Hello Python enthusiasts! Today we'll discuss a very exciting topic - how to run Python code in the cloud. Imagine your code no longer being limited to your local computer, but running on powerful cloud servers instead. Cool, right? Let's explore this new world full of possibilities!
Why Choose Cloud
Before diving into technical details, let's talk about why we should move Python code to the cloud.
First, cloud computing provides us with almost unlimited computing resources. Remember when you ran that big data analysis task locally, with your computer's fan spinning wildly and CPU temperature soaring? In the cloud, you can easily access hundreds or thousands of times more computing power than locally, making complex machine learning model training effortless.
Second, cloud platforms provide rich services and tools that can greatly simplify our development process. For example, setting up a highly available web application might take a long time locally, but in the cloud it might only need a few commands.
Third, cloud collaboration is also a highlight. Your team members can access and modify code anytime, anywhere, without worrying about version confusion or file transfer issues.
Finally, don't forget cost-effectiveness. While cloud services might seem expensive at first glance, when you factor in hardware purchase, maintenance, electricity costs and other hidden expenses, cloud computing might actually be more economical, especially for projects with fluctuating computing needs.
Well, after hearing about all these benefits, are you eager to try it out? Next, let's look at specific methods for running Python code on several major cloud platforms.
Google's Offerings
When it comes to cloud computing, how can we not mention Google Cloud Platform (GCP)? As an industry giant, GCP offers Python developers multiple options for running code. Let's look at some of the most commonly used ones:
Data Processing Powerhouse
First up is Google Cloud Dataproc. This is a service optimized for big data processing and machine learning, particularly suitable for running distributed Python applications like PySpark.
The general process of using Dataproc is as follows:
- Create cluster: Like building a virtual supercomputer.
- Submit job: Send your Python code to run on this "supercomputer".
- Get results: Wait for computation to complete, then harvest the results.
- Delete cluster: Leave after use, saving money and being environmentally friendly.
Let's look at a specific example:
from google.cloud import dataproc_v1 as dataproc
cluster_client = dataproc.ClusterControllerClient(client_options={"api_endpoint": "us-central1-dataproc.googleapis.com"})
cluster = {
"project_id": "my-awesome-project",
"cluster_name": "my-dataproc-cluster",
"config": {
"master_config": {"num_instances": 1, "machine_type_uri": "n1-standard-2"},
"worker_config": {"num_instances": 2, "machine_type_uri": "n1-standard-2"}
}
}
operation = cluster_client.create_cluster(
request={"project_id": "my-awesome-project", "region": "us-central1", "cluster": cluster}
)
result = operation.result()
job_client = dataproc.JobControllerClient(client_options={"api_endpoint": "us-central1-dataproc.googleapis.com"})
job = {
"placement": {"cluster_name": "my-dataproc-cluster"},
"pyspark_job": {"main_python_file_uri": "gs://my-bucket/my_pyspark_script.py"}
}
operation = job_client.submit_job_as_operation(
request={"project_id": "my-awesome-project", "region": "us-central1", "job": job}
)
response = operation.result()
operation = cluster_client.delete_cluster(
request={"project_id": "my-awesome-project", "region": "us-central1", "cluster_name": "my-dataproc-cluster"}
)
operation.result()
Looks complicated? Don't worry, this code is actually doing three things: creating a Dataproc cluster with 1 master node and 2 worker nodes, then submitting a PySpark job, and finally deleting the cluster after the job is complete.
The benefits of using Dataproc are obvious - you can easily process terabytes or even petabytes of data, and only pay for the resources you actually use. For Python projects that need to regularly process large amounts of data, this is truly a godsend!
New Serverless Option
If your Python application doesn't need to run continuously but rather executes tasks occasionally when triggered, then Google Cloud Functions might be more suitable for you.
The steps to use Cloud Functions are very simple:
- Create function: Define your Python function.
- Deploy function: Upload the function to Google Cloud.
- Set trigger: Decide when to execute this function.
Let's look at an example:
def hello_world(request):
"""
This is a simple Cloud Function example
:param request: Flask request object
:return: Response text
"""
name = request.args.get('name', 'World')
return f'Hello, {name}!'
This function does something simple - it accepts an optional name parameter and returns a greeting. After deployment, you can trigger this function via HTTP request, like:
https://us-central1-my-project.cloudfunctions.net/hello_world?name=Python
This will return "Hello, Python!".
The beauty of Cloud Functions lies in its simplicity and flexibility. You don't need to manage any servers, just focus on your code logic. Plus, it can seamlessly integrate with other Google Cloud services, like triggering function execution when files are updated in Cloud Storage.
For Python developers who need to quickly build microservices or APIs, Cloud Functions is definitely worth considering.
Full Control with VMs
If you need more control, or if your Python application requires special environment configuration, then Google Compute Engine (GCE) might be a better choice. GCE is essentially a virtual machine service where you can install any software and libraries you need.
The general steps for using GCE are:
- Create instance: Choose operating system, configuration, etc.
- Connect to instance: Usually via SSH.
- Set up environment: Install Python and required libraries.
- Run code: Just like on your local machine.
Let's look at a specific example:
gcloud compute instances create my-python-instance \
--image-family=debian-10 \
--image-project=debian-cloud \
--machine-type=e2-medium \
--zone=us-central1-a
gcloud compute ssh my-python-instance --zone=us-central1-a
sudo apt-get update
sudo apt-get install -y python3 python3-pip
pip3 install numpy pandas matplotlib
cat << EOF > hello.py
import numpy as np
print("Hello from GCE!")
print("Here's a random number:", np.random.rand())
EOF
python3 hello.py
This example shows how to create a GCE instance, install Python and some common data science libraries, and then run a simple Python script.
The advantage of using GCE is that you have complete control. Need a specific version of Python? No problem. Need to install some unusual dependencies? Go ahead. This flexibility makes GCE a top choice for many Python developers, especially when you need to simulate specific production environments.
Amazon's Solutions
After discussing Google, let's look at another cloud computing giant - Amazon Web Services (AWS). AWS also provides rich options for Python developers, with AWS Lambda being perhaps the most popular.
Pioneer of Serverless Computing
AWS Lambda is one of the pioneers of serverless computing, allowing you to run code without managing servers. The steps to run Python code on Lambda are:
- Create function: Define your Python function in the AWS console.
- Write code: Write directly in the console editor or upload a zip package.
- Configure trigger: Decide when to execute this function.
- Test and deploy: Check if the function works properly, then deploy.
Let's look at a specific example:
import json
def lambda_handler(event, context):
"""
This is a simple AWS Lambda function example
:param event: Trigger event
:param context: Runtime information
:return: Response
"""
name = event.get('name', 'World')
return {
'statusCode': 200,
'body': json.dumps(f'Hello, {name}!')
}
This Lambda function is similar to the Google Cloud Function we saw earlier - it accepts an optional name parameter and returns a greeting. The difference is that Lambda functions need to return a dictionary containing statusCode and body.
The power of Lambda lies in its event-driven nature. You can integrate Lambda functions with various AWS services, like triggering functions when new files are uploaded to an S3 bucket, or creating serverless REST APIs through API Gateway.
For developers who want to quickly build and deploy Python applications without managing complex infrastructure, Lambda is an excellent choice. Moreover, Lambda's pricing model charges based on actual execution time, which might be the most economical choice for applications that don't need to run frequently but require quick response times.
Cloud Development Environments
After talking so much about how to run Python code in the cloud, you might ask: what about developing Python code in the cloud? Don't worry, we have solutions for that too!
The Charm of Online IDEs
First, let's look at Google Colab. Colab is an online IDE based on Jupyter Notebook, particularly suitable for data science and machine learning projects. The benefits of using Colab include:
- Free GPU and TPU resources
- Pre-installed with many common data science libraries
- Easy to share and collaborate
Let's look at a Colab example:
!pip install numpy pandas matplotlib
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
data = np.random.randn(1000)
plt.hist(data, bins=30)
plt.title('Distribution of Random Data')
plt.show()
This example shows how to install libraries, generate data, and create visualizations in Colab. One of Colab's major advantages is its interactivity - you can see results while writing code, which is perfect for exploratory data analysis!
Cloud Version of Jupyter
Besides Colab, many cloud platforms also provide Jupyter Notebook-based services. For example, AWS's SageMaker and GCP's AI Platform Notebooks. These services usually provide more powerful computing resources and more customization options.
The general steps for using cloud Jupyter are:
- Create notebook instance: Choose computing resources, storage, etc.
- Connect to notebook: Access through browser.
- Write and run code: Just like local Jupyter.
Let's look at an example of using Jupyter in AWS SageMaker:
import boto3
s3 = boto3.client('s3')
response = s3.list_buckets()
for bucket in response['Buckets']:
print(f'S3 bucket: {bucket["Name"]}')
from sagemaker import get_execution_role
from sagemaker.amazon.amazon_estimator import get_image_uri
role = get_execution_role()
container = get_image_uri(boto3.Session().region_name, 'xgboost')
xgb = sagemaker.estimator.Estimator(container,
role,
train_instance_count=1,
train_instance_type='ml.m4.xlarge',
output_path='s3://<your-bucket>/output',
sagemaker_session=sagemaker.Session())
xgb.set_hyperparameters(max_depth=5,
eta=0.2,
gamma=4,
min_child_weight=6,
subsample=0.8,
objective='binary:logistic',
num_round=100)
xgb.fit({'train': 's3://<your-bucket>/train.csv'})
This example shows how to use AWS services and SageMaker's built-in algorithms in a SageMaker notebook. The advantage of using cloud Jupyter is that you can easily access powerful computing resources and cloud services while maintaining the convenience of Jupyter's interactive development.
Summary and Outlook
Well, we've explored various ways to run and develop Python code in the cloud. From Google Cloud Platform's Dataproc, Cloud Functions, and Compute Engine, to AWS's Lambda, to various cloud Jupyter solutions, each method has its unique advantages and applicable scenarios.
Which method to choose depends on your specific needs:
- Need to process big data? Consider Dataproc.
- Want to quickly deploy microservices? Cloud Functions or Lambda might be good choices.
- Need complete control over the environment? Compute Engine is a good choice.
- Mainly doing data analysis or machine learning? Try Colab or SageMaker.
Regardless of which method you choose, cloud computing has brought unprecedented possibilities to Python development. You can easily access powerful computing resources, quickly deploy applications, and conveniently collaborate with teams.
However, remember that cloud computing also brings new challenges. Security, cost control, service selection and management all require us to learn and adapt. So while embracing cloud computing, also pay attention to continuously learning and improving your cloud computing skills.
How do you think cloud computing will change the future of Python development? Feel free to share your thoughts in the comments! If you're already using cloud computing for Python development, please also share your experiences and insights. Let's explore the infinite possibilities of Python together in this new era of cloud computing!
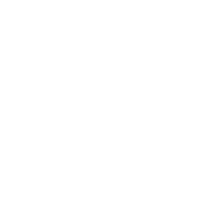
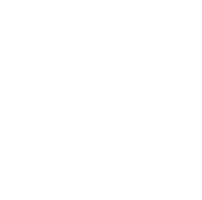