Have you ever encountered a situation where your Python program runs smoothly on your own computer but doesn't work on others? Or you want to deploy your application to a server and find the environment configuration to be a nightmare? If so, Python containerization is definitely a technology you need to understand. Today, let's talk about the cool and practical topic of Python containerization.
What is Containerization
Containerization sounds fancy, but the concept is not complicated. Simply put, it's about packaging your application and everything it needs (like libraries, dependencies, even the operating system) into an independent unit. This unit is called a "container."
Imagine if you could pack your entire development environment into a box, and no matter where you move this box, the environment inside remains exactly the same. Isn't that cool? That's the charm of containerization.
Why Containerize
You might ask, why go to all the trouble of containerizing? What are the benefits? Let me list a few:
-
Environment Consistency: Remember the old saying, "It works on my machine"? With containers, this problem is completely solved. Because the container includes everything needed to run the program, the environment is consistent no matter where it's run.
-
Fast Deployment: Imagine no longer needing to spend a lot of time configuring server environments. With just a few commands, you can deploy your application anywhere. That's the convenience containerization brings.
-
Resource Saving: Compared to traditional virtual machines, containers are more lightweight. They start faster and use fewer resources. This means you can run more applications on the same hardware.
-
Easy Scaling: When your application needs to handle more requests, you can easily start more container instances. This flexibility makes scaling simple.
Docker: The Star of Containerization
Speaking of containerization, we must mention Docker. Docker is like the superhero of the container world, making containerization simple and easy to use.
Docker's core concepts include:
- Docker Image: Like a template, it contains everything needed to run an application.
- Docker Container: A running instance created from an image.
- Dockerfile: A text file with instructions on how to build a Docker image.
Hands-On: Containerizing a Python Project
Enough talk, let's get hands-on and see how to containerize a Python project.
Step 1: Choose a Base Image
First, we need to choose a base image. For Python projects, we usually choose the official Python image. For example, we can use the python:3.9-slim
image.
Step 2: Create a Dockerfile
Next, we need to create a Dockerfile. This file tells Docker how to build our image.
FROM python:3.9-slim
WORKDIR /app
COPY . /app
RUN pip install --no-cache-dir -r requirements.txt
EXPOSE 80
ENV NAME World
CMD ["python", "app.py"]
This Dockerfile does the following: 1. Uses Python 3.9 as the base image 2. Sets the working directory 3. Copies project files into the container 4. Installs dependencies 5. Exposes a port 6. Sets environment variables 7. Specifies the startup command
Step 3: Create requirements.txt
To ensure our application runs smoothly in the container, we need to list all dependencies. Create a requirements.txt
file:
Flask==2.0.1
numpy==1.21.0
pandas==1.3.0
Step 4: Build the Docker Image
Now, we can build the Docker image. Run in the terminal:
docker build -t my-python-app .
This command builds an image named my-python-app
based on the Dockerfile.
Step 5: Run the Docker Container
With the image built, we can run the container:
docker run -p 4000:80 my-python-app
This command starts the container and maps port 80 of the container to port 4000 of the host.
The Magic of Containerization
Look, it's that simple to package a Python application into a container. Now, wherever you run this container, it behaves exactly the same. That's the magic of containerization!
You might ask, how does this help my daily development? Imagine when developing a complex Python project, you might need to use multiple versions of Python or rely on some special system libraries. If developing directly on your machine, these different environments might interfere with each other. But with containers, you can create an isolated environment for each project, preventing interference.
Moreover, when collaborating with team members, containerization can greatly simplify the process. You no longer need to worry about "it works on my computer" because everyone can use exactly the same development environment.
Advanced Tips: Optimizing Your Python Container
Once you're familiar with the basic containerization process, you might want to further optimize your Python container. Here are a few tips to help:
-
Use Multi-Stage Builds: If your project needs to compile some components, you can use multi-stage builds to reduce the final image size.
-
Choose the Right Base Image: Python officially offers multiple images, like
python:3.9-slim
andpython:3.9-alpine
. The Alpine version is smaller but might lack some system libraries. -
Use .dockerignore File: Similar to .gitignore, you can use a .dockerignore file to exclude files that don't need to be copied into the container, speeding up the build process and reducing image size.
-
Arrange Dockerfile Instructions Orderly: Place infrequently changing instructions (like installing system dependencies) at the front and frequently changing instructions (like copying source code) at the back to fully utilize Docker's caching mechanism and speed up builds.
The Future of Containerization: Kubernetes
When talking about containerization, we must mention Kubernetes. If Docker makes managing a single container simple, Kubernetes makes orchestrating large-scale containers possible.
Imagine when your Python application suddenly needs to handle a surge of requests, you need to quickly scale your application instances. Using Kubernetes, you can easily achieve auto-scaling, automatically increasing or decreasing the number of running containers based on load.
Moreover, Kubernetes provides powerful features like service discovery, load balancing, and auto-recovery. These features allow you to build more robust and reliable Python applications.
Conclusion
Containerization technology is changing the way we develop and deploy Python applications. It not only solves the problem of environment consistency but also provides powerful tools for application scaling and management. As a Python developer, mastering containerization technology will undoubtedly give you an advantage in this fast-changing tech world.
What are your thoughts on Python containerization? What challenges have you faced when using containerization technology? Feel free to share your experiences and thoughts in the comments. Let's explore and grow together!
Remember, learning technology is a continuous process. Stay curious, keep trying new things, and you'll find the world of Python more exciting than you imagined. Happy coding!
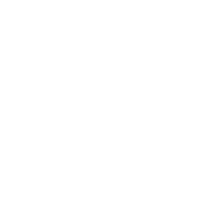
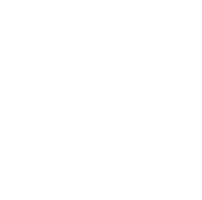