Have you ever thought about freeing your Python code from the confines of your local computer and letting it soar in the cloud? Today, we'll talk about the exciting applications of Python in cloud computing environments. As a Python blogger who loves to share, I can't wait to share with you my observations and insights in this field. Let's embark on this cloud journey together!
Cloud Selection
Choosing the right cloud service platform can be overwhelming. Don't worry, I'll break down a few popular choices for you.
AWS Lambda
Remember those scripts that only run at specific times? AWS Lambda is tailor-made for such scenarios. It uses a serverless architecture where you just upload your Python code, set up triggers, and let AWS handle the rest.
For example, suppose you have a data processing script that needs to run once a day. With AWS Lambda, you can do this:
- Create a new Lambda function
- Upload your Python script
- Set up a daily trigger
- Wait for the results
Isn't it simple? I was amazed by its convenience when I first used it. You can even set environment variables to make your code more flexible.
GitHub Actions
Speaking of automation, GitHub Actions is definitely a choice you shouldn't miss. I have a friend who uses it to run his Python web scraping project, and it works quite well.
His project is like this: - Use Selenium to scrape URLs from certain websites - Add collected events to Google Calendar - Run periodically and store results
Initially, he tried using PythonAnywhere, but the results weren't very stable. Later, he switched to GitHub Actions, and the problem was solved. If your project is closely integrated with GitHub, this is definitely an option worth considering.
PythonAnywhere
Although my friend's experience wasn't very smooth, PythonAnywhere is still a good choice, especially for Python developers who are just starting to explore cloud computing. It provides a complete Python environment where you can write and run Python code directly in your browser.
Personally, I think PythonAnywhere's biggest advantage is its relatively gentle learning curve. You don't need to understand too many cloud computing concepts to start using it. For some simple projects or learning experiments, it's a great starting point.
Cloud Deployment
Alright, we've chosen our cloud platform, now it's time to deploy our Python project to it. This process might be a bit complicated, but don't worry, I'll walk you through it step by step.
Flask Takes Off
Flask is a star framework in Python web development, and deploying it to the cloud is like giving it wings. The two platforms I use most often are Heroku and AWS Elastic Beanstalk.
Taking Heroku as an example, the steps to deploy a Flask application are roughly as follows:
- Create a requirements.txt file listing all dependencies
- Create a Procfile specifying how to run your application
- Initialize a Git repository and commit your code
- Create a new application on Heroku
- Add Heroku as a remote repository and push your code
web: gunicorn app:app
This process might look complicated, but trust me, once you're familiar with it, you'll find it quite intuitive. And when you succeed in deploying, seeing your application come to life on the internet, that sense of achievement is incomparable!
Environment Configuration
When deploying Python projects to the cloud, environment configuration is an often overlooked but crucial step. I once suffered a lot because I ignored the differences between local and cloud environments.
A good practice is to use virtual environments and dependency management tools, such as venv and pip. This ensures that your project can run normally in different environments.
python -m venv myenv
source myenv/bin/activate # On Unix or MacOS
myenv\Scripts\activate.bat # On Windows
pip install -r requirements.txt
Also, for sensitive information like database passwords or API keys, it's best to manage them using environment variables. Most cloud platforms provide the functionality to set environment variables, which can increase the security and portability of your application.
Cloud Development
After migrating Python projects to the cloud, the next challenge we face is how to efficiently develop and debug in this new environment. Cloud development has its unique challenges, but it also brings many opportunities. Let's explore together!
Debugging Tools
Debugging Python applications in a cloud environment can feel a bit daunting. After all, you can't directly set breakpoints or print debug information like you would locally. But don't worry, we have other weapons!
Logging is the core tool for cloud debugging. My personal favorite is Python's logging module, which is simple yet powerful. Take a look at this example:
import logging
logging.basicConfig(level=logging.DEBUG)
logger = logging.getLogger(__name__)
def some_function():
logger.debug("Entering some_function")
# Your code
logger.info("Function completed successfully")
some_function()
This code will output detailed log information, including function entry and exit. In a cloud environment, you can easily view these logs to help you locate problems.
Additionally, AWS CloudWatch is a powerful monitoring tool. It not only collects logs but can also set up alerts to notify you when anomalies occur. I remember once, it was because I set up CloudWatch alerts that I received a notification in the middle of the night and was able to fix a serious database connection issue in time. I've loved this tool ever since!
Data is King
Handling large datasets in a cloud environment is a common requirement. Python has many powerful tools and frameworks in this regard.
For data storage, AWS S3 is a great choice. It can store massive amounts of data and access is fast. You can use the boto3 library to operate S3 in Python:
import boto3
s3 = boto3.resource('s3')
bucket = s3.Bucket('my-bucket')
bucket.upload_file('local_file.txt', 'remote_file.txt')
bucket.download_file('remote_file.txt', 'local_file.txt')
For data processing, Apache Spark is a powerful distributed computing framework. With PySpark, you can easily process large-scale datasets in Python:
from pyspark.sql import SparkSession
spark = SparkSession.builder.appName("MyApp").getOrCreate()
df = spark.read.csv("s3://my-bucket/data.csv")
result = df.groupBy("column").count()
result.write.csv("s3://my-bucket/result.csv")
The first time I used Spark to process a 10GB dataset, I was shocked by its speed. A processing task that used to take several hours could now be completed in just a few minutes. This is the charm of distributed computing!
Cloud Optimization
Deploying Python applications to the cloud is not the end, but a new beginning. How to make your application run faster, more stable, and more secure in the cloud are all issues we need to consider.
Performance Improvement
In a cloud environment, resources are money. How to use these resources efficiently is a question every developer needs to think about.
First, reasonable resource allocation is very important. Most cloud platforms provide auto-scaling functionality, where you can automatically increase or decrease resources based on load. For example, using AWS Auto Scaling:
import boto3
client = boto3.client('autoscaling')
response = client.create_auto_scaling_group(
AutoScalingGroupName='my-asg',
LaunchConfigurationName='my-launch-config',
MinSize=1,
MaxSize=5,
DesiredCapacity=2,
# Other parameters...
)
This code creates an auto-scaling group that can automatically adjust the number of instances based on demand, ensuring your application always has enough resources to handle requests.
Secondly, code optimization is also key to improving performance. In Python, using generators instead of lists, utilizing data structures from the collections
module, avoiding global variables, etc., are all effective optimization techniques. Look at this example:
def process_large_file(filename):
with open(filename) as f:
return [line.strip().upper() for line in f if line.strip()]
def process_large_file(filename):
with open(filename) as f:
for line in f:
line = line.strip()
if line:
yield line.upper()
The optimized version uses a generator, which can greatly reduce memory usage, especially when processing large files.
Security Protection
In a cloud environment, security becomes particularly important. After all, your application and data are now exposed on the internet.
Data encryption is a basic means of protecting sensitive information. Python's cryptography
library provides powerful encryption functionality:
from cryptography.fernet import Fernet
key = Fernet.generate_key()
f = Fernet(key)
encrypted = f.encrypt(b"Secret message")
decrypted = f.decrypt(encrypted)
This code demonstrates how to use the Fernet symmetric encryption algorithm to encrypt and decrypt data. In practical applications, you need to securely store and manage keys.
Access control and authentication are also important components of security. For web applications, you can use libraries like Flask-Login to manage user authentication:
from flask_login import LoginManager, UserMixin, login_required
login_manager = LoginManager()
login_manager.init_app(app)
class User(UserMixin):
# User model
@login_manager.user_loader
def load_user(user_id):
return User.get(user_id)
@app.route('/protected')
@login_required
def protected():
return "This is a protected page!"
This example shows how to implement basic user authentication and access control in a Flask application.
Speaking of security, I recall an interesting experience. Once, I accidentally hard-coded a database password into my code and pushed it to a public repository. Fortunately, GitHub quickly discovered this issue and notified me. Since then, I've learned to use environment variables to manage sensitive information and pay more attention to code review. This lesson taught me that in a cloud environment, security awareness must always remain vigilant.
Conclusion
Our Python cloud journey ends here. We discussed how to choose cloud services, how to deploy Python applications, how to develop and debug in a cloud environment, and how to optimize the performance and security of cloud applications. These topics cover various aspects of Python's application in cloud computing.
Looking back on this journey, do you feel the infinite possibilities that cloud computing brings to Python development? From simple script automation to complex big data processing to highly available web applications, Python's range of applications in the cloud is so broad, and its potential is so great.
However, our learning journey is endless. Cloud computing technology is changing rapidly, and the Python ecosystem is constantly evolving. As developers, we need to maintain our enthusiasm for learning and continuously explore new technologies and methods.
Finally, I want to ask you: What interesting experiences or challenges have you encountered when using cloud computing? What potential application scenarios do you think Python has in cloud computing? Feel free to share your thoughts and experiences in the comments section. Let's discuss and grow together!
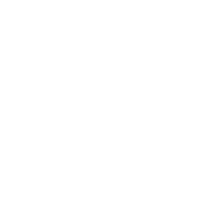
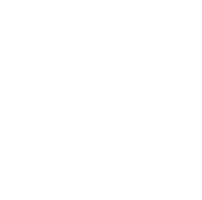
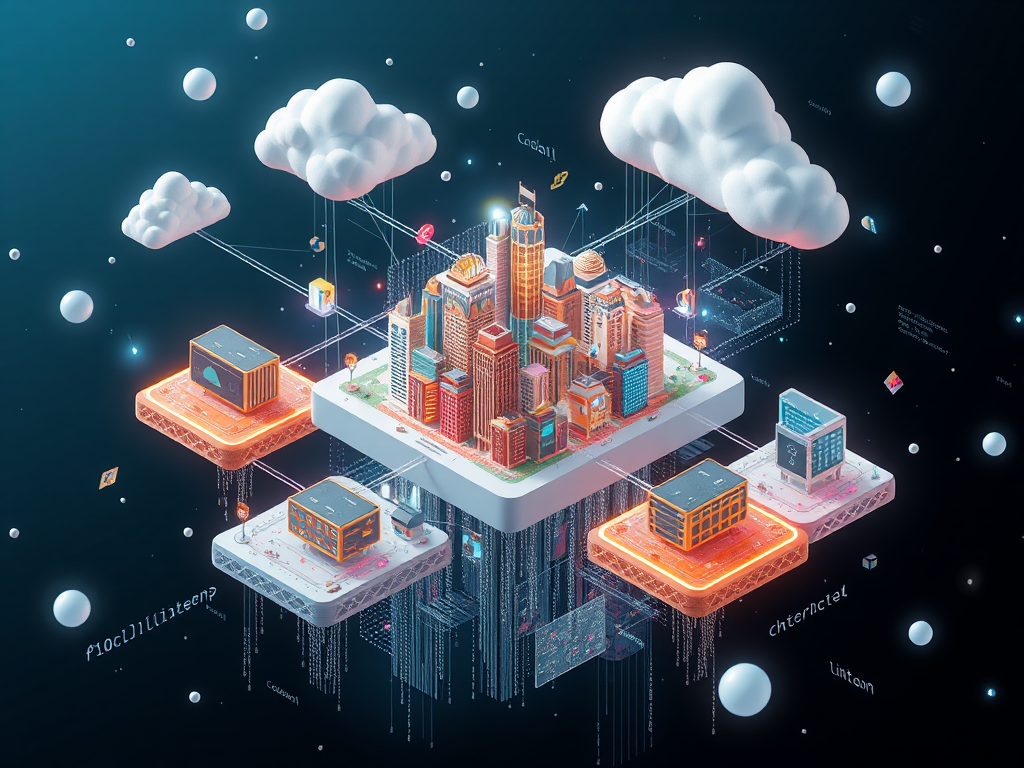
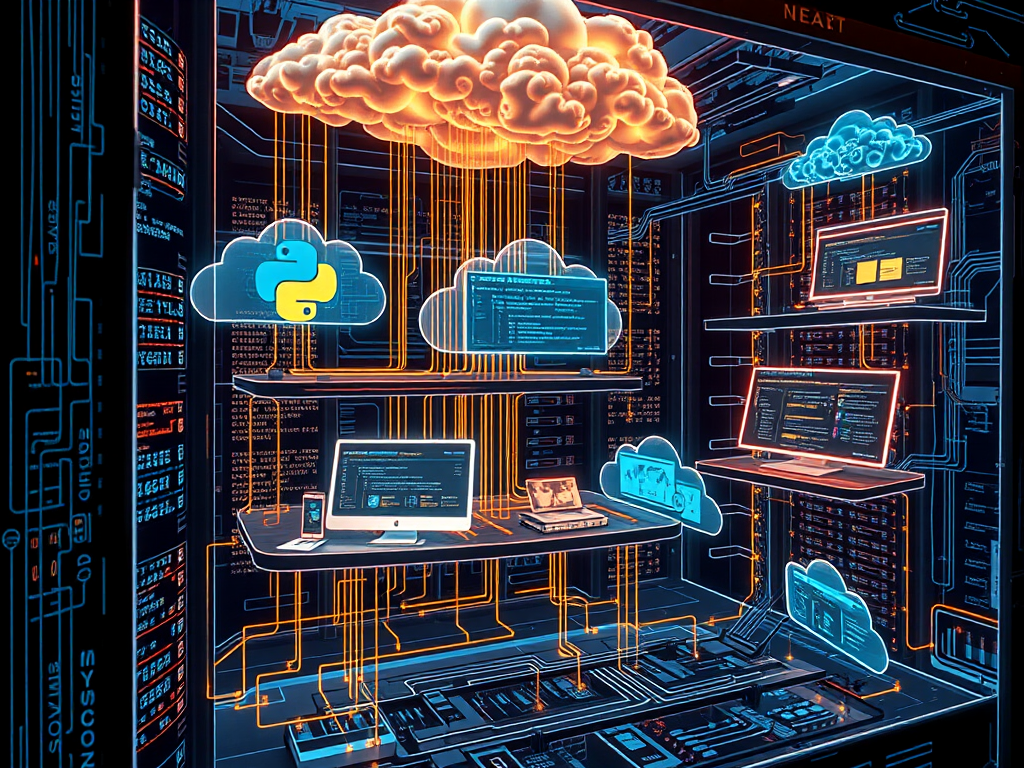
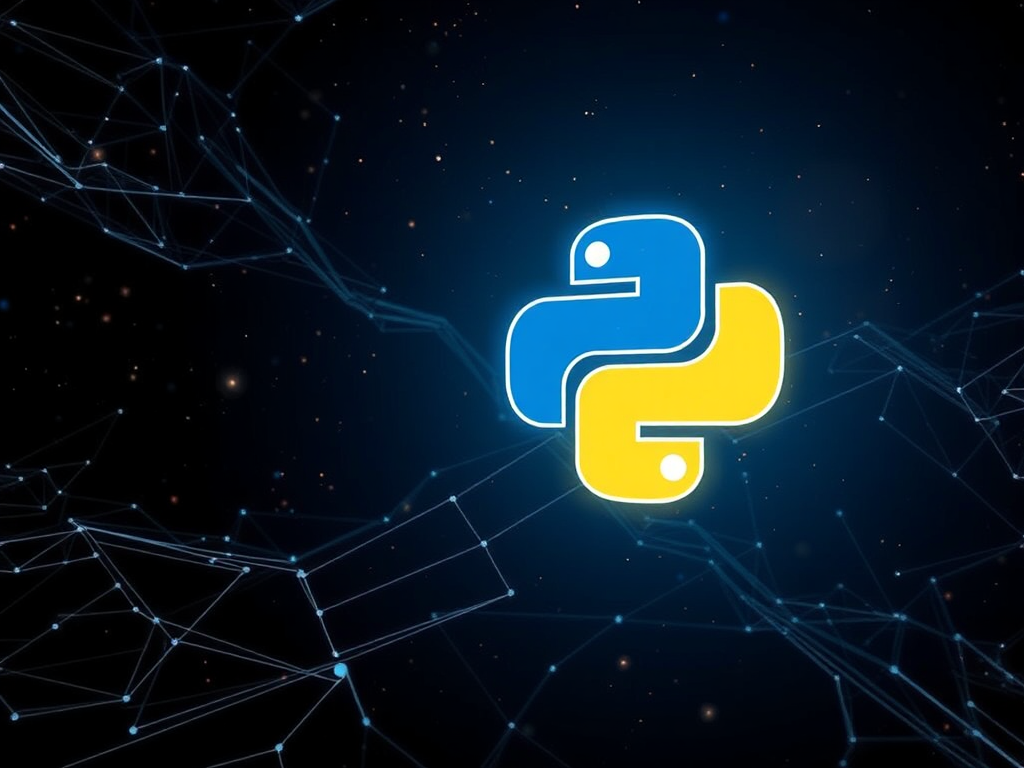