Hello dear readers!
I believe that, like me, you all have a strong interest and curiosity about Python Object-Oriented Programming (OOP). Today, let's discuss this classic topic and see if we can gain some new insights and ideas.
Without further ado, let's get right to the point!
Classes and Objects
The core of object-oriented programming is the concept of classes and objects. A class can be seen as a blueprint or template, while an object is an instance created based on this blueprint.
For example, we can define a Dog
class that includes some attributes and behaviors of dogs:
class Dog:
def __init__(self, name, breed):
self.name = name
self.breed = breed
def bark(self):
print(f"{self.name} says: Woof!")
With this Dog
class, we can create multiple different dog objects:
buddy = Dog("Buddy", "Golden Retriever")
rocky = Dog("Rocky", "Bulldog")
buddy.bark() # Buddy says: Woof!
rocky.bark() # Rocky says: Woof!
Isn't it amazing? We only need to define the Dog
class once, and we can create countless dog objects, each with its unique name and breed, but all sharing the common "bark" behavior.
Object Instantiation and Usage
The process of creating an object is called "instantiation". You can imagine it as pressing a "copy" button, creating a new object copy from the class template.
Each object has its own independent memory space, storing its own attribute values. But they share all the methods defined in the class.
This is why buddy
and rocky
, although different objects, can both call the bark()
method. Because this method is defined in the Dog
class, all dog objects can inherit and use it.
You clever ones must have already realized that by creating objects, we've turned the abstract concept of "dog" into concrete individuals, each with its own characteristics and behaviors. This ability to transform abstract concepts into concrete instances is exactly where the magic of object-oriented programming lies!
Core OOP Concepts
Alright, now that we understand the basics of classes and objects, let's delve deeper into the three core concepts of OOP: encapsulation, inheritance, and polymorphism.
Encapsulation
The core idea of encapsulation is to bundle data and operations on that data together, ensuring data integrity and validity.
Let's use the Dog
class as an example again. Suppose we don't want users to directly modify a dog's name, but instead provide a specific method to do this:
class Dog:
def __init__(self, name, breed):
self._name = name # Add an underscore before the attribute, suggesting it's an internal attribute
self.breed = breed
def bark(self):
print(f"{self._name} says: Woof!")
def rename(self, new_name):
# Here we can add some logic to check if the new name is valid
self._name = new_name
buddy = Dog("Buddy", "Golden Retriever")
buddy.bark() # Buddy says: Woof!
buddy._name = "Bud" # This is just a convention, it won't actually prevent you from doing this
buddy.bark() # Buddy says: Woof! The name hasn't changed
buddy.rename("Bud")
buddy.bark() # Bud says: Woof!
Through encapsulation, we've set the _name
attribute as a protected internal attribute, not allowing direct external access and modification. All operations on name
must go through the rename()
method, thus ensuring data integrity.
This practice of combining data and behavior into a single unit not only enhances code security but also improves code reusability and maintainability. This is the essence of encapsulation.
Inheritance
Inheritance is a very powerful concept in object-oriented programming. It allows us to create new classes based on existing classes, automatically inheriting the attributes and methods of the existing classes.
This way, we can reuse the code of existing classes, greatly saving development time. At the same time, derived classes can also override or extend the functionality of the base class as needed.
Let's look at an example:
class Animal:
def __init__(self, name):
self.name = name
def greet(self):
print(f"Hello, I am {self.name}")
class Dog(Animal):
def __init__(self, name, breed):
super().__init__(name) # Call the base class constructor
self.breed = breed
def bark(self):
print(f"{self.name} says: Woof!")
buddy = Dog("Buddy", "Golden Retriever")
buddy.greet() # Hello, I am Buddy
buddy.bark() # Buddy says: Woof!
In this example, the Dog
class inherits from the Animal
class. It automatically gets the name
attribute and greet()
method, while also adding its own breed
attribute and bark()
method.
This way, when creating the Dog
class, we can reuse the existing functionality of the Animal
class without having to write everything from scratch. At the same time, the Dog
class can customize and extend functionality as needed.
The concept of inheritance not only demonstrates code reusability but also aligns well with real-world inheritance relationships. A dog is certainly a type of animal, so it naturally possesses some common characteristics of animals.
Polymorphism
The concept of polymorphism is a bit harder to understand, but it's a very powerful feature in OOP.
Polymorphism means that the same behavior or method can manifest in completely different forms on different objects.
Let's use the above example and add a new Cat
class:
class Cat(Animal):
def __init__(self, name):
super().__init__(name)
def make_sound(self):
print(f"{self.name} says: Meow!")
animals = [Dog("Buddy", "Golden Retriever"), Cat("Kitty")]
for animal in animals:
animal.greet() # Call the greet() method
animal.make_sound() # Call the make_sound() method
Output result:
Hello, I am Buddy
Buddy says: Woof!
Hello, I am Kitty
Kitty says: Meow!
You see, when we call the make_sound()
method, the dog object will say "Woof", while the cat object will say "Meow". Responding differently to the same method is the manifestation of polymorphism.
The magic of polymorphism lies in that we can handle different objects in a unified way. In the code above, we put dog and cat objects in the same list for iteration, without needing to care about their specific types. As long as they all implement the greet()
and make_sound()
methods, we can call these methods on them indiscriminately.
This idea of generic programming is very powerful, making our code more flexible, extensible, and closer to the way the real world works.
Advanced Python OOP Applications
After introducing the three pillars of OOP, let's look at some more advanced applications, such as design patterns and some special Python syntax.
Design Patterns
Design patterns are general solutions to common problems in software design. They are best practices of code that have been summarized through practice.
Mastering design patterns not only improves the readability and maintainability of code but also allows you to excel in interviews. As an excellent Pythonista, it's natural to be proficient in design patterns.
Here, let's briefly introduce two common design patterns.
Singleton Pattern
The Singleton pattern ensures that a class has only one instance and provides a global access point to it. This is useful in many scenarios, such as logging, configuration management, etc.
Implementing the Singleton pattern in Python is very simple:
class Singleton(object):
_instance = None
def __new__(cls, *args, **kwargs):
if not cls._instance:
cls._instance = super(Singleton, cls).__new__(cls)
return cls._instance
Here, we've overwritten the __new__
method. Each time a new object is created, it first checks if _instance
already exists. If it exists, it directly returns that unique instance; if not, it creates a new instance.
Simple, right? The Singleton pattern ensures that no matter how many places are trying to create instances of this class, only one object is actually created.
Factory Pattern
The Factory pattern is a best practice for creating objects. It defines an interface for creating objects, but lets subclasses decide which class to instantiate.
This approach decouples the object instantiation logic from the code that uses the object, increasing the flexibility and extensibility of the code.
class Animal:
def make_sound(self):
pass
class Dog(Animal):
def make_sound(self):
print("Woof!")
class Cat(Animal):
def make_sound(self):
print("Meow!")
class AnimalFactory:
def create_animal(self, animal_type):
if animal_type == "Dog":
return Dog()
elif animal_type == "Cat":
return Cat()
else:
return Animal()
factory = AnimalFactory()
dog = factory.create_animal("Dog")
cat = factory.create_animal("Cat")
dog.make_sound() # Woof!
cat.make_sound() # Meow!
In this example, we defined an AnimalFactory
class that returns the corresponding Animal
subclass instance based on different animal_type
parameters.
A major benefit of using the Factory pattern is that if we need to add new animal classes in the future, we only need to modify the factory class without changing the calling code. This greatly improves the maintainability and flexibility of the code.
Special Methods and Magic Methods
Python has many special methods that start and end with double underscores. These methods provide some special syntactic behaviors for our class objects.
These methods are often called "magic methods" because they allow our objects to behave like Python's built-in data types, supporting some special syntactic operations.
__init__
and Constructor
The __init__
method is the most common magic method. It's automatically called when creating a new instance of the class and is commonly used as the class constructor.
We've used the __init__
method in our previous examples. It allows us to specify initial attribute values when creating objects.
In addition to the __init__
method, Python also has a __new__
method that is automatically called when creating an instance, even earlier than __init__
. Usually, we don't need to define __new__
ourselves unless we want to control how new instances are created, such as implementing the Singleton pattern.
Operator Overloading
Python's magic methods also allow us to overload common operators, enabling our class objects to support arithmetic operations.
For example, we want to overload the addition operator for the Vector
class:
class Vector:
def __init__(self, x, y):
self.x = x
self.y = y
def __add__(self, other):
return Vector(self.x + other.x, self.y + other.y)
v1 = Vector(1, 2)
v2 = Vector(3, 4)
v3 = v1 + v2
print(v3.x, v3.y) # Output: 4 6
By defining the __add__
method, we can now add two Vector
instances together. Python has a large number of magic methods that can overload different operators. You can view the detailed list in the Python documentation.
The advantage of using magic methods is that we can make our custom class objects possess the behavioral characteristics of built-in types, making the code more concise and readable.
Summary
Alright, that's all for today. We've learned about the basics of Python Object-Oriented Programming, core concepts, and some advanced applications.
Object-oriented programming is not just a programming paradigm, but also a way of understanding and modeling the real world. By abstracting the concepts of classes and objects, we simplify complex things, thereby better managing and manipulating them.
The three core concepts of OOP - encapsulation, inheritance, and polymorphism - provide us with advantages such as code reuse, extensibility, and flexibility, which are powerful tools for building robust, high-quality software.
In addition, advanced features in Python such as design patterns and magic methods further maximize the powerful functionality of OOP.
Learning programming is not something that can be achieved overnight; it requires constant practice and reflection. I hope that through today's sharing, you've gained a deeper understanding of Python's object-oriented programming. Of course, this is just the beginning, and there's still a long road ahead. Let's bravely move forward on this path together!
Remember, coding is not just a skill, but also a unique way of thinking. Keep your curiosity, practice more, and you will definitely become an excellent Pythonista! Looking forward to seeing you in the next sharing session!
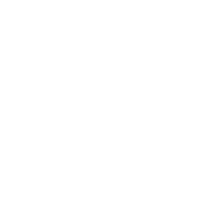
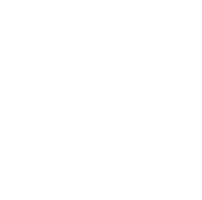