Have you ever wondered why you can directly print an object, or why you can use the len()
function to get the length of an object? Behind these seemingly magical features are Python's magic methods at work. Today, let's delve into Python's magic methods and see how they make our classes more Pythonic!
What Are Magic Methods?
Magic methods, as the name suggests, are special methods that seem a bit magical. In Python, their names start and end with double underscores, like __init__
, __str__
, etc. These methods allow us to customize the behavior of classes, enabling them to work like built-in types.
You might wonder, why such strange naming? It's actually a Python convention to avoid conflicts with user-defined method names. Moreover, this unique naming makes these methods stand out in the code, easily recognizable at a glance.
Common Magic Methods
Construction and Initialization
First, let's look at the most commonly used magic method __init__
:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
p = Person("Alice", 25)
print(p.name, p.age) # Output: Alice 25
The __init__
method is automatically called after an object is created, used to initialize the object's properties. But did you know? Before __init__
, there's a __new__
method that gets called:
class Person:
def __new__(cls, *args, **kwargs):
print("Creating a new Person")
return super().__new__(cls)
def __init__(self, name, age):
print("Initializing the Person")
self.name = name
self.age = age
p = Person("Bob", 30)
The __new__
method is responsible for creating and returning an instance, while __init__
initializes it. In most cases, we don't need to override __new__
, but in certain special cases (like implementing a singleton pattern), it can be very useful.
String Representation
Next, let's look at __str__
and __repr__
, the two magic methods for string representation:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def __str__(self):
return f"{self.name}, {self.age} years old"
def __repr__(self):
return f"Person(name='{self.name}', age={self.age})"
p = Person("Charlie", 35)
print(str(p)) # Output: Charlie, 35 years old
print(repr(p)) # Output: Person(name='Charlie', age=35)
The __str__
method returns the string representation of an object, mainly for users, and should return a concise and readable string. The __repr__
method returns the "official" string representation of an object, mainly for developers, usually containing more detailed information.
A tip: If you define only the __repr__
method without __str__
, Python will use the __repr__
result for string representation. So, if you only want to define one method, __repr__
might be the better choice.
Comparison Operations
Python provides a series of magic methods to customize comparison operations:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def __eq__(self, other):
if isinstance(other, Person):
return self.age == other.age
return False
def __lt__(self, other):
if isinstance(other, Person):
return self.age < other.age
return NotImplemented
p1 = Person("David", 40)
p2 = Person("Eve", 40)
p3 = Person("Frank", 45)
print(p1 == p2) # Output: True
print(p1 < p3) # Output: True
print(p1 > p3) # Output: False
In this example, we defined __eq__
(equal) and __lt__
(less than) methods. Interestingly, by defining these two methods, we automatically gain the capability for other comparison operations. For example, the >
operation will automatically become the reverse of <
.
Numeric Operations
If you want your class to support mathematical operations, you can use the related magic methods:
class Vector:
def __init__(self, x, y):
self.x = x
self.y = y
def __add__(self, other):
return Vector(self.x + other.x, self.y + other.y)
def __mul__(self, scalar):
return Vector(self.x * scalar, self.y * scalar)
def __str__(self):
return f"Vector({self.x}, {self.y})"
v1 = Vector(1, 2)
v2 = Vector(3, 4)
print(v1 + v2) # Output: Vector(4, 6)
print(v1 * 2) # Output: Vector(2, 4)
In this example, we defined the __add__
method to support vector addition and the __mul__
method to support vector-scalar multiplication. Note that the __mul__
method only supports left-to-right multiplication (like v1 * 2
). If you also want to support right-to-left multiplication (like 2 * v1
), you need to define the __rmul__
method.
Container Class Methods
If you want your class to behave like a container (such as a list or dictionary), you can implement the following methods:
class MyList:
def __init__(self, data):
self.data = data
def __len__(self):
return len(self.data)
def __getitem__(self, index):
return self.data[index]
def __setitem__(self, index, value):
self.data[index] = value
def __iter__(self):
return iter(self.data)
my_list = MyList([1, 2, 3, 4, 5])
print(len(my_list)) # Output: 5
print(my_list[2]) # Output: 3
my_list[2] = 10
print(my_list[2]) # Output: 10
for item in my_list:
print(item, end=' ') # Output: 1 2 10 4 5
In this example, we implemented the __len__
, __getitem__
, __setitem__
, and __iter__
methods, making the MyList
class behave much like a list.
Practical Applications
Magic methods have a wide range of applications. For example, in data science, we often need to handle large amounts of data. Let's say we have a class representing a dataset:
import numpy as np
class Dataset:
def __init__(self, data):
self.data = np.array(data)
def __len__(self):
return len(self.data)
def __getitem__(self, index):
return self.data[index]
def __add__(self, other):
if isinstance(other, Dataset):
return Dataset(np.concatenate([self.data, other.data]))
elif isinstance(other, (int, float)):
return Dataset(self.data + other)
else:
return NotImplemented
def __str__(self):
return f"Dataset with {len(self)} samples"
def __repr__(self):
return f"Dataset({repr(self.data.tolist())})"
d1 = Dataset([1, 2, 3])
d2 = Dataset([4, 5, 6])
print(len(d1)) # Output: 3
print(d1[1]) # Output: 2
print(d1 + d2) # Output: Dataset with 6 samples
print(d1 + 10) # Output: Dataset with 3 samples
print(repr(d1 + 10)) # Output: Dataset([11, 12, 13])
In this example, we defined a Dataset
class that can:
1. Get the size of the dataset using the __len__
method
2. Support index access using the __getitem__
method
3. Support dataset concatenation and numeric addition using the __add__
method
4. Provide friendly string representation using the __str__
and __repr__
methods
This design makes the Dataset
class very intuitive and convenient to use, just like operating on Python's built-in types.
Considerations
While magic methods are powerful, there are some issues to note when using them:
-
Don't overuse magic methods. Use them only when truly necessary. Overuse can make the code difficult to understand and maintain.
-
The performance of magic methods may not be as good as regular methods. For example, the
__getattribute__
method is called every time an attribute is accessed, and if it performs complex operations, it might affect performance. -
Some magic methods (such as
__new__
,__init__
,__del__
, etc.) should be used with particular caution, as they may affect the object's lifecycle. -
Remember Python's design philosophy: "Explicit is better than implicit." Although magic methods can make some operations seem magical, you should prioritize more intuitive designs whenever possible.
Summary
Magic methods are a powerful tool in Python's object-oriented programming. By implementing these methods, we can make custom classes behave more like Python's built-in types, allowing us to write more Pythonic code.
In actual programming, you may not need to use all these magic methods frequently. However, knowing their existence and function is valuable. When you encounter a situation that requires customizing class behavior, you'll know which magic method to use.
Finally, learning and using magic methods can deepen your understanding of how Python works. They are like the "unsung heroes" of Python's object-oriented system, quietly supporting many features we take for granted. So, next time you encounter some seemingly magical operations in Python, think about whether there's a magic method at play behind the scenes.
Do you have any thoughts or experiences with Python's magic methods? Feel free to share your ideas in the comments!
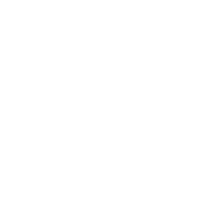
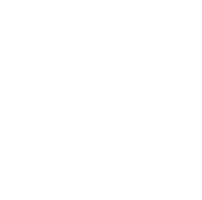