Introduction
Haven't you often encountered this frustration? A Python program that runs perfectly fine on your own computer throws all kinds of errors and incompatibilities on someone else's machine. Or the development environment you painstakingly set up gets messed up by a newly installed package. These issues have given you endless headaches, right?
Don't worry, today we'll talk about Python containerization technology and see how it solves these maddening problems. Containerization not only makes your Python applications more stable and reliable but also greatly improves your development efficiency. Want to know how it's done? Then keep reading!
What is a Container?
Before delving into Python containerization, let's first understand what a container is. Simply put, a container is a lightweight, portable, self-contained software package that includes everything needed to run an application: code, runtime environment, system tools, system libraries, and so on. It's like a standardized box where you can put your application and all its dependencies, and then run it on any system that supports this type of box.
Does that sound a bit abstract? Let's use a more vivid analogy to explain. Imagine you're preparing a lunchbox. You'd put the main dish, side dishes, fruits, and so on into a lunchbox, so no matter where you go, as long as you bring this lunchbox, you can enjoy a complete meal. A container is like this "software lunchbox," containing your application and all its "side dishes" it needs.
Why Containerize?
You might be wondering, why should I containerize my Python application? That's a good question! Let's look at the benefits containerization can bring us:
-
Environment Consistency: Remember that old saying? "It works on my machine." With containers, this problem is completely solved. Whether it's on your laptop, a coworker's desktop, or a cloud server, as long as there's a container runtime environment, your application will behave consistently.
-
Rapid Deployment: Using containers, you can start a new application instance in just a few seconds. This is a godsend for web applications that need to scale quickly. Imagine your website suddenly experiences a traffic spike; with just a few commands, you can rapidly spin up multiple application instances to handle it.
-
Resource Isolation: Each container is a relatively independent environment, meaning you can run multiple different versions of Python or conflicting libraries on the same machine without interfering with each other. This is especially helpful for managing complex dependency relationships.
-
Version Control: Container images can be versioned, allowing you to precisely control each version of your application, including its runtime environment. Need to roll back to a previous version? Just start the container for that version.
-
Simplified Operations: With containers, development, testing, and production environments can be highly consistent. This greatly reduces issues caused by environment differences and simplifies operations work.
How to Containerize
After hearing about all these benefits, you must be eager to know how to containerize your Python application, right? Don't worry, let's look at the specific steps.
Step 1: Prepare Your Python Application
First, you need to have a Python application that can run normally. Let's use a simple Flask application as an example:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def hello():
return "Hello, Containerized Python!"
if __name__ == '__main__':
app.run(host='0.0.0.0', port=5000)
Save this code as app.py
.
Step 2: Create requirements.txt
To ensure that all necessary dependencies are installed in the container, we need to create a requirements.txt
file:
Flask==2.0.1
Step 3: Write Dockerfile
The Dockerfile is the blueprint for building the container image. Create a file named Dockerfile
with the following contents:
FROM python:3.9-slim-buster
WORKDIR /app
COPY . /app
RUN pip install --no-cache-dir -r requirements.txt
EXPOSE 5000
ENV NAME World
CMD ["python", "app.py"]
Step 4: Build the Container Image
Now, we can use Docker to build the container image. Run in the terminal:
docker build -t my-python-app .
This command will create an image named my-python-app
based on the Dockerfile.
Step 5: Run the Container
After the image is built, we can run the container:
docker run -p 5000:5000 my-python-app
This command will start the container and map the container's port 5000 to the host's port 5000.
It's that simple! Now you can open your browser and visit http://localhost:5000
to see the "Hello, Containerized Python!" message.
Advanced Techniques
After mastering the basic containerization steps, let's look at some advanced techniques to make your Python containerization journey even smoother.
1. Multi-stage Builds
If your application needs to compile some C extensions or has other complex build processes, you can consider using multi-stage builds. This can help you keep the final image slim. For example:
FROM python:3.9 AS builder
WORKDIR /app
COPY requirements.txt .
RUN pip install --user -r requirements.txt
FROM python:3.9-slim
WORKDIR /app
COPY --from=builder /root/.local /root/.local
COPY . .
ENV PATH=/root/.local/bin:$PATH
CMD ["python", "app.py"]
2. Use a Non-root User
For improved security, it's best to run your application as a non-root user inside the container:
FROM python:3.9-slim
RUN useradd -m myuser
USER myuser
WORKDIR /home/myuser/app
COPY --chown=myuser:myuser . .
RUN pip install --user -r requirements.txt
CMD ["python", "app.py"]
3. Optimize Caching
Docker's build process uses caching to speed up builds. You can take full advantage of caching by arranging the order of instructions in your Dockerfile appropriately:
FROM python:3.9-slim
WORKDIR /app
COPY requirements.txt .
RUN pip install --no-cache-dir -r requirements.txt
COPY . .
CMD ["python", "app.py"]
This way, dependencies will only be reinstalled when requirements.txt
changes.
4. Use .dockerignore
Create a .dockerignore
file to exclude files that don't need to be copied into the container, which can speed up builds and reduce image size:
__pycache__
*.pyc
*.pyo
*.pyd
.git
.env
.vscode
5. Health Checks
Adding health checks to your container can help Docker better manage the container lifecycle:
FROM python:3.9-slim
WORKDIR /app
COPY . .
RUN pip install --no-cache-dir -r requirements.txt
HEALTHCHECK CMD curl --fail http://localhost:5000/health || exit 1
CMD ["python", "app.py"]
Remember to add a /health
endpoint in your application to respond to health check requests.
Common Issues
In the process of containerizing your Python applications, you may encounter some common issues. Let's take a look at these issues and their solutions:
1. Image Size Too Large
Issue: The built Docker image is too large, affecting transmission and deployment efficiency.
Solutions:
- Use a smaller base image, such as python:3.9-alpine
- Combine multiple commands in a single RUN instruction to reduce layers
- Use multi-stage builds
- Clean up unnecessary files and caches
For example:
FROM python:3.9-alpine
WORKDIR /app
COPY requirements.txt .
RUN pip install --no-cache-dir -r requirements.txt && \
rm -rf /root/.cache/pip
COPY . .
CMD ["python", "app.py"]
2. Slow Build Speed
Issue: Building the Docker image takes a long time every time, affecting development efficiency.
Solutions: - Leverage Docker's build cache - Use a .dockerignore file to exclude unnecessary files - Consider using BuildKit for parallel builds - Use volume mounts for local development and only build the image when deploying
3. Runtime Permission Issues
Issue: The application inside the container cannot write to certain directories or files.
Solution: - Set file permissions correctly in the Dockerfile - Use volumes for data persistence - Consider running the application as a non-root user
FROM python:3.9-slim
RUN useradd -m appuser
USER appuser
WORKDIR /home/appuser/app
COPY --chown=appuser:appuser . .
RUN pip install --user -r requirements.txt
CMD ["python", "app.py"]
4. Complex Dependency Management
Issue: Project dependencies are complex, making it difficult to install and manage them correctly in the container.
Solutions: - Use virtual environment tools like venv or pipenv - Consider using Poetry for dependency management - Use multi-stage builds in the Dockerfile to handle complex dependencies
For example, using Poetry:
FROM python:3.9 as builder
RUN pip install poetry
WORKDIR /app
COPY pyproject.toml poetry.lock ./
RUN poetry export -f requirements.txt --output requirements.txt --without-hashes
FROM python:3.9-slim
WORKDIR /app
COPY --from=builder /app/requirements.txt .
RUN pip install --no-cache-dir -r requirements.txt
COPY . .
CMD ["python", "app.py"]
5. Environment Variable Management
Issue: How to securely manage sensitive environment variables inside the container.
Solutions: - Use Docker's secrets feature - Use environment variable files (.env) - Consider using a dedicated key management service
For example, using an .env file:
FROM python:3.9-slim
WORKDIR /app
COPY . .
RUN pip install --no-cache-dir -r requirements.txt
CMD ["python", "app.py"]
Then, when running the container:
docker run --env-file .env my-python-app
Best Practices
When containerizing your Python applications, following some best practices can help you build more efficient, secure, and maintainable containers. Let's look at these important practices:
1. Keep Base Images Updated
Regularly updating your base images is crucial. This not only gets you the latest security patches but also performance improvements and new features. You can automate this process in your CI/CD pipeline:
steps:
- name: Update base image
run: docker pull python:3.9-slim
- name: Build application image
run: docker build -t my-python-app .
2. Use Pinned Dependency Versions
Use exact version numbers in requirements.txt
to ensure build consistency and reproducibility:
Flask==2.0.1
SQLAlchemy==1.4.23
You can take it a step further and use pip freeze > requirements.txt
to generate a complete list of dependencies, including indirect ones.
3. Implement Graceful Shutdown
Ensure your Python application can properly handle the SIGTERM signal and shut down gracefully. This is crucial for maintaining data consistency and avoiding resource leaks:
import signal
import sys
def sigterm_handler(_signo, _stack_frame):
# Perform cleanup operations
print("Received SIGTERM. Cleaning up...")
sys.exit(0)
signal.signal(signal.SIGTERM, sigterm_handler)
4. Log Management
Output logs to stdout and stderr, so you can easily use Docker's logging management features:
import logging
logging.basicConfig(
level=logging.INFO,
format='%(asctime)s [%(levelname)s] %(message)s',
handlers=[logging.StreamHandler()]
)
logger = logging.getLogger(__name__)
logger.info("Application started")
5. Use Multi-stage Builds to Optimize Image Size
Multi-stage builds can help you create smaller final images:
FROM python:3.9 AS builder
WORKDIR /app
COPY requirements.txt .
RUN pip install --user -r requirements.txt
FROM python:3.9-slim
WORKDIR /app
COPY --from=builder /root/.local /root/.local
COPY . .
ENV PATH=/root/.local/bin:$PATH
CMD ["python", "app.py"]
6. Implement Health Checks
Adding health checks can help Docker better manage your containers:
HEALTHCHECK --interval=30s --timeout=30s --start-period=5s --retries=3 \
CMD curl -f http://localhost:5000/health || exit 1
Implement the corresponding health check endpoint in your application:
@app.route('/health')
def health_check():
# Perform necessary health checks
return jsonify({"status": "healthy"}), 200
7. Use a .dockerignore File
Create a .dockerignore
file to exclude unnecessary files, which can speed up builds and reduce image size:
__pycache__
*.pyc
*.pyo
*.pyd
.git
.env
.vscode
8. Security Considerations
- Run the application as a non-root user
- Don't include sensitive information in the image
- Scan the image for security vulnerabilities
FROM python:3.9-slim
RUN useradd -m appuser
USER appuser
WORKDIR /home/appuser/app
COPY --chown=appuser:appuser . .
RUN pip install --user -r requirements.txt
CMD ["python", "app.py"]
9. Use Caching Effectively
By arranging the order of instructions in your Dockerfile appropriately, you can better leverage Docker's build cache:
FROM python:3.9-slim
WORKDIR /app
COPY requirements.txt .
RUN pip install --no-cache-dir -r requirements.txt
COPY . .
CMD ["python", "app.py"]
10. Container Orchestration Considerations
If you plan to use container orchestration systems (like Kubernetes) in production, you need to consider some additional factors:
- Implement appropriate probes (like liveness and readiness probes)
- Set resource limits and requests appropriately
- Consider using an init process to handle zombie processes
spec:
containers:
- name: my-python-app
image: my-python-app:latest
resources:
requests:
cpu: 100m
memory: 128Mi
limits:
cpu: 500m
memory: 512Mi
livenessProbe:
httpGet:
path: /health
port: 5000
readinessProbe:
httpGet:
path: /ready
port: 5000
Hands-on Case Study
Now that we've learned how to containerize Python applications and some best practices, let's combine this knowledge through a practical case study and see how to build a more complex, production-ready Python application container.
We'll create a simple Flask API service that connects to a PostgreSQL database and provides basic CRUD operations. This example will showcase how to handle database connections, environment variables, logging, and other real-world issues.
Step 1: Prepare the Application Code
First, let's create our Flask application. Create a file named app.py
:
import os
import logging
from flask import Flask, jsonify, request
from flask_sqlalchemy import SQLAlchemy
from sqlalchemy.sql import text
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
app = Flask(__name__)
db_url = os.environ.get('DATABASE_URL', 'postgresql://user:password@db/mydatabase')
app.config['SQLALCHEMY_DATABASE_URI'] = db_url
app.config['SQLALCHEMY_TRACK_MODIFICATIONS'] = False
db = SQLAlchemy(app)
class Item(db.Model):
id = db.Column(db.Integer, primary_key=True)
name = db.Column(db.String(80), unique=True, nullable=False)
def __repr__(self):
return f'<Item {self.name}>'
@app.route('/health')
def health():
try:
db.session.execute(text('SELECT 1'))
return jsonify({"status": "healthy"}), 200
except Exception as e:
logger.error(f"Health check failed: {str(e)}")
return jsonify({"status": "unhealthy"}), 500
@app.route('/items', methods=['GET'])
def get_items():
items = Item.query.all()
return jsonify([{"id": item.id, "name": item.name} for item in items])
@app.route('/items', methods=['POST'])
def create_item():
data = request.json
new_item = Item(name=data['name'])
db.session.add(new_item)
db.session.commit()
return jsonify({"id": new_item.id, "name": new_item.name}), 201
if __name__ == '__main__':
app.run(host='0.0.0.0', port=5000)
Step 2: Create requirements.txt
Create a requirements.txt
file listing all dependencies:
Flask==2.0.1
Flask-SQLAlchemy==2.5.1
psycopg2-binary==2.9.1
gunicorn==20.1.0
Step 3: Write Dockerfile
Create a Dockerfile
:
FROM python:3.9-slim-buster
WORKDIR /app
RUN apt-get update && apt-get install -y --no-install-recommends \
gcc \
&& rm -rf /var/lib/apt/lists/*
COPY requirements.txt .
RUN pip install --no-cache-dir -r requirements.txt
COPY . .
RUN useradd -m myuser
USER myuser
EXPOSE 5000
CMD ["gunicorn", "--bind", "0.0.0.0:5000", "app:app"]
Step 4: Create docker-compose.yml
For local development and testing convenience, we can create a docker-compose.yml
file:
version: '3.8'
services:
web:
build: .
ports:
- "5000:5000"
environment:
- DATABASE_URL=postgresql://user:password@db/mydatabase
depends_on:
- db
healthcheck:
test: ["CMD", "curl", "-f", "http://localhost:5000/health"]
interval: 30s
timeout: 10s
retries: 3
db:
image: postgres:13
environment:
- POSTGRES_USER=user
- POSTGRES_PASSWORD=password
- POSTGRES_DB=mydatabase
volumes:
- postgres_data:/var/lib/postgresql/data
volumes:
postgres_data:
Step 5: Build and Run
Now, we can use Docker Compose to build and run our application:
docker-compose up --build
This will build our Python application image, start the PostgreSQL database, and run our application.
Step 6: Test the Application
Use curl or your preferred API testing tool to test our application:
curl -X POST -H "Content-Type: application/json" -d '{"name":"Test Item"}' http://localhost:5000/items
curl http://localhost:5000/items
curl http://localhost:5000/health
This hands-on case study demonstrates how to containerize a Python web application with a database. It includes many of the best practices we discussed earlier, such as using a non-root user, health checks, environment variable configuration, and more.
In an actual production environment, you may need to consider additional factors, such as:
- Using Docker secrets or an external key management service to handle sensitive information.
- Implementing more advanced logging and monitoring strategies.
- Using Docker networks to isolate services.
- Implementing a database migration strategy.
- Setting appropriate resource limits.
Remember, containerization is a continuous improvement process. As your application becomes more complex, you may need to constantly adjust and optimize your containerization strategy.
Conclusion
Well, our journey into Python containerization has come to an end. We started from the basics and gradually delved into practical application scenarios. You should now have a comprehensive understanding of how to containerize Python applications and have mastered some important best practices.
Containerization technology is profoundly changing the way we develop and deploy software. It not only simplifies the development process but also greatly enhances application portability and scalability. For Python developers, mastering containerization is undoubtedly an important skill.
However, remember that technology is constantly evolving. What we've learned today may need to be updated tomorrow. So, maintain your passion for learning and keep up with the latest technology trends – this is crucial for every developer.
Finally, I want to say, don't be afraid to try. While theoretical knowledge is important, practice is the best way to improve your skills. So, get your hands dirty! Containerize your Python projects, solve problems as you encounter them, and you'll find that you've learned far more than you imagined in the process.
Do you have any other questions about Python containerization? Or have you encountered any interesting issues in your practice? Feel free to share your experiences and thoughts in the comments section. Let's continue to move forward together in this challenging and opportunity-filled technological world!
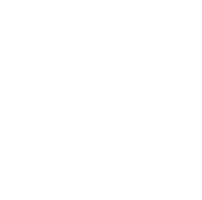