Hey, Python enthusiasts! Today let's talk about a topic that's both magical and practical - Python's application in cloud computing. Have you also often marveled at how powerful Python is, seemingly everywhere? Indeed, in the hot field of cloud computing, Python really shines. Let's unveil the mysterious face of Python in the cloud and see how it makes cloud computing simpler, more efficient, and more fun!
Walking in the Cloud
First, let's imagine: you're standing on a soft, fluffy cloud, holding a Python wand. Sounds dreamy, right? This is actually the theme we're going to explore today - how Python interacts with cloud services.
In this world of cloud computing, Python is like a universal translator, easily "conversing" with various cloud services. For example, if you want to create a data processing cluster on Google Cloud, Python can help you handle it easily.
Here's an example:
from google.cloud import dataproc_v1
client = dataproc_v1.ClusterControllerClient()
cluster = {
"project_id": "your-project-id",
"cluster_name": "my-super-cluster",
"config": {
"master_config": {"num_instances": 1},
"worker_config": {"num_instances": 2}
}
}
operation = client.create_cluster(
request={"project_id": cluster["project_id"], "region": "us-central1", "cluster": cluster}
)
result = operation.result()
print(f"Cluster {result.cluster_name} created successfully!")
See that? In just a few lines of code, you've created a powerful data processing cluster. Don't you feel like you've instantly become a cloud computing master?
Serverless Magic
Next, let's move to another magical realm of cloud computing - serverless computing. Here, Python is like a fish in water. Imagine your code appearing like magic when needed and disappearing when done - isn't that cool?
Google Cloud Functions is such a platform that lets your Python code stand by, ready at a moment's notice. For example, if you want a function to process images uploaded to cloud storage, you can write it like this:
from google.cloud import storage
from PIL import Image
import io
def process_image(data, context):
"""Automatically process images when new ones are uploaded"""
file_data = data
file_name = file_data["name"]
bucket_name = file_data["bucket"]
storage_client = storage.Client()
bucket = storage_client.get_bucket(bucket_name)
blob = bucket.get_blob(file_name)
# Download image
image_data = blob.download_as_bytes()
img = Image.open(io.BytesIO(image_data))
# Process image (resizing as an example)
img_resized = img.resize((300, 300))
# Save processed image
output_bucket = storage_client.get_bucket("processed-images")
output_blob = output_bucket.blob(f"processed_{file_name}")
img_byte_arr = io.BytesIO()
img_resized.save(img_byte_arr, format=img.format)
img_byte_arr = img_byte_arr.getvalue()
output_blob.upload_from_string(img_byte_arr, content_type=f"image/{img.format.lower()}")
print(f"Image {file_name} processed and saved!")
Look, once this function is deployed to Cloud Functions, it automatically monitors your specified bucket. Whenever a new image is uploaded, it springs into action, processes the image to 300x300 size, and saves it to another bucket. This is the charm of serverless computing - you only need to focus on code logic, leaving everything else to the cloud platform.
Art Gallery in the Cloud
Speaking of cloud computing, you might think it's only for serious business applications. But let me tell you a little secret: cloud computing can be fun too! For instance, we can draw with Python on a virtual desktop in the cloud. Sounds magical, doesn't it?
Alibaba Cloud's Cloud Computer provides such a platform. Imagine sitting at home, opening a browser window, and entering a fully functional cloud computer. Here, you can run various Python programs, including graphics drawing.
Look at this cute example where we use Python's Turtle library to draw a little tiger in the cloud:
from turtle import *
import time
speed(1000) # Set drawing speed
def set_start(x, y, w, c='#B2814D'):
penup()
setx(x)
sety(y)
setheading(towards(0, 0))
width(w)
pencolor(c)
pendown()
def draw_head():
set_start(0, -40, 2.5)
circle(100, 360)
def draw_ears():
set_start(-70, 80, 2)
setheading(160)
circle(30, 230)
set_start(70, 80, 2)
setheading(20)
circle(-30, 230)
def draw_eyes():
set_start(-40, 50, 2, 'black')
dot(20)
set_start(40, 50, 2, 'black')
dot(20)
def draw_nose():
set_start(0, 20, 2, 'black')
dot(15)
def draw_mouth():
set_start(-20, 0, 2, 'black')
setheading(-60)
circle(25, 120)
draw_head()
draw_ears()
draw_eyes()
draw_nose()
draw_mouth()
hideturtle() # Hide the pen
done() # Complete the drawing
See, in just a few lines of code, an adorable little tiger appears on screen. This isn't just a fun programming exercise, but a wonderful way to combine art and technology. Imagine holding a Python drawing competition in the cloud, where participants can showcase their talents and create various amazing patterns with code. This not only sparks creativity but also helps people better understand Python's graphics processing capabilities.
Infinite Possibilities in the Cloud
At this point, have you gained a new understanding of Python's applications in cloud computing? From processing big data and deploying serverless functions to drawing in the cloud, Python seems capable of anything. But this is just the beginning - the world of cloud computing has infinite possibilities waiting for us to explore.
For example, you could use Python to develop a cloud-based AI assistant to help students solve programming problems; or create a distributed computing system using the cloud's powerful computing capabilities to solve complex scientific problems; or even build a cloud gaming platform that allows players to enjoy high-quality gaming experiences on any device.
Python's application in cloud computing is like giving us wings, allowing our imagination to soar freely. Every line of code has the potential to bring world-changing power, and cloud computing provides an infinite stage for this power.
Embarking on the Cloud Journey
So, are you ready to start your Python cloud computing journey? Don't worry, everyone starts from zero. Remember, the most important thing in learning programming is maintaining curiosity and an exploratory spirit. Why not start with small projects, like trying to deploy a simple web application on cloud functions, or using Python to analyze data stored in the cloud?
During the learning process, you might encounter various challenges. Maybe it's difficult-to-understand error messages or complex API documentation. But don't be discouraged - these are opportunities for growth. Remember to communicate with other developers, participate in open-source projects, or ask questions on Stack Overflow. The cloud computing community is very active, and there's always someone willing to help.
Finally, I want to say that the combination of Python and cloud computing isn't just a fusion of technologies, but a perfect combination of innovation and practice. It gives us unlimited possibilities to build smarter, more efficient, and more interesting applications. So, let's embrace this era of cloud computing full of opportunities and use Python to weave our cloud dreams!
What thoughts or experiences do you have about Python's applications in cloud computing? Feel free to share your story in the comments. Let's discuss, learn together, and create miracles in the cloud together!
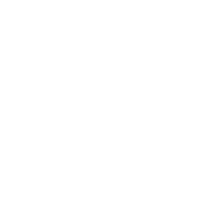
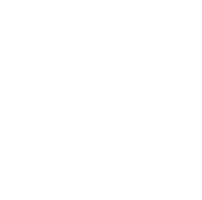
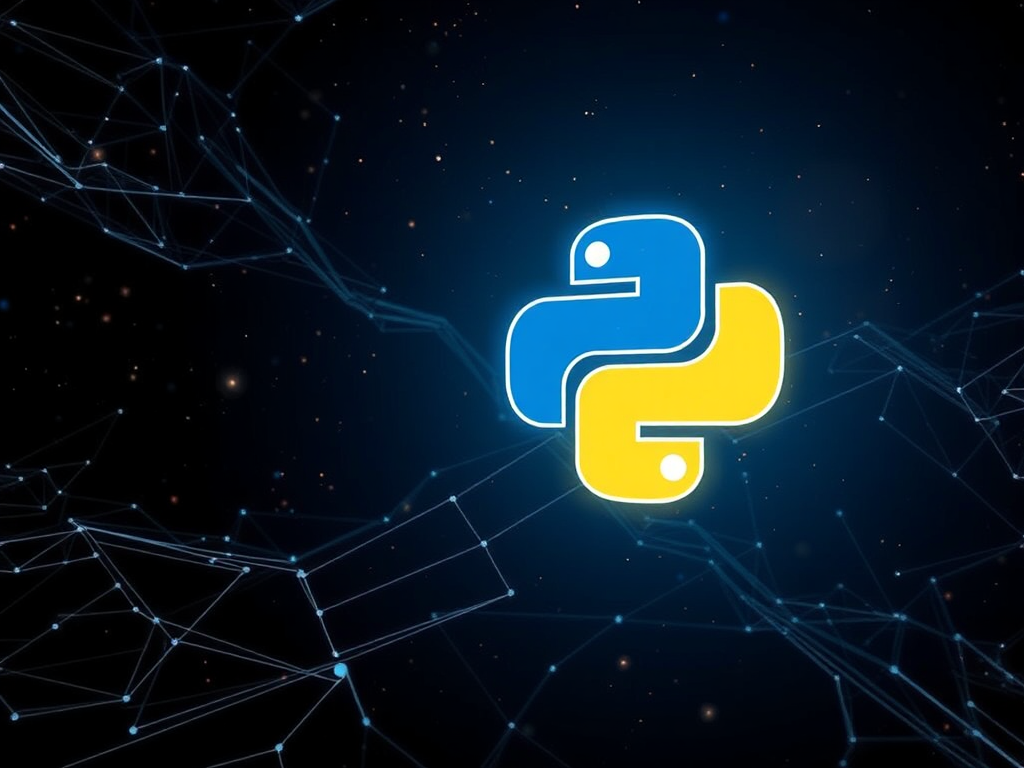
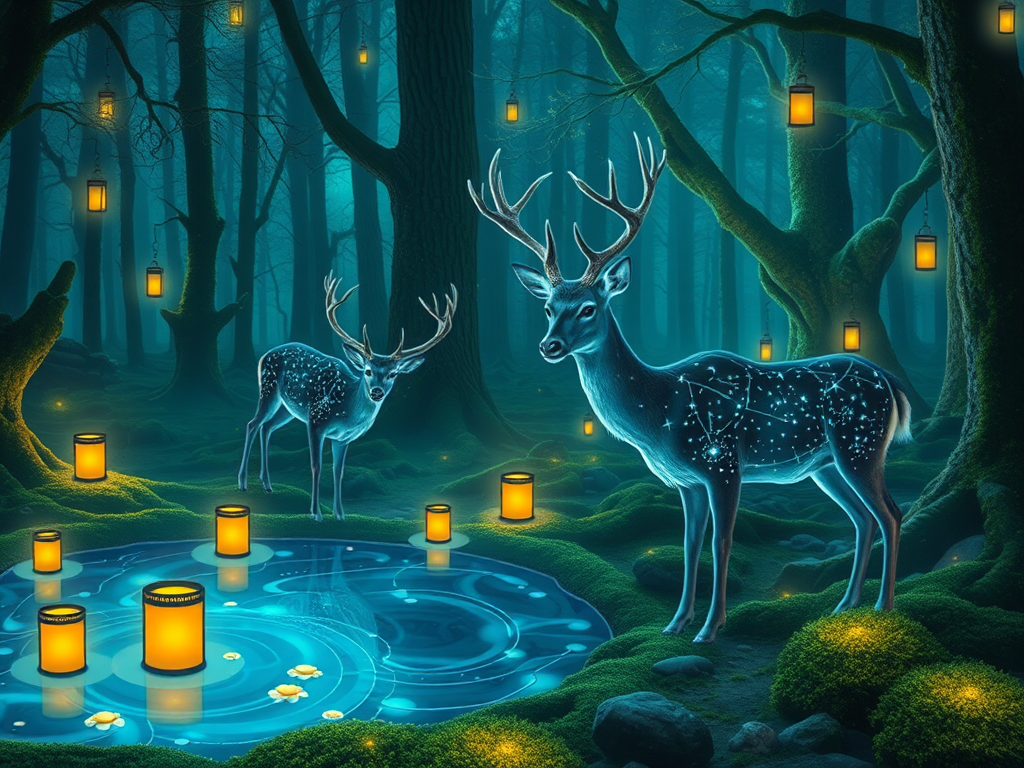
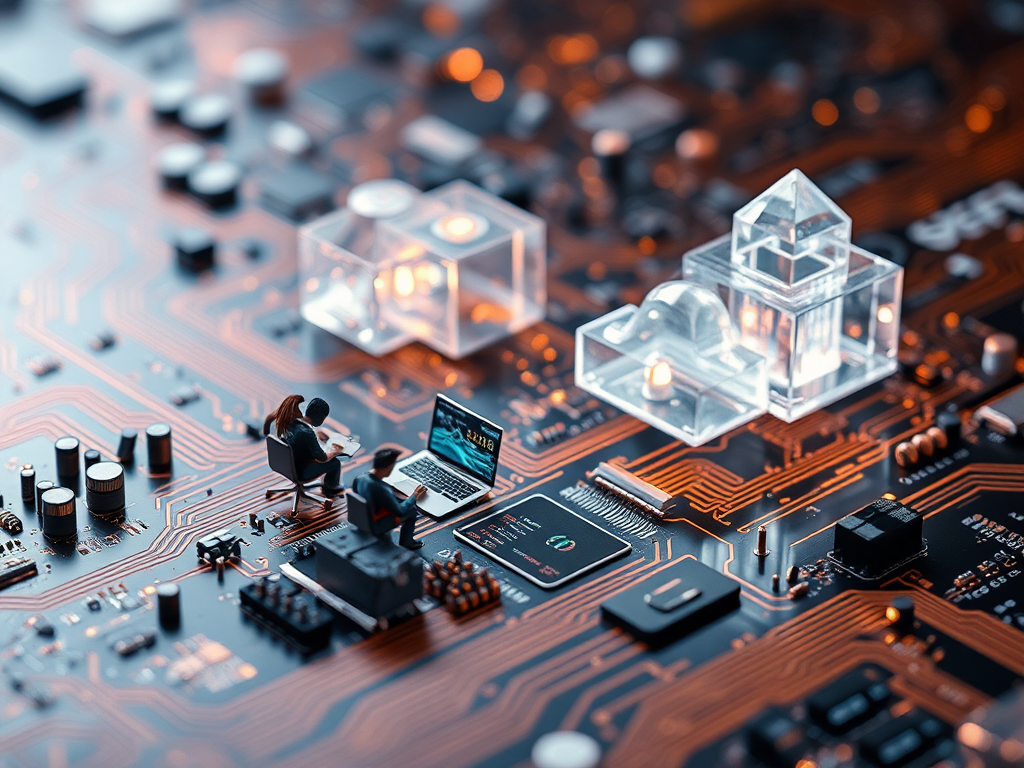