Origins
Have you ever wondered why Python is called the most beginner-friendly programming language, yet can power massive applications like YouTube and Instagram? This mystery largely comes down to Python's elegant object-oriented programming features.
As a developer who has written Python code for over ten years, I deeply understand the power of object-oriented programming. Let's explore this fascinating programming paradigm and see how it helps us write more elegant and maintainable code.
Understanding
Before diving deep, I want to share a perspective: object-oriented programming isn't just a programming method, but a way of thinking. It teaches us how to abstract real-world things into classes and objects in code.
Here's a simple example. Imagine you're developing a library management system. In traditional procedural programming, you might organize code like this:
book_titles = []
book_authors = []
book_isbns = []
def add_book(title, author, isbn):
book_titles.append(title)
book_authors.append(author)
book_isbns.append(isbn)
def find_book(isbn):
for i, book_isbn in enumerate(book_isbns):
if book_isbn == isbn:
return book_titles[i], book_authors[i]
return None
But in object-oriented programming, we think differently: In the real world, there's a concept of "book" with properties like title, author, ISBN, and actions like being borrowed and returned. So, we can create a Book class to correspond to this real concept:
class Book:
def __init__(self, title, author, isbn):
self.title = title
self.author = author
self.isbn = isbn
self.is_borrowed = False
def borrow(self):
if not self.is_borrowed:
self.is_borrowed = True
return True
return False
def return_book(self):
self.is_borrowed = False
See how this code better matches our thinking patterns?
Deep Dive
Let's explore the four pillars of object-oriented programming: encapsulation, inheritance, polymorphism, and abstraction. These concepts might sound abstract, but through practical examples, you'll see they all stem from real-world principles.
Encapsulation
Encapsulation is like putting protective clothing on data, only allowing access and modification through specific methods. Here's an example:
class BankAccount:
def __init__(self, owner_name, initial_balance):
self._owner_name = owner_name
self._balance = initial_balance
self._transaction_history = []
def deposit(self, amount):
if amount > 0:
self._balance += amount
self._transaction_history.append(f"Deposit: {amount}")
return True
return False
def withdraw(self, amount):
if 0 < amount <= self._balance:
self._balance -= amount
self._transaction_history.append(f"Withdrawal: {amount}")
return True
return False
def get_balance(self):
return self._balance
def get_transaction_history(self):
return self._transaction_history.copy()
In this example, the account balance _balance is private and can't be modified directly from outside - it must be accessed through deposit() and withdraw() methods. This ensures data safety and consistency. This design principle is very important in actual development, and I often use it to prevent accidental data modification.
Inheritance
Inheritance allows us to create new classes based on existing ones, which greatly reduces code duplication in actual development. For example, we can create a special savings account based on the BankAccount class:
class SavingsAccount(BankAccount):
def __init__(self, owner_name, initial_balance, interest_rate):
super().__init__(owner_name, initial_balance)
self._interest_rate = interest_rate
def add_interest(self):
interest = self._balance * self._interest_rate
self.deposit(interest)
self._transaction_history.append(f"Interest: {interest}")
This example shows the power of inheritance: SavingsAccount inherits all functionality from BankAccount while adding its own interest calculation feature.
Polymorphism
Polymorphism is one of the most interesting features in object-oriented programming. It allows different classes to have different implementations of the same method. Let's look at a practical example:
class Shape:
def area(self):
raise NotImplementedError("Subclasses must implement this method")
class Circle(Shape):
def __init__(self, radius):
self.radius = radius
def area(self):
return 3.14 * self.radius * self.radius
class Rectangle(Shape):
def __init__(self, width, height):
self.width = width
self.height = height
def area(self):
return self.width * self.height
def calculate_total_area(shapes):
return sum(shape.area() for shape in shapes)
shapes = [Circle(5), Rectangle(4, 6), Circle(3)]
total_area = calculate_total_area(shapes)
Notice how the calculate_total_area function doesn't need to know what specific shape it's dealing with - it works with any Shape subclass. That's the power of polymorphism: same interface, different implementations.
Practice
Now that we've covered the theory, let's look at a more complex practical application: a simple game system. This example will use all the concepts we've learned:
class GameCharacter:
def __init__(self, name, health, attack_power):
self._name = name
self._health = health
self._max_health = health
self._attack_power = attack_power
self._is_alive = True
def take_damage(self, damage):
if self._is_alive:
self._health = max(0, self._health - damage)
if self._health == 0:
self._is_alive = False
return f"{self._name} has been defeated!"
return f"{self._name} took {damage} damage, remaining health {self._health}"
def heal(self, amount):
if self._is_alive:
self._health = min(self._max_health, self._health + amount)
return f"{self._name} recovered {amount} health, current health {self._health}"
return f"{self._name} can no longer recover health"
def attack(self, target):
if self._is_alive:
return target.take_damage(self._attack_power)
return f"{self._name} can no longer attack"
class Warrior(GameCharacter):
def __init__(self, name):
super().__init__(name, health=100, attack_power=15)
self._shield = 20
def take_damage(self, damage):
reduced_damage = max(0, damage - self._shield)
return super().take_damage(reduced_damage)
class Mage(GameCharacter):
def __init__(self, name):
super().__init__(name, health=70, attack_power=20)
self._mana = 100
def cast_spell(self, target):
if self._mana >= 20:
self._mana -= 20
return target.take_damage(self._attack_power * 1.5)
return f"{self._name} doesn't have enough mana to cast spells"
This game system demonstrates all features of object-oriented programming:
- Encapsulation: Health, attack power, and other attributes are private and can only be modified through methods
- Inheritance: Warrior and Mage both inherit from GameCharacter
- Polymorphism: Warrior and Mage each have their own implementation of take_damage
- Abstraction: GameCharacter defines basic game character behaviors
Reflection
Object-oriented programming brings us many benefits:
- Clearer code organization: Each class has its clear responsibilities
- Easier code reuse: Inheritance helps avoid rewriting similar code
- Easier maintenance: Encapsulation ensures data safety and makes bugs easier to locate
- Better extensibility: Through inheritance and polymorphism, we can easily add new features
But be careful not to overuse object-oriented features. Sometimes, simple functions might be a better choice. As I often say: Programming paradigms are tools, not dogmas.
Insights
My biggest realization while learning object-oriented programming is: good code should flow naturally like storytelling. When designing classes, try asking yourself: What story is this class telling? Do its methods make intuitive sense?
Finally, here's a thought exercise: If you were to add a new character type "Rogue" to the game system above, how would you design its special abilities?
Remember, learning programming is a gradual process. The concepts we learned today might be hard to fully digest at once, but as you keep applying them in practice, they'll become part of your programming mindset.
Let's explore the world of object-oriented programming together and create more elegant code!
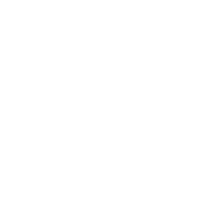
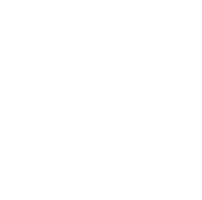