Origins
Have you encountered scenarios where you need to add logging, performance monitoring, or permission checks to business code, but don't want to mix these "cross-cutting concerns" with core business logic? This is exactly the problem that the decorator pattern solves. As a Python developer, I've found that decorators not only elegantly implement code reuse but are also excellent tools for Aspect-Oriented Programming (AOP). Let's dive deep into this powerful feature.
Basic Knowledge
Before explaining decorators, we need to understand some basic concepts in Python. Functions in Python are first-class citizens, meaning they can be passed around and used like regular variables. Let's look at a simple example:
def greet(name):
return f"Hello, {name}"
my_func = greet
print(my_func("Python")) # Output: Hello, Python
def execute_func(func, param):
return func(param)
result = execute_func(greet, "World")
print(result) # Output: Hello, World
See that? Functions can be assigned and passed around like regular objects. This feature lays the foundation for implementing decorators.
Evolution
How did the design concept of decorators evolve? Let's trace its development process.
Initially, when we needed to add some common logic before and after function execution, we might write:
def business_logic():
print("Executing business logic")
def business_logic_with_log():
print("Starting execution")
business_logic()
print("Execution completed")
The problem with this approach is obvious - we modified the original function, violating the Open-Closed Principle. If multiple functions need logging, we would have to repeat similar code.
Later, we learned to use higher-order functions:
def add_logging(func):
def wrapper():
print("Starting execution")
func()
print("Execution completed")
return wrapper
def business_logic():
print("Executing business logic")
business_logic = add_logging(business_logic)
This approach is more elegant, but the calling syntax isn't very intuitive. To simplify this process, Python introduced decorator syntax:
@add_logging
def business_logic():
print("Executing business logic")
Implementation Details
A decorator is essentially a callable object (usually a function) that takes a function as a parameter and returns a new function. Let's explore several common decorator implementation methods:
- Function decorator without parameters:
def timing_decorator(func):
def wrapper(*args, **kwargs):
import time
start = time.time()
result = func(*args, **kwargs)
end = time.time()
print(f"Function {func.__name__} execution time: {end - start:.4f} seconds")
return result
return wrapper
@timing_decorator
def slow_function():
import time
time.sleep(1)
print("Function execution completed")
- Decorator with parameters:
def retry(max_attempts=3, delay_seconds=1):
def decorator(func):
def wrapper(*args, **kwargs):
for attempt in range(max_attempts):
try:
return func(*args, **kwargs)
except Exception as e:
if attempt == max_attempts - 1:
raise e
import time
time.sleep(delay_seconds)
return None
return wrapper
return decorator
@retry(max_attempts=3, delay_seconds=2)
def unstable_network_call():
import random
if random.random() < 0.7:
raise ConnectionError("Network connection failed")
return "Successfully retrieved data"
Application Scenarios
In practical development, decorators have a wide range of applications. Here are some scenarios I frequently use:
- Performance Monitoring
In large systems, performance monitoring is essential. We can use decorators to collect function execution times:
def performance_monitor(func):
def wrapper(*args, **kwargs):
import time
start = time.perf_counter()
result = func(*args, **kwargs)
duration = time.perf_counter() - start
# Performance data can be sent to monitoring system here
print(f"Performance data: function={func.__name__}, duration={duration:.4f}s")
return result
return wrapper
@performance_monitor
def process_large_data(data_size):
import time
time.sleep(data_size / 1000) # Simulating data processing
- Cache Optimization
For computation-intensive or network request operations, we can implement caching using decorators:
def memoize(func):
cache = {}
def wrapper(*args):
if args not in cache:
cache[args] = func(*args)
return cache[args]
return wrapper
@memoize
def fibonacci(n):
if n < 2:
return n
return fibonacci(n-1) + fibonacci(n-2)
- Permission Control
In web applications, permission control is a common requirement:
def require_permission(permission):
def decorator(func):
def wrapper(*args, **kwargs):
# Assuming current_user is a global object
if not hasattr(wrapper, 'current_user'):
raise ValueError("Not logged in")
if permission not in wrapper.current_user.permissions:
raise ValueError("Insufficient permissions")
return func(*args, **kwargs)
return wrapper
return decorator
@require_permission("admin")
def delete_user(user_id):
print(f"Deleting user: {user_id}")
Important Considerations
When using decorators, there are some details that need special attention:
- Function Signature Issue
Decorators will change the original function's signature, which might affect tools or frameworks that depend on function signatures. The solution is to use functools.wraps:
from functools import wraps
def my_decorator(func):
@wraps(func)
def wrapper(*args, **kwargs):
return func(*args, **kwargs)
return wrapper
@my_decorator
def example():
"""This is the example function's docstring"""
pass
print(example.__name__) # Output: example
print(example.__doc__) # Output: This is the example function's docstring
- Execution Order
When multiple decorators are used together, the execution order is from bottom to top:
def decorator1(func):
def wrapper():
print("decorator1")
return func()
return wrapper
def decorator2(func):
def wrapper():
print("decorator2")
return func()
return wrapper
@decorator1
@decorator2
def hello():
print("hello")
- Performance Overhead
Decorators add overhead to function calls. For frequently called small functions, this overhead might become significant:
def heavy_decorator(func):
def wrapper(*args, **kwargs):
# Some time-consuming operations here
import time
time.sleep(0.1) # Simulating overhead
return func(*args, **kwargs)
return wrapper
@heavy_decorator
def light_function():
return 1 + 1
Advanced Techniques
For those who have mastered the basics, I want to share some advanced decorator techniques:
- Class Decorators
Besides function decorators, Python also supports class decorators:
class CountCalls:
def __init__(self, func):
self.func = func
self.count = 0
def __call__(self, *args, **kwargs):
self.count += 1
print(f"Function {self.func.__name__} has been called {self.count} times")
return self.func(*args, **kwargs)
@CountCalls
def say_hello():
print("Hello!")
- Reusable Decorator Factory
Creating a generic decorator factory that can generate decorators based on different needs:
def create_decorator(pre_action=None, post_action=None):
def decorator(func):
@wraps(func)
def wrapper(*args, **kwargs):
if pre_action:
pre_action()
result = func(*args, **kwargs)
if post_action:
post_action()
return result
return wrapper
return decorator
def log_start():
print("Starting execution")
def log_end():
print("Execution completed")
@create_decorator(pre_action=log_start, post_action=log_end)
def business_function():
print("Executing business logic")
- Decorator Chains
Combining multiple decorators to implement complex functionality:
def validate_input(func):
@wraps(func)
def wrapper(*args, **kwargs):
if not args and not kwargs:
raise ValueError("Parameters cannot be empty")
return func(*args, **kwargs)
return wrapper
def log_exceptions(func):
@wraps(func)
def wrapper(*args, **kwargs):
try:
return func(*args, **kwargs)
except Exception as e:
print(f"Exception: {str(e)}")
raise
return wrapper
@validate_input
@log_exceptions
def process_data(data):
# Code to process data
pass
Practical Experience
In my practical project experience, decorators are often combined with other Python features to achieve more powerful functionality. For example:
- Combining with Context Managers:
from contextlib import contextmanager
@contextmanager
def transaction():
print("Starting transaction")
try:
yield
print("Committing transaction")
except Exception:
print("Rolling back transaction")
raise
def transactional(func):
def wrapper(*args, **kwargs):
with transaction():
return func(*args, **kwargs)
return wrapper
@transactional
def update_database():
print("Updating database")
# If an exception occurs here, the transaction will automatically rollback
- Combining with Descriptors:
class ValidateType:
def __init__(self, type_):
self.type_ = type_
def __call__(self, func):
def wrapper(*args, **kwargs):
for arg in args:
if not isinstance(arg, self.type_):
raise TypeError(f"Parameter type must be {self.type_}")
return func(*args, **kwargs)
return wrapper
@ValidateType(str)
def process_strings(*strings):
return "".join(strings)
Development Trends
As Python language evolves, decorator applications continue to advance. In Python 3.9+, we see more new features and best practices regarding decorators:
- Type Annotation Support:
from typing import Callable, TypeVar, ParamSpec
P = ParamSpec('P')
R = TypeVar('R')
def logged(func: Callable[P, R]) -> Callable[P, R]:
def wrapper(*args: P.args, **kwargs: P.kwargs) -> R:
print(f"Calling function: {func.__name__}")
return func(*args, **kwargs)
return wrapper
- Async Decorators:
import asyncio
from functools import wraps
def async_retry(max_attempts: int = 3):
def decorator(func):
@wraps(func)
async def wrapper(*args, **kwargs):
for attempt in range(max_attempts):
try:
return await func(*args, **kwargs)
except Exception as e:
if attempt == max_attempts - 1:
raise
await asyncio.sleep(2 ** attempt)
return None
return wrapper
return decorator
@async_retry(max_attempts=3)
async def fetch_data():
# Async network request
pass
Future Outlook
Looking ahead, I believe decorators will play a bigger role in the following areas:
- Service registration and discovery in microservice architecture
- Promotion of functional programming paradigm
- Implementation of code-as-configuration concept
- Smarter performance optimization and monitoring
- More powerful type checking and runtime validation
Summary
Through this article, we've explored Python decorators comprehensively. From basic concepts to advanced applications, from implementation details to best practices, I believe you now have a thorough understanding of decorators.
In practical development, decorators not only help us write more concise and maintainable code but also elegantly solve cross-cutting concerns. Remember, the core idea of decorators is to add new functionality to functions or classes without modifying the original code.
What other interesting applications of decorators can you think of? Feel free to share your thoughts and experiences in the comments. If you want to learn more about Python programming, see you next time.
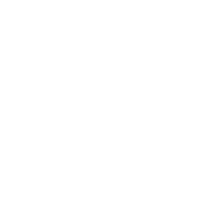
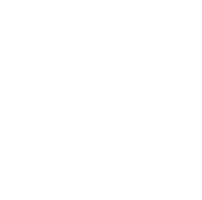
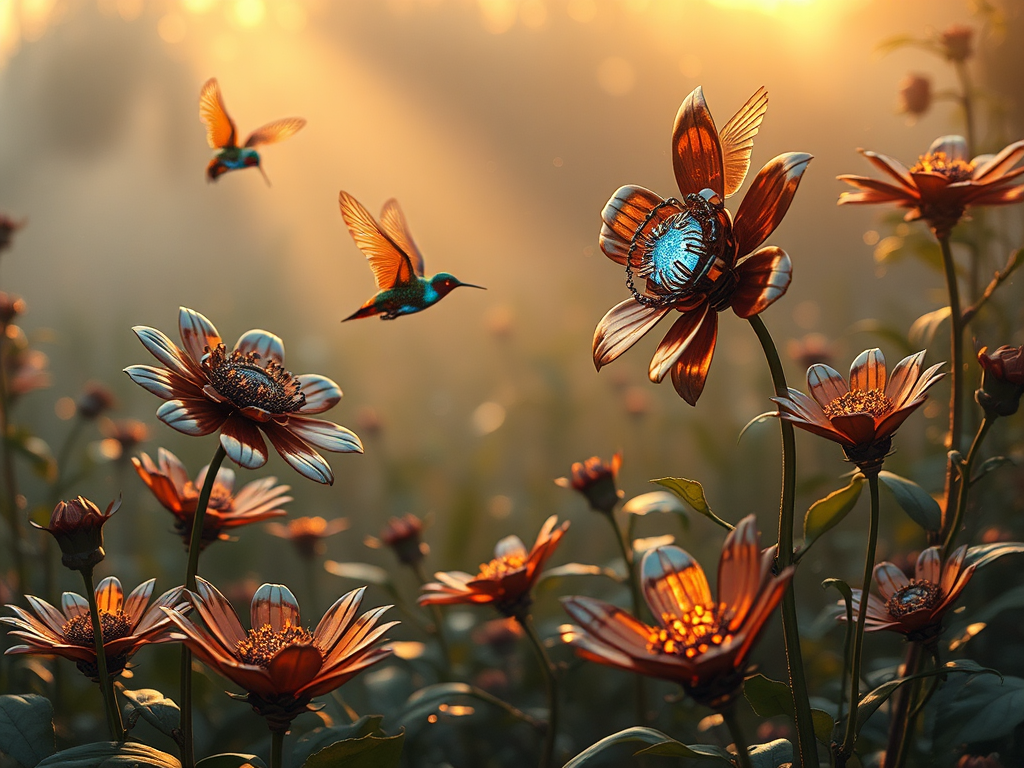
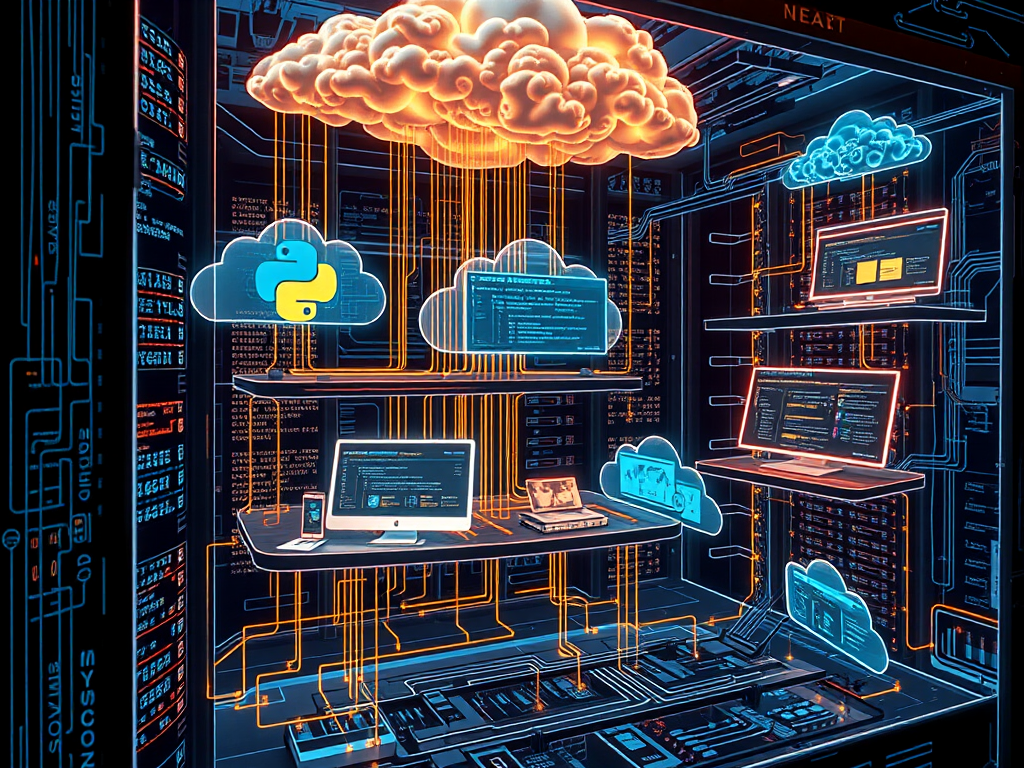
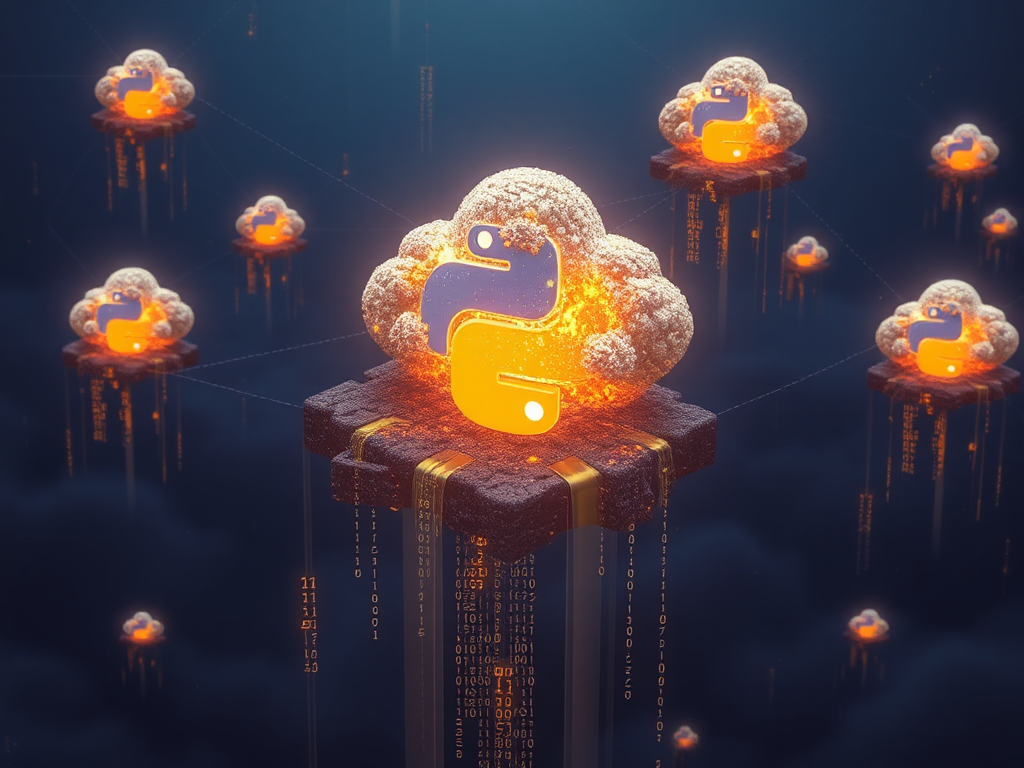