Have you ever wondered how a small Python script can enable your smart devices to communicate seamlessly with cloud servers? Today, let's delve into the fascinating applications of Python in IoT device connectivity. Whether you're a newcomer just getting started with IoT or a seasoned veteran who has been in this field for years, I believe this article will bring you some new insights and food for thought.
Getting Started
Let's begin with the most basic connection between ESP8266 and a SocketIO server. This might be the first challenge many people face when they start IoT development.
WiFi Configuration
I remember when I first started working with ESP8266, I always got stuck at the WiFi connection step. Have you encountered the same problem? Actually, the solution is quite simple:
import network
def connect_wifi(ssid, password):
sta_if = network.WLAN(network.STA_IF)
if not sta_if.isconnected():
print('Connecting to WiFi...')
sta_if.active(True)
sta_if.connect(ssid, password)
while not sta_if.isconnected():
pass
print('WiFi connected successfully, network config:', sta_if.ifconfig())
connect_wifi('Your WiFi Name', 'Your WiFi Password')
This code looks simple, but it solves our first challenge. You see, through the network
module, we can easily control the network connection of ESP8266. The key here is to make sure your WiFi name and password are correct.
SocketIO Connection
Next, let's look at how to connect to a SocketIO server:
from socketio import Client
sio = Client()
@sio.event
def connect():
print('Connected to SocketIO server')
@sio.event
def disconnect():
print('Disconnected from SocketIO server')
sio.connect('http://your-server-address:port')
This code doesn't look complicated either, right? But if your connection status keeps showing DISCONNECTED, don't panic! Let's check some possible reasons:
- Make sure your WiFi connection is normal. You can try pinging your server address first.
- Carefully check if the address and port of the SocketIO server are correct. Sometimes, a small typo can cause connection failure.
- Make sure the SocketIO library you're using is compatible with ESP8266. Different versions of the library may have compatibility issues.
I remember once I spent an entire afternoon debugging, only to find out that the problem was a missing letter in the server address. So, checking every detail carefully is really important!
Diving Deeper
After covering the basics of ESP8266, let's look at a more complex scenario - MQTTS connection between SIM7080G module and AWS IoT Core. This task sounds quite sophisticated, doesn't it? But don't worry, we'll take it step by step.
Firmware Support
First, we need to ensure that the firmware of the SIM7080G module supports MQTTS. This is crucial because if the firmware doesn't support it, all subsequent efforts will be in vain. You can check the firmware version with the following AT command:
AT+CGMR
If the version number is lower than a specific value (you'll need to check the SIM7080G documentation for the exact value), then you might need to upgrade the firmware first.
SSL Certificate Configuration
Next is the SSL certificate configuration. This step might give many people a headache, but it's actually not that complicated:
import serial
def configure_ssl(ser):
ser.write(b'AT+CSSLCFG="sslversion",0,4\r
')
ser.write(b'AT+CSSLCFG="authmode",0,2\r
')
ser.write(b'AT+CSSLCFG="cacert",0,"cacert.pem"\r
')
ser.write(b'AT+CSSLCFG="clientcert",0,"client.crt"\r
')
ser.write(b'AT+CSSLCFG="clientkey",0,"client.key"\r
')
ser = serial.Serial('/dev/ttyUSB0', 115200, timeout=1)
configure_ssl(ser)
This code sets the SSL version, authentication mode, and configures the necessary certificates. Remember, all certificate files need to be uploaded to the SIM7080G module in advance.
AT Command Connection
Finally, we use AT commands to establish the MQTTS connection:
def connect_mqtt(ser):
ser.write(b'AT+CMQTTSTART\r
')
ser.write(b'AT+CMQTTACCQ=0,"clientId","username","password"\r
')
ser.write(b'AT+CMQTTCONNECT=0,"tcp://your-aws-endpoint:8883",60,1\r
')
connect_mqtt(ser)
This code starts the MQTT client, sets the client ID, username and password, and then connects to AWS IoT Core.
If you encounter problems during this process, don't get discouraged! Debugging is an integral part of IoT development. You can get more error information by checking the serial port output:
def debug_output(ser):
while True:
if ser.in_waiting:
print(ser.readline().decode('utf-8').strip())
debug_output(ser)
This function will continuously output the content of the serial port, helping you locate the problem.
Management
After the connection is established, we enter the stage of data management and processing. Here, I'd like to share some experiences I've accumulated in actual projects.
Event-Driven Architecture
In IoT development, event-driven architecture is a very important concept. We often need to handle large amounts of nested event data. My suggestions for this are:
-
Use JSON format to serialize event messages. JSON is not only easy to read but also very convenient when dealing with nested data.
-
Consider using MongoDB to store these nested schemas. MongoDB's document-type storage is very suitable for the characteristics of IoT data.
-
Use RabbitMQ to handle event streams. RabbitMQ can help us better manage a large number of concurrent events.
Here's a simple example showing how to use Python to process JSON format event data and store it in MongoDB:
import json
from pymongo import MongoClient
client = MongoClient('mongodb://localhost:27017/')
db = client['iot_database']
collection = db['events']
def process_event(event_json):
event_data = json.loads(event_json)
collection.insert_one(event_data)
event_json = '{"device_id": "123", "temperature": 25.5, "humidity": 60}'
process_event(event_json)
This code parses JSON format event data and stores it in MongoDB. What do you think of this approach? Does it meet your needs?
ThingsBoard Platform
Speaking of IoT platforms, ThingsBoard is a very powerful choice. I've used it in multiple projects, and here are some practical tips.
- Create a timer/delay in the rule chain:
var delay = 5000; // 5 seconds delay
return {
delay: delay
}
This code can be used in the JavaScript node of the rule chain, it will create a 5-second delay. This is very useful when you need to process or delay the execution of certain operations.
- Automatically assign devices to the owner of the gateway:
var msg = {};
msg.ownerId = metadata.prevOwner;
return {
msg: msg,
metadata: metadata,
msgType: "POST_ATTRIBUTES_REQUEST"
};
This code can be used in the rule chain, it will automatically assign newly created devices to the owner of the gateway. This can greatly simplify the workflow when managing a large number of devices.
Have you encountered similar needs when using ThingsBoard? Are these solutions helpful to you?
Reflection
Looking back at the entire process of IoT device connection, from the most basic WiFi connection, to complex MQTTS communication, to data management and platform applications, we can see how important a role Python has played. Python's concise syntax and rich libraries make IoT development easier and more interesting.
However, we also need to recognize that IoT development is not all smooth sailing. Network connection instability, hardware compatibility issues, data security challenges, these are all real problems we need to face. As developers, we need to keep learning and keep trying to stay competitive in this rapidly developing field.
What challenges have you encountered in IoT development? And how did you overcome them? I'd love to hear about your experiences and thoughts.
Finally, I want to say that the world of IoT is so vast and full of possibilities. Every connected device, every piece of transmitted data, could bring an opportunity to change the world. As Python developers, we are fortunate to be able to participate in this exciting revolution. Let's continue to explore, continue to innovate, and use code to build a smarter, more connected future!
What are your expectations for the future of IoT? And what role will Python play in it? Let's discuss, think, and grow together.
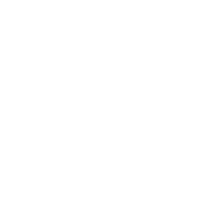
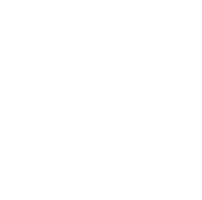