Hey, Python enthusiasts! Today, let’s dive into a cool and practical topic—how to run your Python code in the cloud. Do you often face issues like insufficient local computer performance for big data analysis? Or worry about code security and reliability? Don’t fret; cloud computing platforms are here to save the day! Let’s explore how to let your Python code soar in the cloud.
Taking Off in the Cloud
First, we need to understand why run Python code in the cloud. Imagine you have a super complex machine learning model to train, but your laptop runs like a snail. This is where the powerful computing capabilities of the cloud come into play. Moreover, cloud platforms offer high availability, elastic scalability, security, and other benefits.
I remember once handling a large dataset, and my local computer just quit. Switching to the cloud made it lightning-fast—what a difference! Since then, I’ve fallen in love with cloud computing.
Google Cloud Platform: Your Cloud Buddy
When it comes to cloud computing, Google Cloud Platform (GCP) is a must-mention. As an industry giant, GCP offers a range of powerful tools to support Python development. Let’s look at some of its star products.
Dataproc: A Big Data Tool
Have you heard of Apache Spark or Hadoop? These big data processing frameworks are favorites among data scientists. Google Cloud Dataproc is a managed Spark and Hadoop service, allowing you to easily run large-scale data processing jobs in the cloud.
Using Python to operate Dataproc is simple. First, you need to install the Google Cloud client library:
pip install google-cloud-dataproc
Then, you can create and manage Dataproc clusters with Python code. For example, creating a cluster:
from google.cloud import dataproc_v1
client = dataproc_v1.ClusterControllerClient()
cluster = {
"project_id": "your-project-id",
"cluster_name": "my-python-cluster",
"config": {
"master_config": {"num_instances": 1, "machine_type_uri": "n1-standard-2"},
"worker_config": {"num_instances": 2, "machine_type_uri": "n1-standard-2"},
},
}
operation = client.create_cluster(
request={"project_id": "your-project-id", "region": "us-central1", "cluster": cluster}
)
result = operation.result()
See, in just a few lines of code, you’ve created a Spark cluster in the cloud! Isn’t it amazing?
Cloud Functions: Serverless Magic
Next, let’s talk about Google Cloud Functions. This is an exciting serverless computing service. Imagine running Python code without managing any servers—isn’t that cool?
Creating a Cloud Function is super easy. You just need to create a new function in the Google Cloud Console, choose Python as the runtime, and start coding.
For example, we can create a simple HTTP trigger function:
def hello_world(request):
return 'Hello, World!'
Just like that, your function is deployed! Whenever an HTTP request arrives, this function gets triggered.
I once used Cloud Functions to make an email auto-reply tool. Whenever an email with a specific subject arrived, it automatically generated a polite reply. It saved me a lot of time and made me look super efficient!
AWS Lambda: Amazon’s Serverless Solution
After Google’s products, let’s look at another cloud giant—Amazon’s AWS. They also have a similar serverless computing service called AWS Lambda.
Running Python code with Lambda is straightforward. You can create a new Lambda function in the AWS Management Console, select Python as the runtime, and start coding.
For example, suppose we want to create a function to handle S3 upload events:
import json
def lambda_handler(event, context):
# Get the bucket name and object key from the event
bucket = event['Records'][0]['s3']['bucket']['name']
key = event['Records'][0]['s3']['object']['key']
print(f"File {key} was uploaded to bucket {bucket}")
return {
'statusCode': 200,
'body': json.dumps(f'Successfully processed {key}')
}
This function gets triggered when a new file is uploaded to an S3 bucket, printing the file information. Isn’t it convenient?
I once used Lambda to create an automatic image processing service. Whenever a user uploaded an image to S3, the Lambda function automatically cropped, compressed, and processed it. This greatly improved my application’s response speed, and the user experience became much better.
Online Python Development Environments: Code Anywhere
Besides these professional cloud computing platforms, there are lighter online Python development environments that let you code anytime, anywhere.
Google Colab: A Data Science Helper
Google Colab is one of my favorite online Python environments. It not only provides all the features of Jupyter Notebook but also offers free GPU resources! This is a boon for machine learning projects.
Using Colab is very simple; you just need a Google account. Log in, create a new Notebook, and start writing and running Python code.
For example, you can easily install and use various libraries:
!pip install pandas numpy matplotlib
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
data = pd.DataFrame(np.random.randn(100, 2), columns=['A', 'B'])
plt.scatter(data['A'], data['B'])
plt.title('Sample Scatter Plot')
plt.xlabel('A')
plt.ylabel('B')
plt.show()
This code installs the necessary libraries, generates random data, and plots a scatter plot. In Colab, you can directly see the graphical output—very convenient!
Jupyter Notebook: Local Cloud Feel
If you prefer working locally, Jupyter Notebook is a great choice. It provides an interactive programming environment similar to Colab but runs on your own computer.
Installing Jupyter is simple:
pip install jupyter
Then, enter jupyter notebook
in the command line to open the Jupyter interface in your browser.
You can even deploy Jupyter to a cloud server, accessing your Notebook from anywhere. I often do this, especially when handling long-running tasks, to check progress anytime—it’s very convenient.
Conclusion: Cloud Python, Endless Possibilities
Today we talked a lot about running Python in the cloud. From Google Cloud Platform to AWS, from Colab to Jupyter Notebook, these tools provide us with powerful cloud computing capabilities and flexible development environments.
Have you ever thought about what we can do with these tools? Maybe a real-time data analysis system, a large-scale machine learning model, or a high-concurrency web application. The possibilities are endless!
I’m curious, have you used these cloud computing tools? Any interesting challenges or insights? Feel free to share your experiences and thoughts in the comments. Let’s explore the endless possibilities of Python in the cloud together!
Remember, in the world of programming, the sky is the limit. And with cloud computing, we can even surpass the sky, reaching anywhere we want to go. So, are you ready? Let’s soar in the cloud together!
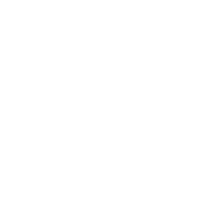
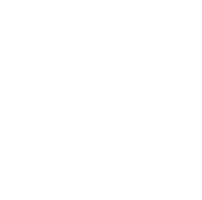