Have you ever wondered why some Python methods are prefixed with decorators like @classmethod
or @staticmethod
? What sets these methods apart from regular instance methods? Today, let's explore this intriguing topic!
Types of Methods
In Python, methods within a class are primarily divided into three types: instance methods, class methods, and static methods. Each has its characteristics and is suitable for different scenarios. Let's look at each:
Instance Methods
Instance methods are the most common type of methods. Their first parameter is usually named self
, representing the instance of the class. You can access instance attributes and other methods through self
.
class Student:
def __init__(self, name, age):
self.name = name
self.age = age
def introduce(self):
return f"My name is {self.name}, and I am {self.age} years old."
In this example, introduce
is an instance method. We need to create an instance of Student
before calling this method:
student = Student("Xiao Ming", 18)
print(student.introduce()) # Output: My name is Xiao Ming, and I am 18 years old.
Class Methods
Class methods are defined using the @classmethod
decorator. Their first parameter is usually named cls
, representing the class itself. Class methods can access and modify class state, which is particularly useful for implementing alternative constructors or managing class-level data.
class Student:
count = 0
def __init__(self, name, age):
self.name = name
self.age = age
Student.count += 1
@classmethod
def from_birth_year(cls, name, birth_year):
return cls(name, 2023 - birth_year)
@classmethod
def get_count(cls):
return cls.count
In this example, from_birth_year
and get_count
are class methods. We can call these methods directly through the class:
student1 = Student.from_birth_year("Xiao Hong", 2000)
print(student1.age) # Output: 23
student2 = Student("Xiao Ming", 18)
print(Student.get_count()) # Output: 2
See how convenient it is? We can use the from_birth_year
method to create a Student
instance without manually calculating the age. Meanwhile, the get_count
method lets us know how many Student
instances have been created.
Static Methods
Static methods are defined using the @staticmethod
decorator. They do not require self
or cls
as parameters, meaning they cannot access instance attributes or class attributes. So, what's their use? Static methods are typically used to implement some functionality related to the class but do not need to access the class or instance state.
class MathOperations:
@staticmethod
def add(x, y):
return x + y
@staticmethod
def multiply(x, y):
return x * y
We can call static methods directly through the class without creating an instance:
print(MathOperations.add(3, 5)) # Output: 8
print(MathOperations.multiply(3, 5)) # Output: 15
How to Choose?
When should we use these three types of methods? Here are some suggestions:
- Use instance methods if your method needs to access or modify the instance's state.
- Use class methods if your method needs to access or modify the class's state, or if you want to create an alternative constructor.
- Use static methods if your method does not need to access the instance or class state but logically belongs to the class.
Practical Application
Let's look at a more complex example that uses all three types of methods:
class BookStore:
discount = 0.1 # Class attribute representing the discount
def __init__(self, name, author, price):
self.name = name
self.author = author
self.price = price
def get_price(self):
return self.price * (1 - self.discount)
@classmethod
def set_discount(cls, discount):
cls.discount = discount
@staticmethod
def is_expensive(price):
return price > 100
book = BookStore("Python Programming", "Zhang San", 80)
print(f"The original price of the book is {book.price} yuan, the discounted price is {book.get_price():.2f} yuan")
BookStore.set_discount(0.2) # Modify the discount
print(f"The new discounted price is {book.get_price():.2f} yuan")
print(f"The book is {'expensive' if BookStore.is_expensive(book.price) else 'not expensive'}")
In this example:
get_price
is an instance method that uses the instance'sprice
attribute and the class'sdiscount
attribute to calculate the discounted price.set_discount
is a class method that can modify the class'sdiscount
attribute.is_expensive
is a static method that determines whether a given price is high.
By wisely using these three types of methods, we can make our code clearer and more flexible!
Summary
Class and static methods offer us more programming options, allowing us to better organize and manage our code. Remember, the choice of method type depends on your specific needs. Don't be afraid to try; practice more, and you'll discover their respective advantages!
Are you ready to apply this knowledge in your next project? I suggest trying to refactor some of your old code to see if you can improve it with class or static methods. Trust me, this process will give you a deeper understanding of Python's object-oriented programming!
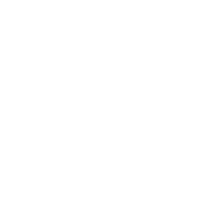
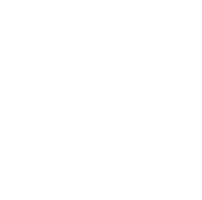