Hello, Python enthusiasts! Today we're diving into a hot topic—containerizing Python applications. Are you often troubled by environment configuration issues? Or do you encounter strange errors when deploying on different machines? Don't worry, containerization can help solve these problems. Let's explore how to package Python applications into containers for true "build once, run anywhere."
Introduction to Containerization
First, let's talk about what containerization is. Simply put, it's packaging an application and all its dependencies into an isolated environment. This environment is like a small, independent operating system containing everything needed to run the application.
Think of a container as a carefully packed suitcase. Wherever you go, just open it, and everything inside is complete and ready to use. Python applications in containers work the same way, ensuring consistent environments no matter which machine they run on.
What are the core advantages of containerization? I think there are several:
- Environment Isolation: Each container is independent and won't affect others.
- Portability: Runs on any system that supports container technology.
- Version Control: Easily manage different versions of applications.
- Resource Efficiency: Lighter and faster to start than traditional virtual machines.
For Python developers, the benefits of containerization are even more apparent. Remember those debugging nightmares caused by inconsistent environments? With containers, those problems become history.
Docker: The Synonym for Containerization
When it comes to containerization, Docker is a must-mention. Docker has become the de facto standard in container technology. As a Python developer, I was initially intimidated by Docker, but once I started using it, I found it wasn't complicated and greatly improved my efficiency.
Let's step through using Docker to containerize a Python application.
Step 1: Choose a Base Image
First, we need to choose a base image. It's like selecting a base environment for your application. Python's official Docker images come in various versions, so we can choose based on our needs. For a lightweight environment, consider python:3.11-slim
.
FROM python:3.11-slim
This line tells Docker to use the slim version of Python 3.11 as the base image.
Step 2: Create a Non-Privileged User
Security is paramount. Running applications in a container with a non-privileged user is a good practice to reduce potential security risks.
RUN groupadd -g 1000 python && \
useradd -r -u 1000 -g python python
This code creates a user named "python" and a group with the same name.
Step 3: Manage Dependencies
Next, we need to install the application's dependencies. We typically use a requirements.txt
file to manage them.
COPY requirements.txt .
RUN pip install -r requirements.txt
Here, we first copy the requirements.txt
file into the container and then use pip to install all dependencies.
Step 4: Set Up the Work Environment
To maintain good organization, we usually create a dedicated work directory in the container.
RUN mkdir /app && chown python:python /app
WORKDIR /app
This code creates a /app
directory and sets it as the current work directory.
Step 5: Copy Application Code
Now, it's time to copy our Python code into the container.
COPY app.py .
This line copies the app.py
file into the container's current work directory.
Step 6: Configure the Runtime Environment
Finally, we need to configure the container's runtime environment.
USER 1000
CMD ["python", "app.py"]
Here, we switch to the previously created non-privileged user and set the command to execute when the container starts.
Build and Run the Docker Image
After completing the Dockerfile, we can build the Docker image. In the terminal, navigate to the directory containing the Dockerfile and run:
docker build -t my-python-app .
This command builds a Docker image named my-python-app
. The build process may take a few minutes depending on your network speed and machine performance.
Once built, we can run the image:
docker run -p 8080:80 my-python-app
This command starts a container and maps the container's port 80 to host port 8080. If your application is a web service, you should now be able to access it at http://localhost:8080
.
Isn't it amazing? The first time I successfully ran a containerized Python application, I was deeply impressed by this magic. Imagine, whether on your development machine or production server, your application can run identically as long as Docker is present, greatly reducing the "it works on my machine" headaches.
Docker Compose: Managing Complex Applications
At this point, you might wonder, what if my application is complex and includes multiple services? Don't worry—Docker Compose is designed to solve this problem.
Docker Compose allows you to define and run multiple Docker containers using a single YAML file. This is particularly useful for managing Python applications with multiple components. For example, you might have a Flask application, a Redis cache, and a PostgreSQL database.
Let's look at a simple example. Suppose we have a Flask application and a Redis cache. First, create a Dockerfile
for the Flask app:
FROM python:3.8
WORKDIR /code
COPY requirements.txt .
RUN pip install -r requirements.txt
COPY ./src .
CMD ["python", "./server.py"]
Then, create a docker-compose.yml
file:
version: '3'
services:
web:
build: .
ports:
- "5000:5000"
redis:
image: "redis:alpine"
This file defines two services: our Flask application (web) and Redis. Using Docker Compose, we can start the entire application with a single command:
docker-compose up
This way, our Flask application and Redis cache start simultaneously, and they can communicate using service names. Doesn't managing complex applications feel much simpler now?
Best Practices for Containerization
Throughout my containerization journey, I've gathered some best practices that I hope will help you:
-
Choose the Right Base Image: Use official, slim images whenever possible. This reduces image size and improves security.
-
Optimize the Dockerfile: Place less frequently changing instructions (like installing system dependencies) at the top and more frequently changing ones (like copying application code) at the bottom. This fully utilizes Docker's caching mechanism to speed up builds.
-
Use Multi-Stage Builds: In production, use multi-stage builds to reduce the final image size. For example, compile code in one stage and copy only the compiled results in another.
-
Do Not Store Data in Containers: Containers should be stateless. Store data in volumes or use external database services.
-
Use Environment Variables for Configuration: Avoid hardcoding configurations in images; use environment variables. This allows the same image to use different configurations in different environments.
-
Regularly Update Base Images: Base images are frequently updated to fix security vulnerabilities. Regular updates ensure your application runs in the safest environment.
-
Use a .dockerignore File: Similar to .gitignore, a .dockerignore file prevents unnecessary files from being copied into the Docker image, reducing its size.
Conclusion
Containerization has brought revolutionary changes to Python development. It not only simplifies the deployment process but also enhances the portability and scalability of applications. Mastering containerization as a Python developer can make your work more efficient and open new career opportunities.
Have you used containerization? What challenges have you faced? Feel free to share your thoughts and experiences in the comments. Let's discuss how to better leverage containerization to improve our Python development process.
Remember, technology is constantly evolving. Staying passionate about learning is key to remaining competitive in this fast-changing field. Next time, we can delve into how to use Kubernetes to manage and scale containerized Python applications. Are you looking forward to it?
Happy coding, Pythonistas!
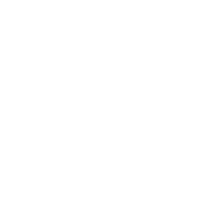