Have you ever encountered a situation where you wrote a complex Python program, but your local computer's performance was too slow, like a snail crawling? Or you wanted to perform large-scale data processing, but didn't have enough local hard drive space? Or perhaps you want your Python program to run 24/7 without interruption, but don't want to keep your computer on all the time?
If these are your concerns, then cloud Python programming is definitely your savior! Today, let's talk about how to run Python on cloud platforms, allowing your code to break free from local limitations and unleash unlimited potential.
Cloud Magic
First, let's understand why cloud Python programming is so powerful. Imagine suddenly having a supercomputer with unlimited computing power and storage space, accessible anytime, anywhere. This is the magic that cloud computing brings us!
In the cloud, you can easily obtain: 1. Powerful computing power: Run complex algorithms and models without upgrading local hardware. 2. Flexible resource allocation: Need more memory or CPU? Just a few clicks will do. 3. High availability: Your program can run 24/7 uninterrupted without worrying about local computer shutdowns. 4. Collaboration convenience: Team members can easily share and collaborate on the same codebase.
Sounds tempting, right? So, let's start exploring how to wield Python magic on major cloud platforms!
Google Cloud Platform
When it comes to cloud computing, how can we not mention Google Cloud Platform (GCP)? As a leader in the cloud computing world, GCP offers powerful tools for Python developers. Let's take a look at a few key players:
Data Processing Tool: Google Cloud Dataproc
If you need to process massive amounts of data, Google Cloud Dataproc is definitely your powerful assistant. It is a managed service based on Apache Spark and Apache Hadoop, allowing you to easily perform large-scale data processing.
Imagine having a task to analyze hundreds of GB of log files. Running locally might take days, but with Dataproc, you can complete it in hours! How is it done? The secret lies in its ability to automatically set up and manage distributed computing clusters for you.
Here's how to create a Dataproc cluster with Python code:
from google.cloud import dataproc_v1
cluster_client = dataproc_v1.ClusterControllerClient(client_options={"api_endpoint": "us-central1-dataproc.googleapis.com"})
cluster_config = {
"cluster_name": "my-python-cluster",
"config": {
"master_config": {
"num_instances": 1,
"machine_type_uri": "n1-standard-2",
},
"worker_config": {
"num_instances": 2,
"machine_type_uri": "n1-standard-2",
},
},
}
operation = cluster_client.create_cluster(
project_id="your-project-id",
region="us-central1",
cluster=cluster_config
)
result = operation.result()
print(f"Cluster created successfully: {result.cluster_name}")
See, with just a few lines of code, you've created a powerful distributed computing cluster! Isn't it amazing?
But be sure to delete the cluster when you're done to avoid unnecessary costs. You can delete the cluster like this:
delete_operation = cluster_client.delete_cluster(
project_id="your-project-id",
region="us-central1",
cluster_name="my-python-cluster"
)
delete_operation.result()
print("Cluster deleted successfully")
Lightweight Option: Google Cloud Functions
If Dataproc seems a bit overkill for you, then Google Cloud Functions might be more suitable. It's a "serverless" computing platform that lets you run single Python functions without managing entire servers.
Imagine having a simple Python function that processes user-uploaded images. With Cloud Functions, you can easily deploy this function to automatically trigger when a user uploads an image. Cool, right?
Here's how to deploy a simple Python function to Cloud Functions:
First, create a file named main.py
with the following content:
def hello_world(request):
return 'Hello, World!'
Then, use the following command to deploy the function:
gcloud functions deploy hello_world --runtime python39 --trigger-http --allow-unauthenticated
Just like that, your Python function is running in the cloud! Whenever an HTTP request arrives, this function will be triggered.
Versatile Option: Google Compute Engine
If you need more control and flexibility, Google Compute Engine (GCE) is your best choice. It's like a virtual machine in the cloud where you can install any software you want and run any type of Python program.
With GCE, you can easily create a virtual machine instance running Python. Here's an example:
gcloud compute instances create my-python-instance \
--zone=us-central1-a \
--machine-type=e2-medium \
--image-family=debian-10 \
--image-project=debian-cloud \
--metadata=startup-script='#! /bin/bash
sudo apt-get update
sudo apt-get install -y python3 python3-pip
pip3 install numpy pandas matplotlib'
This command creates a virtual machine named my-python-instance
and automatically installs Python3 and some common data science libraries. Isn't it convenient?
Amazon Web Services
After talking about Google Cloud, let's look at another cloud computing giant: Amazon Web Services (AWS). AWS also offers a wealth of tools and services for Python developers.
EC2: Your Cloud Host
AWS EC2 (Elastic Compute Cloud) is one of AWS's core services. It's like renting a computer in the cloud where you can do anything you want, including running Python programs.
The steps to run Python programs using EC2 are roughly as follows:
- Create an EC2 instance (can be done through the AWS console or AWS CLI)
- Connect to your EC2 instance
- Install Python (if not already installed)
- Run your Python code
Here's an example showing how to connect to an EC2 instance and run Python code:
ssh -i "your-key-pair.pem" ec2-user@your-instance-public-dns
sudo yum update -y
sudo yum install python3 -y
echo "print('Hello from AWS EC2!')" > hello.py
python3 hello.py
See, it's that simple—your Python code is running in the cloud!
Online IDE: Code Anytime, Anywhere
In addition to these powerful cloud platforms, there are also online IDEs that allow you to write and run Python code anytime, anywhere. These tools are especially useful for quickly testing ideas or performing simple programming exercises.
Repl.it: Easy-to-Use Online Python Environment
Repl.it is a very popular online IDE. With Repl.it, you can start writing and running Python code just by opening a browser. It even supports some common Python libraries like numpy and pandas.
Using Repl.it is very simple:
- Open Repl.it
- Click "+ New repl"
- Choose Python
- Start coding!
Google Colab: A Great Helper for Data Science
If you're interested in data science and machine learning, then Google Colab is definitely your friend. It not only provides an online Python environment but also pre-installs many commonly used data science libraries like numpy, pandas, and matplotlib. Plus, it offers free GPU resources, making it easy to train machine learning models.
Using Google Colab is also simple:
- Open Google Colab
- Create a new notebook
- Start coding!
In Colab, you can easily install other Python libraries:
!pip install some_library
Things to Consider with Cloud Python
While cloud Python programming brings many conveniences, there are some things to keep in mind:
-
Cost control: Using cloud services costs money, so be mindful of controlling costs. For example, remember to delete Dataproc clusters after use, and shut down unused EC2 instances promptly.
-
Security: Protect your sensitive data when running code in the cloud. Use appropriate security settings, such as setting firewall rules and using secure API keys.
-
Network latency: Although cloud services usually have good network connections, network latency issues can still arise. Consider this when designing your application.
-
Learning curve: Each cloud platform has its own features and usage methods, which may take some time to learn initially. But don't worry, once you master these skills, you'll greatly increase your productivity!
Conclusion
Cloud Python programming opens a new door for us, allowing us to break free from local environment limitations and achieve more interesting and challenging projects. Whether you want to process large-scale data, deploy a simple web service, or just write code anytime, anywhere, cloud platforms can meet your needs.
So, are you ready to start your cloud Python journey? Maybe you already have some ideas you want to implement in the cloud? Or are you particularly interested in a specific cloud service? Feel free to share your thoughts in the comments, and let's discuss how to unleash Python's unlimited potential in the cloud!
Remember, in the world of programming, the sky's the limit. And with cloud computing, even the sky is no longer the limit! Let's soar in the cloud and create more amazing Python projects together!
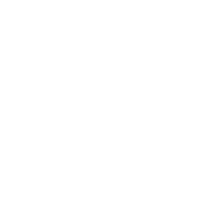
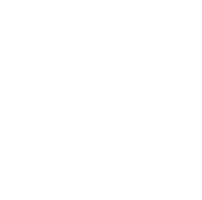