Hello, Python enthusiasts! Today we're going to talk about a very interesting topic - the application of Python in Internet of Things (IoT) development. As a programmer who loves Python, I've found that Python really shines in the IoT field, especially when dealing with various communication protocols. Let's dive in and see how Python makes the dialogue between devices so smooth!
The Magical MQTT
First, let's talk about the MQTT (Message Queuing Telemetry Transport) protocol. This name sounds complicated, but it's actually a lightweight message transmission protocol designed specifically for IoT. Imagine what it would be like if all your smart home devices were chatting using MQTT?
The working principle of MQTT is actually very simple: it's based on a publish/subscribe model. You can think of it as a super-efficient postal system. Devices can send messages to specific "topics" (like mailing letters) and can also subscribe to topics they're interested in (like subscribing to magazines). This way, devices can efficiently exchange information.
So, how does Python interact with MQTT? It's simple, we have a powerful library called paho-mqtt. Let's see how to use it to send a message:
import paho.mqtt.client as mqtt
client = mqtt.Client()
client.connect("broker.hivemq.com", 1883)
client.publish("home/livingroom/temperature", "25")
client.disconnect()
Isn't it simple? With just a few lines of code, you can make your Python program send messages to devices around the world!
But is MQTT really suitable for all scenarios? This brings us to the difference between MQTT and another popular system, Apache Kafka.
MQTT is like a lightweight postman designed specifically for IoT devices. It's fast, efficient, and particularly suitable for working in environments with poor network conditions. Kafka, on the other hand, is more like a large logistics center. It can handle massive data streams and is suitable for scenarios that require complex data processing and analysis.
Personally, I think MQTT is sufficient for most IoT projects. It's simple, efficient, and Python supports it very well. But if your project involves large-scale data analysis, you might need to consider using Kafka.
MQTT's Little Secret
Speaking of MQTT, do you know what its message length limit is? This question is actually quite interesting. Theoretically, the maximum message length supported by the MQTT protocol is 256MB. However, in practical use, this limit is often much smaller.
Why? Mainly because IoT devices usually have limited resources and may have difficulty processing large amounts of data. Also, network conditions will affect the actual message size that can be transmitted. So, in practical applications, we usually control the message size to between a few KB and tens of KB.
Here's a little trick: if you need to transmit a large amount of data, you can consider splitting the data and sending it through multiple messages. Python's list slicing feature comes in handy here:
data = "This is a very long message..."
chunk_size = 1000 # Size of each chunk
for i in range(0, len(data), chunk_size):
chunk = data[i:i+chunk_size]
client.publish("long_message", chunk)
This way, we can easily send even very long messages through MQTT. Isn't that great?
The Magic of WiFi Connection
After talking about MQTT, let's discuss WiFi connections. In IoT development, we often need to connect devices to specific WiFi networks. Here's an interesting question: how do you connect to a WiFi network without internet access?
This issue is particularly common in Android development. For example, you might need to have your Android application connect to a hotspot created by a smart device. This is where we need to use Android's WifiManager class.
Although this is not a pure Python solution, it's still helpful for those who develop Android applications using Kivy or other Python frameworks. The basic idea is:
- Get the WifiManager instance
- Create a WifiConfiguration object
- Set the network SSID and password
- Use the addNetwork() method to add the network
- Use the enableNetwork() method to enable the network
The specific code might look like this:
from jnius import autoclass
WifiManager = autoclass('android.net.wifi.WifiManager')
WifiConfiguration = autoclass('android.net.wifi.WifiConfiguration')
wifi_manager = WifiManager.getSystemService(Context.WIFI_SERVICE)
config = WifiConfiguration()
config.SSID = '"YourSSID"' # Note the double quotes
config.preSharedKey = '"YourPassword"' # Note the double quotes
network_id = wifi_manager.addNetwork(config)
wifi_manager.enableNetwork(network_id, True)
This code uses the pyjnius library to call Android's Java API. Although it looks a bit complex, once you understand the principle, you'll find that it's actually a very powerful feature.
The Challenge of Video Streaming
When it comes to IoT communication, we can't avoid talking about a more challenging topic: video streaming. You might ask, can we transmit video streams through IoT protocols?
The answer is: yes, but there are many factors to consider.
First, we need to understand that IoT protocols like MQTT are mainly designed for transmitting small messages. However, if we use these protocols properly, we can achieve video streaming.
The key lies in how to process and compress video data. We can consider using low bit-rate video encoding, such as H.264 or VP8, and then split the video frames into small chunks for transmission. Python's opencv-python library can be very useful here:
import cv2
import paho.mqtt.client as mqtt
cap = cv2.VideoCapture(0)
client = mqtt.Client()
client.connect("broker.example.com", 1883)
while True:
ret, frame = cap.read()
if not ret:
break
# Compress image
_, buffer = cv2.imencode('.jpg', frame, [cv2.IMWRITE_JPEG_QUALITY, 50])
# Send image data
client.publish("video/stream", buffer.tobytes())
cap.release()
client.disconnect()
This example shows how to capture video frames, compress them, and then send them via MQTT. Of course, in practical applications, we also need to consider issues such as frame rate control and error handling.
Personally, I think that while it's feasible to transmit video streams through IoT protocols, it's more suitable for low-bandwidth, low-frame-rate application scenarios. For scenarios that require high-quality video transmission, traditional streaming protocols might be more appropriate.
The Mystery of Bluetooth Communication
Finally, let's talk about Bluetooth communication. In the IoT field, Bluetooth is a very important communication technology, especially for some short-range, low-power application scenarios.
Python also has powerful capabilities when dealing with Bluetooth communication. Although we may need to rely on APIs from languages like C++ for some low-level operations, Python provides many convenient libraries to simplify this process.
For example, we can use the PyBluez library for Bluetooth device discovery and connection:
import bluetooth
nearby_devices = bluetooth.discover_devices(lookup_names=True)
print("Found {} devices.".format(len(nearby_devices)))
for addr, name in nearby_devices:
print(" {} - {}".format(addr, name))
sock = bluetooth.BluetoothSocket(bluetooth.RFCOMM)
sock.connect(("00:11:22:33:44:55", 1))
sock.send("Hello from Python!")
sock.close()
This code demonstrates how to discover nearby Bluetooth devices and establish a connection with a specific device. Don't you feel that Python makes Bluetooth communication so simple?
Of course, in actual IoT projects, Bluetooth communication may involve more complex operations, such as handling pairing, encryption, etc. But Python's powerful ecosystem provides us with various tools to meet these challenges.
Conclusion
Alright, we've talked a lot today about Python's applications in IoT communication. From MQTT to WiFi, from video streaming to Bluetooth, we've seen Python demonstrate its powerful capabilities in various fields.
Have you noticed that Python not only makes these complex communications simple, but also provides us with great flexibility? This is why I love using Python in IoT projects so much.
Of course, technology in the IoT field is developing very fast, and what we discussed today may soon change. But I believe that as long as we maintain our enthusiasm for learning, we can definitely keep up with the pace of technology.
So, what interesting challenges have you encountered when using Python in IoT projects? Feel free to share your experiences and thoughts in the comments section. Let's explore the infinite possibilities of Python in the IoT world together!
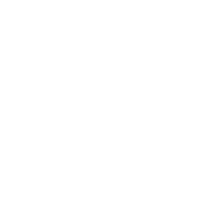
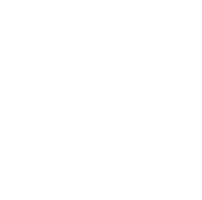