Origin
Have you ever experienced this? Clicking around various cloud platform consoles daily, creating virtual machines, configuring networks, managing storage... These repetitive operations not only consume time and energy but are also prone to errors. As a Python developer, I deeply felt this pain point. Until one day, I discovered that Python could liberate us from these repetitive tasks, which led me to embark on an amazing journey of automation operations.
Exploration
Speaking of cloud computing, you might think of major cloud service providers like AWS, Google Cloud, and Azure. But did you know? These platforms all provide powerful Python SDKs that allow us to manage cloud resources through code. Take AWS's boto3 for example - it's like a magical key that enables us to complete complex cloud resource management tasks with simple Python code.
Let's look at a practical example. Suppose you need to dynamically create and destroy EC2 instances based on business load. Traditionally, you would need to log into the console and operate manually, but with Python code, it can be easily achieved:
import boto3
import time
def create_ec2_instance():
ec2 = boto3.client('ec2')
response = ec2.run_instances(
ImageId='ami-0c55b159cbfafe1f0',
InstanceType='t2.micro',
MinCount=1,
MaxCount=1,
TagSpecifications=[
{
'ResourceType': 'instance',
'Tags': [
{
'Key': 'Name',
'Value': 'AutoScaledInstance'
},
]
},
]
)
return response['Instances'][0]['InstanceId']
def monitor_and_scale():
cloudwatch = boto3.client('cloudwatch')
# Get CPU utilization metrics
response = cloudwatch.get_metric_statistics(
Namespace='AWS/EC2',
MetricName='CPUUtilization',
Dimensions=[{'Name': 'InstanceId', 'Value': instance_id}],
StartTime=time.time() - 300,
EndTime=time.time(),
Period=300,
Statistics=['Average']
)
# Decide whether to scale based on CPU utilization
if response['Datapoints'][0]['Average'] > 80:
new_instance_id = create_ec2_instance()
Wonder how this code works? Let me explain. This code first creates an EC2 client, then launches a new instance using predefined parameters. Even better, it includes a monitoring function that can check CPU utilization in real-time and automatically trigger scaling when the load is too high. This is the charm of Infrastructure as Code.
Practice
In actual work, I found that cloud computing applications go far beyond this. Let me share some experiences I've summarized from practice.
First is the application of serverless computing (FaaS). I remember once we needed to process large amounts of user-uploaded images, requiring automatic resizing and thumbnail generation. The traditional approach would require deploying dedicated servers, but with AWS Lambda and Python, we only need to write a simple function:
import boto3
from PIL import Image
import io
def lambda_handler(event, context):
s3_client = boto3.client('s3')
bucket = event['Records'][0]['s3']['bucket']['name']
key = event['Records'][0]['s3']['object']['key']
# Download original image
response = s3_client.get_object(Bucket=bucket, Key=key)
image_data = response['Body'].read()
# Process image
image = Image.open(io.BytesIO(image_data))
thumbnail_size = (200, 200)
image.thumbnail(thumbnail_size)
# Save thumbnail
buffer = io.BytesIO()
image.save(buffer, format=image.format)
thumbnail_key = f"thumbnails/{key}"
s3_client.put_object(
Bucket=bucket,
Key=thumbnail_key,
Body=buffer.getvalue()
)
In terms of data processing, Python's advantages are even more significant. I once was responsible for a project that needed to process massive log data, and easily implemented data cleaning and analysis using Python's Pandas library combined with cloud storage:
import pandas as pd
import boto3
from io import StringIO
def process_logs():
s3 = boto3.client('s3')
# Read log file from S3
response = s3.get_object(Bucket='logs-bucket', Key='app-logs.csv')
data = response['Body'].read().decode('utf-8')
# Process data using Pandas
df = pd.read_csv(StringIO(data))
# Data cleaning and analysis
df['timestamp'] = pd.to_datetime(df['timestamp'])
daily_stats = df.groupby(df['timestamp'].dt.date).agg({
'user_id': 'count',
'response_time': 'mean'
})
# Save processed results back to S3
buffer = StringIO()
daily_stats.to_csv(buffer)
s3.put_object(
Bucket='processed-logs',
Key='daily-stats.csv',
Body=buffer.getvalue()
)
Gains
Through this period of practice, I deeply experienced Python's powerful capabilities in the cloud computing era. It not only simplifies infrastructure management but also opens up a new world of automated operations. For example, we can use Python to:
- Automate resource configuration: Automatically create and configure cloud resources based on predefined templates
- Intelligent monitoring and alerting: Monitor system status in real-time, automatically handle or alert on anomalies
- Cost optimization: Find the best resource usage solutions through data analysis, automatically shut down idle resources
- Security compliance: Automatically scan for security vulnerabilities, ensure system compliance with security standards
In this process, I also summarized some experiences. First, thoroughly understand the cloud service provider's API documentation, which is the foundation for writing reliable automation scripts. Second, pay attention to error handling and logging, as problems in cloud environments are often harder to troubleshoot than local development. Finally, make good use of the monitoring and alerting functions provided by cloud platforms to discover and solve problems in a timely manner.
Have you thought about how the nature of operations work is changing with the development of cloud computing? From manual operations to automation scripts, from passive response to proactive prevention, Python is helping us redefine the way we do operations work. This not only improves efficiency but also gives us more time to focus on more valuable things.
So, are you ready to start your Python cloud computing journey? Welcome to share your thoughts and experiences in the comments.
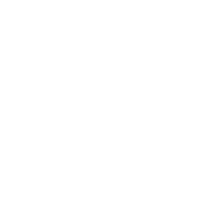
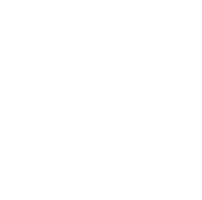
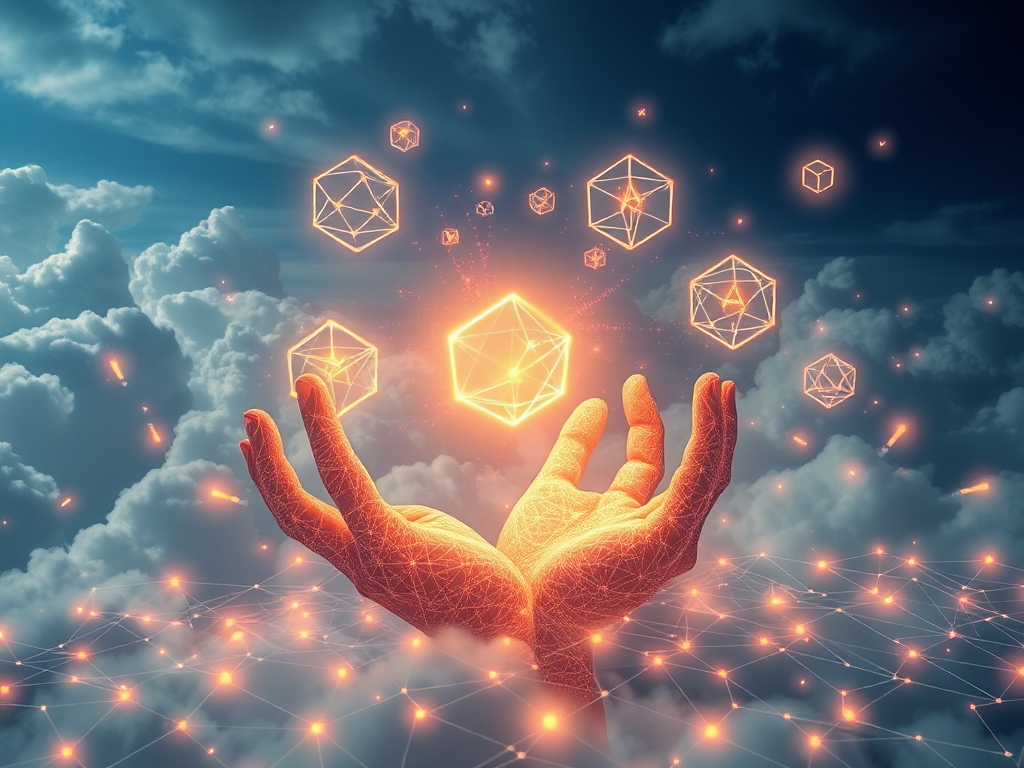
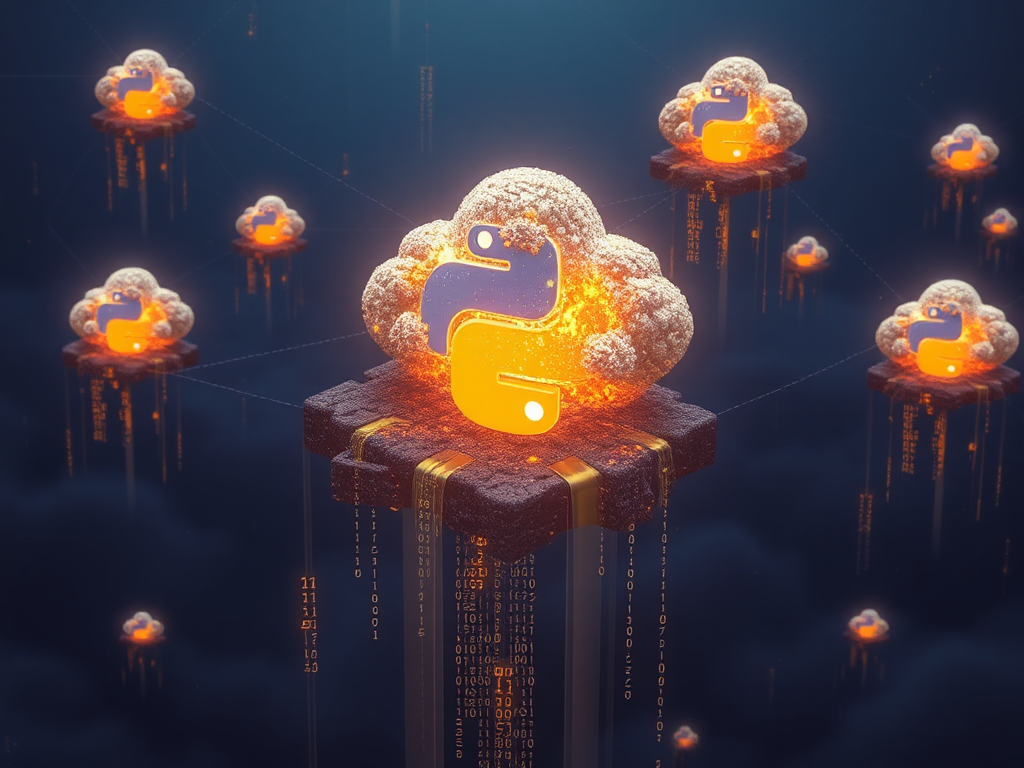
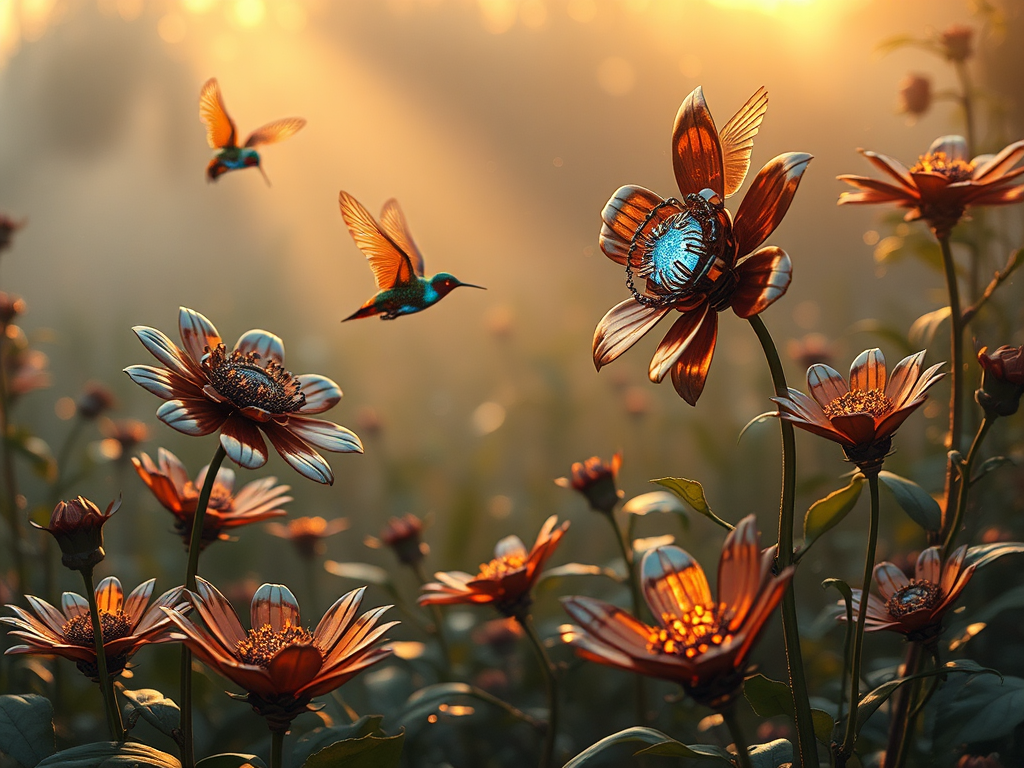