [start_time + timedelta(hours=i) for i in range(24)]
temperatures = [random.uniform(20, 30) for _ in range(24)]
humidities = [random.uniform(40, 60) for _ in range(24)]
df = pd.DataFrame({
'time': times,
'temperature': temperatures,
'humidity': humidities
})
fig, ax1 = plt.subplots(figsize=(12,6))
ax1.set_xlabel('Time')
ax1.set_ylabel('Temperature (°C)', color='tab:red')
ax1.plot(df['time'], df['temperature'], color='tab:red')
ax1.tick_params(axis='y', labelcolor='tab:red')
ax2 = ax1.twinx() # Create a second y-axis sharing the x-axis
ax2.set_ylabel('Humidity (%)', color='tab:blue')
ax2.plot(df['time'], df['humidity'], color='tab:blue')
ax2.tick_params(axis='y', labelcolor='tab:blue')
fig.tight_layout() # Automatically adjust subplot parameters to fill the entire image area
plt.title('Temperature and Humidity over 24 Hours')
plt.show()
This code simulates 24-hour temperature and humidity data, processes it with Pandas, and then uses Matplotlib to create a beautiful chart. With this chart, we can visually see the trends in temperature and humidity changes.
Data analysis allows our IoT devices to not only collect data but also understand it. Imagine if our smart home system could analyze past temperature and humidity data and automatically adjust the air conditioner and humidifier settings. How cool would that be?
Machine Learning: The Future of IoT
Speaking of making IoT devices "smarter," we can't ignore machine learning. Python dominates the machine learning field with rich libraries and tools like scikit-learn, TensorFlow, and PyTorch.
Let's look at a simple example of using scikit-learn to predict future temperatures:
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
import numpy as np
days = np.arange(1, 31).reshape(-1, 1)
temperatures = np.random.uniform(20, 30, 30)
X_train, X_test, y_train, y_test = train_test_split(days, temperatures, test_size=0.2, random_state=42)
model = LinearRegression()
model.fit(X_train, y_train)
future_days = np.arange(31, 38).reshape(-1, 1)
predicted_temperatures = model.predict(future_days)
print("Predicted temperatures for the next 7 days:")
for day, temp in zip(future_days, predicted_temperatures):
print(f"Day {day[0]}: {temp:.2f}°C")
This code uses a linear regression model to predict temperatures for the next 7 days. Although this is a straightforward model, it demonstrates the potential of machine learning in IoT.
Imagine if our IoT devices could learn user behavior patterns and predict user needs. How would that change our lives? For example, a smart home system could predict when you come home and turn on the air conditioner in advance; a smart agriculture system could predict future weather and automatically adjust irrigation plans. This is the charm of combining IoT and machine learning.
Security: A Top Priority in IoT
When talking about IoT, we can't ignore security. As more devices connect to the network, security becomes increasingly important. Python provides many tools to help us enhance the security of IoT devices.
For example, we can use Python's cryptography
library to encrypt data:
from cryptography.fernet import Fernet
key = Fernet.generate_key()
f = Fernet(key)
message = "This is a secret message"
encrypted_message = f.encrypt(message.encode())
print("Encrypted message:", encrypted_message)
decrypted_message = f.decrypt(encrypted_message)
print("Decrypted message:", decrypted_message.decode())
This code shows how to use symmetric encryption to protect data. In real IoT applications, we can use this method to protect sensor data, user information, and other sensitive data.
Besides encryption, Python can help us implement other security measures, such as authentication and access control. Remember, in IoT development, security is always the top priority!
Cloud Computing: The Strong Backbone of IoT
Finally, let's talk about cloud computing. Cloud computing provides strong backend support for IoT, allowing our IoT devices to store and process large amounts of data and perform complex computing tasks.
Python offers many libraries to help us interact with cloud services. For example, we can use the boto3
library to interact with Amazon Web Services (AWS):
import boto3
s3 = boto3.client('s3')
s3.upload_file('local_file.txt', 'my_bucket', 'remote_file.txt')
s3.download_file('my_bucket', 'remote_file.txt', 'downloaded_file.txt')
response = s3.list_objects_v2(Bucket='my_bucket')
for obj in response['Contents']:
print(obj['Key'])
This code demonstrates how to use Python to upload files to AWS S3, download files, and list all objects in a bucket. In real IoT applications, we can use this method to store and manage large amounts of sensor data.
Cloud computing not only provides storage services but also powerful computing capabilities. We can perform complex data processing and machine learning tasks in the cloud, significantly reducing the burden on IoT devices.
Conclusion: The Bright Future of Python and IoT
Well, our Python IoT journey ends here. We started with basic GPIO control and explored complex machine learning and cloud computing applications. Did you feel the powerful charm of Python in the IoT field?
Python's simple syntax, rich libraries, and robust ecosystem make it an ideal choice for IoT development. Whether you want to control a small LED or build a complex smart home system, Python can meet your needs.
Remember, the world of IoT is vast, and we've only scratched the surface today. Many interesting topics await exploration, such as edge computing and blockchain applications in IoT. I hope this article inspires your interest in IoT and encourages you to further explore this exciting field.
Finally, I'd like to say that technology is advancing rapidly, and we must maintain a passion for learning and constantly update our knowledge. The combination of Python and IoT has a long way to go, and the future is full of possibilities. Let's look forward, work together, and create a smarter, better future!
What are your thoughts on Python's application in IoT? Do you have any interesting IoT projects to share? Feel free to leave a comment, and let's discuss and learn together!
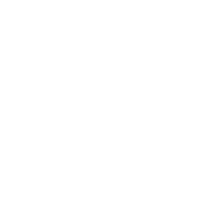
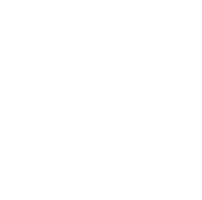