Introduction
Hello, Python enthusiasts! Today I'm bringing you a blog post about Python Object-Oriented Programming (OOP). I believe OOP is not unfamiliar to most Python developers, as it's a programming paradigm with enormous influence. But have you truly grasped the essence and practical details of OOP? Let's explore together!
What is Object-Oriented
Before we formally explain OOP, let's look at a simple example. Suppose we're developing a simple game with two types of characters: Players and Enemies, both having attributes like health and attack power. You might implement it like this:
player_health = 100
player_attack = 10
enemy_health = 80
enemy_attack = 15
At first glance, there doesn't seem to be a problem; the code implements the requirements. But if the game becomes more complex, with multiple players and enemies, and each character has more attributes and methods, this "procedural" coding approach will become increasingly chaotic and difficult to maintain.
This is where OOP shines. OOP bundles data (attributes) and behavior (methods) into an object. Different objects are independent, with cohesive data structures and clear interfaces, greatly improving code readability and maintainability.
Classes and Objects
The core concepts of OOP are "Class" and "Object". A class is an abstract concept used to define a "template" or "blueprint" for objects. An object is a concrete instance created based on a class, encapsulating data and code internally. Let's feel it through code:
class Player:
def __init__(self, name, health, attack):
self.name = name
self.health = health
self.attack = attack
def get_stats(self):
print(f'{self.name} has health {self.health} and attack power {self.attack}')
player1 = Player('Hero', 100, 20)
player2 = Player('Swordsman', 80, 25)
player1.get_stats() # Output: Hero has health 100 and attack power 20
player2.get_stats() # Output: Swordsman has health 80 and attack power 25
We defined a Player
class with three attributes: name
, health
, and attack
, and a get_stats
method. Using the Player
class, we created two specific object instances player1
and player2
.
See? Doesn't the code become clearer and more organized? Each object encapsulates its own data and behavior internally, and when accessed from the outside, you only need to call the object's public methods without worrying about the internal implementation details.
Encapsulation
The above example also demonstrates the "encapsulation" feature of OOP. Encapsulation means organically encapsulating the attributes and methods of an object, providing strict access control to internal data.
class BankAccount:
def __init__(self, balance=0):
self.balance = balance
def deposit(self, amount):
self.balance += amount
def withdraw(self, amount):
if self.balance >= amount:
self.balance -= amount
else:
print('Insufficient balance!')
account = BankAccount(1000)
account.deposit(500) # Balance becomes 1500
account.withdraw(2000) # Outputs "Insufficient balance!"
In this example, the balance
attribute of the BankAccount
class is set as private and cannot be directly accessed from the outside. The account balance can only be modified through the two public methods deposit
and withdraw
, and the withdraw
method adds a balance check to ensure no overdraft. This ensures the integrity of the data.
Inheritance
Inheritance is another important feature of OOP. It allows one class (subclass) to inherit attributes and methods from another class (parent class), achieving code reuse. This is useful in many scenarios, such as when you need to create different types of game characters:
class Character:
def __init__(self, name, health, attack):
self.name = name
self.health = health
self.attack = attack
def get_stats(self):
print(f'{self.name} has health {self.health} and attack power {self.attack}')
class Player(Character):
def __init__(self, name, health, attack, race):
super().__init__(name, health, attack)
self.race = race
def get_race(self):
print(f'{self.name} is a {self.race} race')
player = Player('Hero', 100, 20, 'Human')
player.get_stats() # Hero has health 100 and attack power 20
player.get_race() # Hero is a Human race
In the code above, the Player
class inherits from the Character
class, automatically acquiring attributes like name
, health
, attack
, and the get_stats
method. At the same time, it adds its own race
attribute and get_race
method. Code reuse greatly reduces repetitive work.
Polymorphism
Polymorphism is the third major feature of OOP. Polymorphism means that the same operation acting on different objects can produce different behaviors and results. This is achieved through method overriding and abstract classes/interfaces.
class Animal:
def make_sound(self):
pass
class Dog(Animal):
def make_sound(self):
print('Woof woof!')
class Cat(Animal):
def make_sound(self):
print('Meow meow!')
animals = [Dog(), Cat()]
for animal in animals:
animal.make_sound()
In this example, both Dog
and Cat
inherit from the Animal
class, but they override the make_sound
method to output different animal sounds. When we loop through each element in the animals
list and access the make_sound
method, due to polymorphism, the program automatically calls the overridden method version for each.
Design Patterns
Design patterns are summaries of common code structures and programming ideas in software design, which are very helpful in improving code reusability, extensibility, and maintainability. In Python, common design patterns include:
- Singleton Pattern: Ensures a class has only one instance and provides a global access point. This is useful in scenarios like thread pools, caches, log objects, etc.
- Factory Pattern: Creates instances of other classes through a factory class, decoupling object creation and use.
- Observer Pattern: Defines a one-to-many dependency between objects so that when one object changes state, all its dependents are notified and updated automatically.
Although design patterns may seem abstract in concept, they are ubiquitous in actual coding. Understanding and mastering them helps write more elegant and robust code.
Code Refactoring
Speaking of OOP, how can we not mention code refactoring? As requirements change and code iterates constantly, the codebase will inevitably become messy and chaotic one day. This is when code refactoring is needed to improve code readability, maintainability, and extensibility. Common refactoring techniques include:
- Extracting Superclass: Extract repeated attributes and methods into a parent class, and subclasses inherit to reuse code.
- Using Composition Instead of Inheritance: Sometimes when inheritance relationships become too complex, composition can be used to delegate some functionality to other objects.
- Refactoring Conditional Logic: Extract and encapsulate scattered conditional logic into independent functions or modules.
In addition, there are many other refactoring techniques, such as extracting methods, inlining code, removing dead code, etc. Mastering and appropriately applying these techniques can greatly improve code quality.
OOP and Other Paradigms
Ultimately, OOP is just one paradigm or way of thinking about programming; it's not omnipotent. In many scenarios, OOP needs to be combined with other programming paradigms to leverage their respective advantages, such as:
- Functional Programming: Python supports functional features like higher-order functions, lambdas, decorators, which can be flexibly used in OOP.
- Procedural Programming: For some simple tasks, procedural programming might be more concise and intuitive.
In short, OOP is not a dogma. Rather than rigidly adhering to rules, it's better to integrate other ideas to improve code readability and practicality. Mastering multiple programming paradigms is the programming literacy that a qualified Pythonista should have.
Summary
Well, that's it for this article. We've covered everything from the core concepts of OOP to code practices, interspersed with plenty of example code and personal insights. I hope that through this blog post, you not only grasp the various aspects of OOP in Python but also understand the design philosophy and essence of OOP.
Of course, OOP is just one programming paradigm; it's not the entirety of programming. Combining it with other programming ideas is the way to unleash Python's full potential. Maintaining an open and inclusive mindset, continuously learning and thinking, is our driving force to move forward. Let's progress together on the path of Python!
Who has not died since ancient times? Let's not waste today to achieve great deeds. With tiger-like vigilance and wolf-like caution, We must exert all our strength to the fullest. - Anonymous
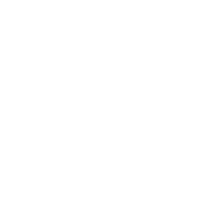
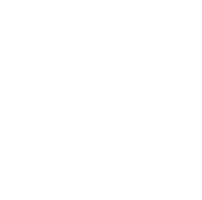