Introduction
Do you often wonder why some developers can easily navigate cloud computing while you struggle just to set up the environment? Today, let's uncover the mysteries of Python cloud development and explore a smoother learning path.
Environment Setup
Before you start coding, you need to set up your "home." I remember facing many hurdles with environment setup when I first started learning cloud development. Looking back, if someone had shared these tips with me, I might have avoided many detours.
When setting up a Python development environment, I recommend using a virtual environment first. Why? It prevents dependency conflicts between different projects. You can create one like this:
python -m venv cloud_dev
source cloud_dev/bin/activate # Linux/Mac
cloud_dev\Scripts\activate.bat # Windows
Storage Practice
In cloud development, storage services are arguably the most fundamental and commonly used features. Have you ever encountered a situation where everything works fine locally, but issues arise when deployed to the cloud? This often happens due to incorrect handling of storage paths.
Let's look at a more complete cloud storage example:
from google.cloud import storage
import os
class CloudStorage:
def __init__(self, bucket_name):
self.storage_client = storage.Client()
self.bucket = self.storage_client.bucket(bucket_name)
def upload_file(self, source_path, destination_blob_name):
blob = self.bucket.blob(destination_blob_name)
blob.upload_from_filename(source_path)
return blob.public_url
def download_file(self, source_blob_name, destination_path):
blob = self.bucket.blob(source_blob_name)
os.makedirs(os.path.dirname(destination_path), exist_ok=True)
blob.download_to_filename(destination_path)
def list_files(self, prefix=None):
return [blob.name for blob in self.bucket.list_blobs(prefix=prefix)]
Wondering what this code does? It creates a complete cloud storage management class with upload, download, and list functions. I particularly like this encapsulation style because it makes the code easier to maintain and expand.
API Development
In cloud development, the design and implementation of API interfaces is another important topic. Did you know? A well-designed API can greatly enhance the usability and maintainability of an application.
Let's look at a more practical API example:
from fastapi import FastAPI, HTTPException
from pydantic import BaseModel
import uvicorn
app = FastAPI()
class Item(BaseModel):
name: str
price: float
is_offer: bool = None
@app.get("/items/{item_id}")
async def read_item(item_id: int):
try:
# Add database query logic here
return {"item_id": item_id, "name": "Sample Item"}
except Exception as e:
raise HTTPException(status_code=404, detail="Item not found")
@app.post("/items/")
async def create_item(item: Item):
try:
# Add database storage logic here
return {"status": "success", "item": item}
except Exception as e:
raise HTTPException(status_code=400, detail="Failed to create item")
if __name__ == "__main__":
uvicorn.run(app, host="0.0.0.0", port=8000)
Monitoring Solutions
When it comes to cloud application operations, monitoring is of utmost importance. Have you ever been woken up by a phone call in the middle of the night saying the system is down? With a good monitoring solution, many issues can be detected and prevented in advance.
Here's a snippet of monitoring code I often use:
import logging
from datetime import datetime
from google.cloud import monitoring_v3
class CloudMonitor:
def __init__(self, project_id):
self.client = monitoring_v3.MetricServiceClient()
self.project_name = f"projects/{project_id}"
def write_metric(self, metric_type, value, resource_type="global"):
time_series = monitoring_v3.TimeSeries()
time_series.metric.type = f"custom.googleapis.com/{metric_type}"
time_series.resource.type = resource_type
time_series.resource.labels = {
"project_id": self.project_name.split('/')[-1]
}
point = time_series.points.add()
point.value.double_value = value
point.interval.end_time.seconds = int(datetime.now().timestamp())
self.client.create_time_series(
request={
"name": self.project_name,
"time_series": [time_series]
}
)
Security Protection
In cloud development, security is always the top priority. Did you know? Statistics show that over 60% of security vulnerabilities stem from improper authentication and authorization handling. Let me share an example of security practice:
from functools import wraps
from google.oauth2 import id_token
from google.auth.transport import requests
import jwt
from datetime import datetime, timedelta
class SecurityManager:
def __init__(self, secret_key):
self.secret_key = secret_key
def create_token(self, user_id):
payload = {
'user_id': user_id,
'exp': datetime.utcnow() + timedelta(days=1)
}
return jwt.encode(payload, self.secret_key, algorithm='HS256')
def verify_token(self, token):
try:
payload = jwt.decode(token, self.secret_key, algorithms=['HS256'])
return payload['user_id']
except jwt.ExpiredSignatureError:
return None
except jwt.InvalidTokenError:
return None
def require_auth(f):
@wraps(f)
def decorated(*args, **kwargs):
token = request.headers.get('Authorization')
if not token:
return {'message': 'Missing token'}, 401
security = SecurityManager('your-secret-key')
user_id = security.verify_token(token)
if not user_id:
return {'message': 'Invalid token'}, 401
return f(*args, **kwargs)
return decorated
Best Practices
Through many years of cloud development experience, I've summarized some best practices. Do you find these suggestions useful?
- Always use configuration files to manage environment variables
- Implement comprehensive error handling mechanisms
- Establish a unified logging system
- Use asynchronous operations to improve performance
- Implement a graceful service degradation mechanism
Let's look at a specific configuration management example:
import yaml
import os
from pathlib import Path
class Config:
_instance = None
def __new__(cls):
if cls._instance is None:
cls._instance = super().__new__(cls)
cls._instance._load_config()
return cls._instance
def _load_config(self):
env = os.getenv('ENVIRONMENT', 'development')
config_path = Path(__file__).parent / f"config/{env}.yaml"
with open(config_path) as f:
self.config = yaml.safe_load(f)
def get(self, key, default=None):
return self.config.get(key, default)
Performance Optimization
Performance optimization is an eternal topic. Did you know? Often, performance issues do not stem from the code itself but from how resources are used.
Here's an example of asynchronous operations:
import asyncio
from aiohttp import ClientSession
import time
async def fetch_url(session, url):
async with session.get(url) as response:
return await response.text()
async def main():
urls = [
'http://api1.example.com',
'http://api2.example.com',
'http://api3.example.com'
]
async with ClientSession() as session:
tasks = [fetch_url(session, url) for url in urls]
results = await asyncio.gather(*tasks)
return results
if __name__ == "__main__":
start = time.time()
results = asyncio.run(main())
print(f"Time taken: {time.time() - start} seconds")
Debugging Tips
Do you find debugging code in a cloud environment particularly challenging? Here, I'll share a remote debugging solution I often use:
import logging
import sys
from datetime import datetime
class CloudDebugger:
def __init__(self, log_file=None):
self.logger = logging.getLogger('cloud_debugger')
self.logger.setLevel(logging.DEBUG)
formatter = logging.Formatter(
'%(asctime)s - %(name)s - %(levelname)s - %(message)s'
)
# Console handler
ch = logging.StreamHandler(sys.stdout)
ch.setFormatter(formatter)
self.logger.addHandler(ch)
# File handler
if log_file:
fh = logging.FileHandler(log_file)
fh.setFormatter(formatter)
self.logger.addHandler(fh)
def debug(self, message):
self.logger.debug(message)
def info(self, message):
self.logger.info(message)
def error(self, message, exc_info=True):
self.logger.error(message, exc_info=exc_info)
Conclusion and Outlook
Through this article, we've explored the core knowledge and practical experience of Python cloud development together. Do you find this content helpful?
Cloud computing technology is constantly evolving, with new services and features continually emerging. As developers, we need to maintain a passion for learning and continually update our knowledge base. Do you have any thoughts to share? Feel free to discuss in the comments.
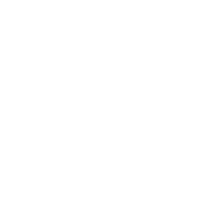
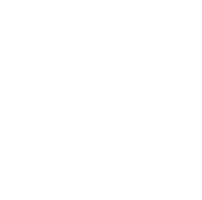