Hello, Python enthusiasts! Today, let's dive into a love-hate topic—asynchronous programming. Are you often tormented by blocking operations? Don't worry; asynchronous programming was born to solve this problem. Let's explore how to make your Python code as free as the wind!
Why Asynchronous
Imagine you're cooking a pot of soup. If you only know synchronous cooking, you would just stand there staring at the soup. But if you know asynchronous cooking, you can cut vegetables, wash dishes, or even check your phone while the soup cooks. That's the charm of asynchronous programming!
In the programming world, we often encounter time-consuming operations like network requests and file I/O. If our programs execute these operations one by one, most of the time will be wasted waiting. Asynchronous programming allows us to perform other tasks while waiting for these operations to complete, greatly improving program efficiency.
Coroutines: The Soul of Asynchronous
When it comes to asynchronous programming, we must mention coroutines. What are coroutines? You can think of them as functions that can be paused and resumed. When a coroutine encounters a time-consuming operation, it can voluntarily yield control, allowing other coroutines to execute. This mechanism lets us write asynchronous code in a synchronous manner, ensuring readability while improving efficiency.
Python 3.5 introduced the async
and await
keywords, making coroutine definition and usage more intuitive. Let's look at a simple example:
import asyncio
async def say_hello(name):
await asyncio.sleep(1) # Simulate a time-consuming operation
print(f"Hello, {name}!")
async def main():
await asyncio.gather(
say_hello("Alice"),
say_hello("Bob"),
say_hello("Charlie")
)
asyncio.run(main())
In this example, the say_hello
function is defined as a coroutine. It uses the await
keyword to wait for an asynchronous operation (asyncio.sleep
) to complete. The main
function uses asyncio.gather
to start multiple coroutines simultaneously.
Guess how long this code takes to run? If executed synchronously, it would take 3 seconds (each say_hello
needs 1 second). But in reality, it only takes about 1 second! That's the power of asynchronous programming.
Event Loop: The Heart of Asynchronous
The core of asynchronous programming is the event loop. You can think of the event loop as a tireless waiter, constantly moving between tables to see which customer (coroutine) needs service.
In Python, the asyncio
module provides an implementation of the event loop. When we call asyncio.run(main())
, Python creates an event loop and runs the main
coroutine in it.
The event loop works roughly as follows:
- Start a coroutine
- Run the coroutine until it encounters
await
- Return control to the event loop
- The event loop selects the next coroutine to run
- Repeat steps 2-4 until all coroutines are complete
This process seems complex, but fortunately, Python handles all the details for us. We just need to focus on writing coroutines and leave the rest to asyncio
!
Asynchronous I/O: The Source of Efficiency
The most common application of asynchronous programming is I/O-intensive tasks. For example, if we need to fetch data from multiple websites, using a synchronous approach means waiting for each request to complete before starting the next one. But with asynchronous programming, we can initiate multiple requests simultaneously, greatly reducing total wait time.
Let's look at a practical example:
import asyncio
import aiohttp
async def fetch_url(session, url):
async with session.get(url) as response:
return await response.text()
async def main():
urls = [
"https://api.github.com",
"https://api.github.com/events",
"https://api.github.com/repos/python/cpython"
]
async with aiohttp.ClientSession() as session:
tasks = [fetch_url(session, url) for url in urls]
results = await asyncio.gather(*tasks)
for url, result in zip(urls, results):
print(f"Fetched {len(result)} bytes from {url}")
asyncio.run(main())
In this example, we use the aiohttp
library to asynchronously make HTTP requests. Notice how we use the async with
statement to manage the lifecycle of the session and response objects. This program can initiate multiple requests simultaneously, significantly improving efficiency.
Asynchronous Context Managers
Speaking of async with
, we must mention asynchronous context managers. They work similarly to regular context managers but can perform asynchronous operations upon entering and exiting the context.
Let's implement a simple asynchronous context manager:
import asyncio
class AsyncTimer:
def __init__(self, name):
self.name = name
async def __aenter__(self):
self.start = asyncio.get_event_loop().time()
print(f"Starting {self.name}")
return self
async def __aexit__(self, exc_type, exc_val, exc_tb):
end = asyncio.get_event_loop().time()
print(f"{self.name} took {end - self.start:.2f} seconds")
async def main():
async with AsyncTimer("Task 1"):
await asyncio.sleep(1)
async with AsyncTimer("Task 2"):
await asyncio.sleep(2)
asyncio.run(main())
The AsyncTimer
class helps measure the execution time of asynchronous operations. Note how the __aenter__
and __aexit__
methods are defined. This pattern is useful in scenarios such as resource management and performance measurement.
Asynchronous Generators
Asynchronous generators are another powerful tool. They allow us to generate a series of values asynchronously. Let's look at an example:
import asyncio
async def countdown(n):
while n > 0:
yield n
await asyncio.sleep(1)
n -= 1
async def main():
async for i in countdown(5):
print(i)
asyncio.run(main())
The countdown
function is an asynchronous generator. It generates a number every second until it counts down to 0. Note how we use async for
to iterate over this asynchronous generator.
Asynchronous Iterators
Closely related to asynchronous generators are asynchronous iterators. Any object implementing the __aiter__
and __anext__
methods can be considered an asynchronous iterator. Let's implement a simple asynchronous iterator:
import asyncio
class AsyncRange:
def __init__(self, start, stop):
self.start = start
self.stop = stop
def __aiter__(self):
return self
async def __anext__(self):
if self.start >= self.stop:
raise StopAsyncIteration
value = self.start
self.start += 1
await asyncio.sleep(1)
return value
async def main():
async for i in AsyncRange(0, 5):
print(i)
asyncio.run(main())
The AsyncRange
class mimics the built-in range
function, but it is asynchronous and pauses for one second between generating each number.
Concurrency vs. Parallelism
At this point, we need to clarify a common misconception: asynchronous programming is not the same as parallel processing. Asynchronous programming is about organizing and managing concurrent tasks, while parallel processing is about executing multiple tasks simultaneously.
In Python, due to the Global Interpreter Lock (GIL), the standard CPython interpreter cannot truly execute Python code in parallel on multicore systems. However, for I/O-intensive tasks, asynchronous programming can still provide significant performance improvements because it allows the program to perform other tasks while waiting for I/O operations.
If you need true parallel computation, consider using multiprocessing or other Python implementations (like Jython or IronPython).
Asynchronous Pitfalls
While asynchronous programming is powerful, it also brings new challenges. Here are some common pitfalls:
-
Forgetting to use await: If you call a coroutine from another coroutine but forget to use
await
, the call won't actually execute. -
Blocking the event loop: If you perform a long-running synchronous operation in a coroutine, it will block the entire event loop, preventing other coroutines from executing.
-
Concurrency control: When multiple coroutines access shared resources simultaneously, you need to carefully handle concurrency issues.
asyncio
provides tools likeLock
andSemaphore
to help manage concurrency. -
Exception handling: Exception handling in asynchronous code becomes more complex. You need to ensure all exceptions are properly caught and handled.
-
Debugging difficulty: The execution order of asynchronous code may not be intuitive, making debugging difficult.
To avoid these pitfalls, I recommend following these best practices:
- Always use
await
to call coroutines. - Use asynchronous versions of potentially blocking operations (e.g.,
aiohttp
instead ofrequests
). - Use concurrency primitives provided by
asyncio
to manage shared resources. - Take full advantage of
try/except
statements to handle exceptions. - Use
asyncio.create_task()
to create and manage tasks.
Practical: Asynchronous Web Crawler
Let's apply what we've learned to a practical project! We'll implement a simple asynchronous web crawler that can crawl multiple web pages simultaneously.
import asyncio
import aiohttp
from bs4 import BeautifulSoup
async def fetch_url(session, url):
async with session.get(url) as response:
return await response.text()
async def parse_html(html):
soup = BeautifulSoup(html, 'html.parser')
return soup.title.string if soup.title else "No title"
async def crawl(url):
async with aiohttp.ClientSession() as session:
html = await fetch_url(session, url)
title = await parse_html(html)
print(f"Title of {url}: {title}")
async def main():
urls = [
"https://www.python.org",
"https://github.com",
"https://stackoverflow.com",
"https://www.google.com",
"https://www.bbc.com"
]
tasks = [asyncio.create_task(crawl(url)) for url in urls]
await asyncio.gather(*tasks)
asyncio.run(main())
This crawler can simultaneously fetch multiple web pages and extract the title of each page. Notice how we use asyncio.create_task()
to create tasks and asyncio.gather()
to wait for all tasks to complete.
Conclusion
Asynchronous programming is a powerful tool that helps us write efficient I/O-intensive programs. While the learning curve might be steep, once mastered, you can write highly performant Python programs.
Remember, asynchronous programming is not a silver bullet. It might not provide significant performance gains for CPU-intensive tasks. However, for I/O-intensive tasks, especially those requiring handling many concurrent connections, asynchronous programming can greatly enhance program efficiency.
What are your thoughts on asynchronous programming? Have you used it in real projects? Feel free to share your experiences and thoughts in the comments!
Finally, let's summarize today's content in one sentence: Asynchronous programming is like giving your code wings, allowing it to soar freely over the sea of I/O. Now it's your turn to try. Go ahead, let your code fly!
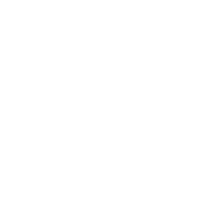
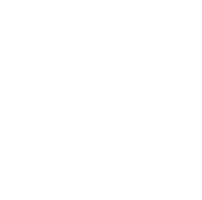
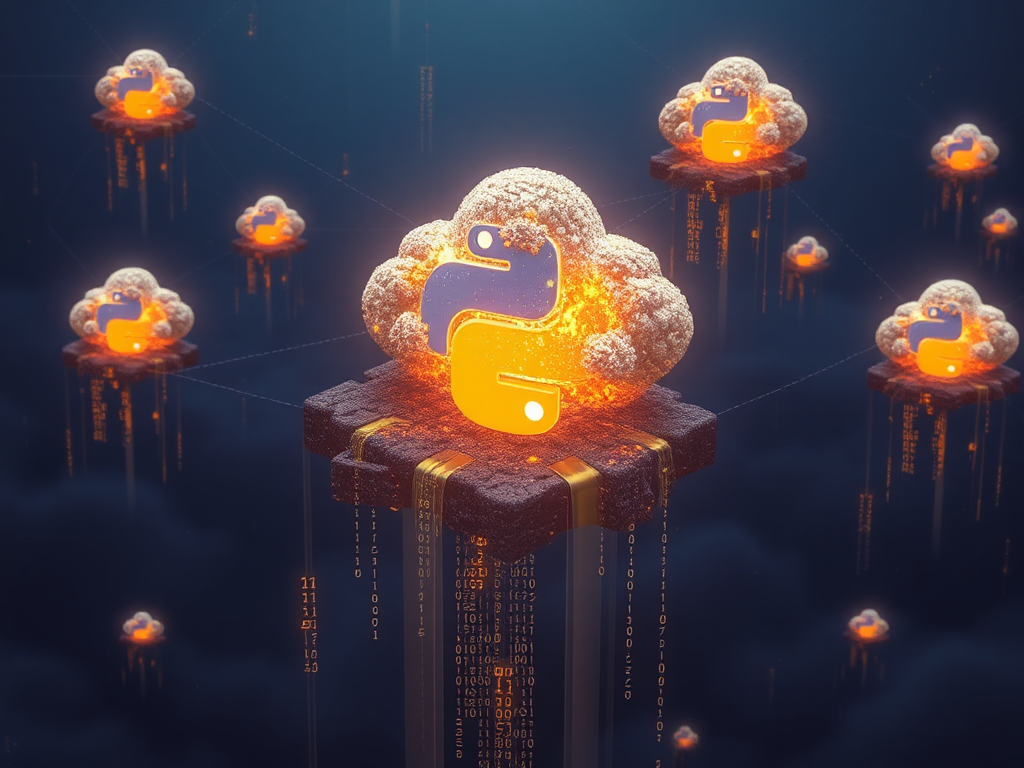
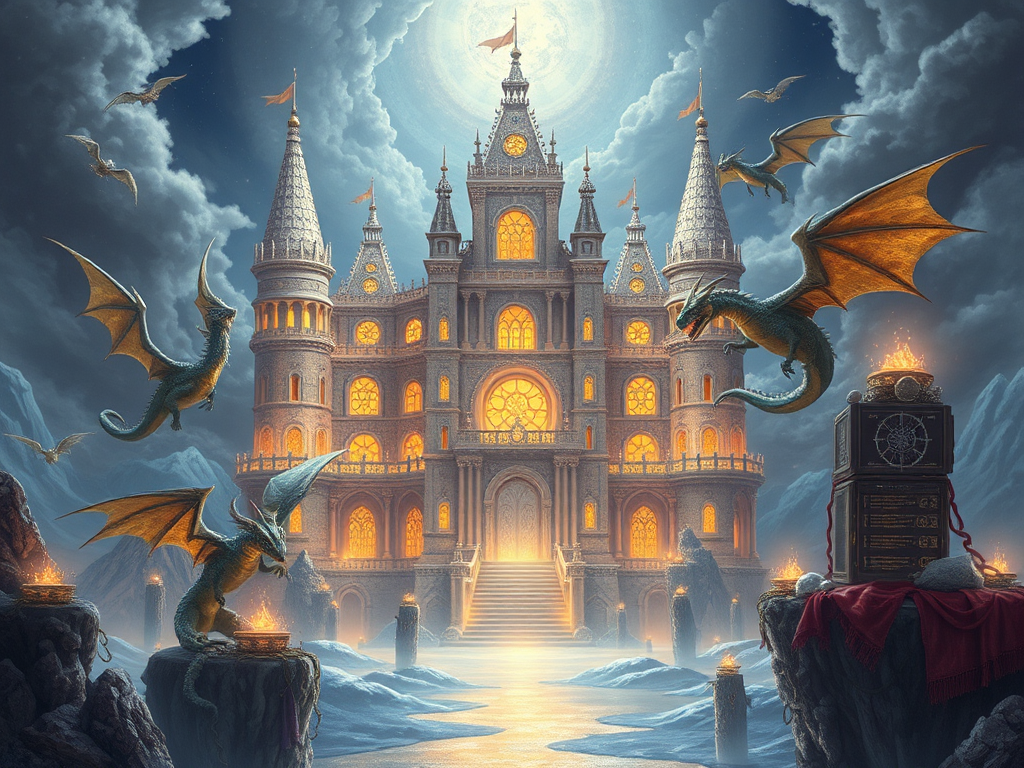
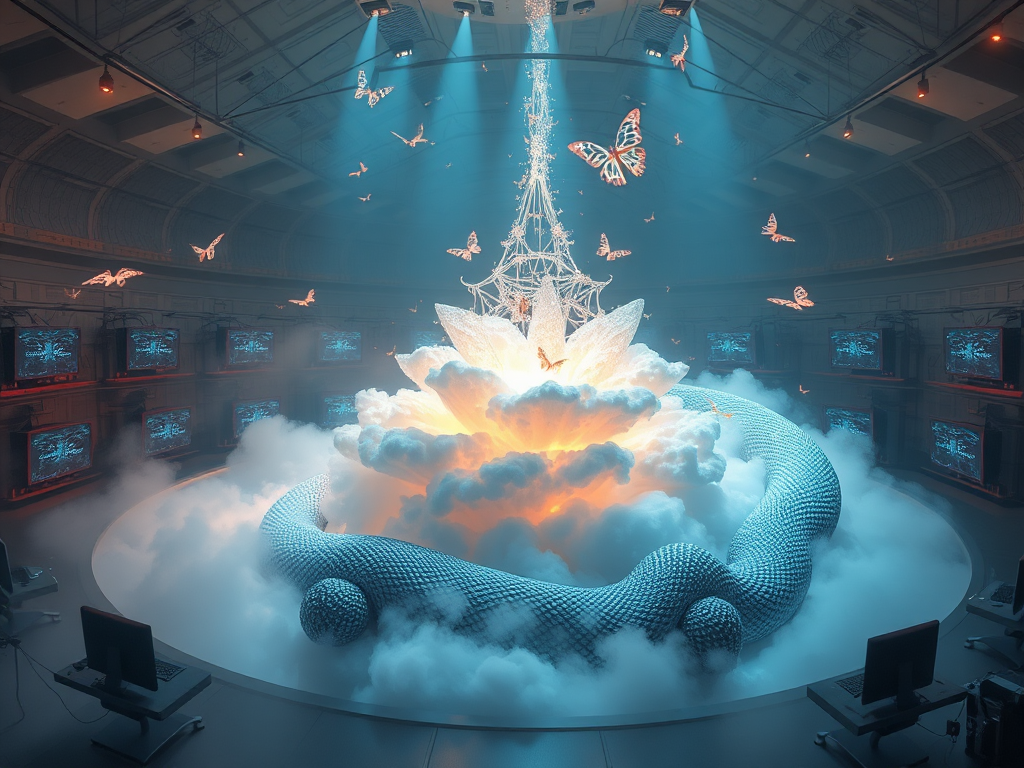